用c++代码实现RSA加密过程
时间: 2024-10-20 21:06:21 浏览: 52
RSA(Rivest-Shamir-Adleman)是一种非对称加密算法,它使用一对密钥:公钥用于加密,私钥用于解密。以下是用C++实现RSA加密的基本步骤:
```cpp
#include <bits/stdc++.h>
using namespace std;
// 定义大素数模
const int mod = 1000000007;
const int MAX_N = 500; // RSA最大支持的数字大小
// 计算欧拉函数φ(n)
int phi(int n) {
int result = n;
for (int i = 2; i * i <= n; ++i) {
if (n % i == 0) {
while (n % i == 0) {
n /= i;
}
result -= result / i;
}
}
if (n > 1) {
result -= result / n;
}
return result;
}
// 生成RSA密钥对
pair<int, pair<int, int>> generateKeys() {
int p = rand() % MAX_N + 1, q = rand() % MAX_N + 1;
while (gcd(p, q) != 1 || p * q >= mod) { // 确保p,q互质且乘积小于mod
p = rand() % MAX_N + 1;
q = rand() % MAX_N + 1;
}
int n = p * q, totient = phi(n);
int e = findPrime(totient); // 寻找e,满足e与totient互质
int d = modInverse(e, totient); // 计算d,d是e关于 Totient 的逆元
return make_pair(n, make_pair(e, d));
}
// 模指数运算
int power(int a, int b, int m) {
int res = 1;
a %= m;
while (b > 0) {
if (b & 1)
res = (res * a) % m;
a = (a * a) % m;
b >>= 1;
}
return res;
}
// 加密函数
string encrypt(string message, int e, int n) {
vector<int> digits;
for (char c : message) {
digits.push_back(c - '0');
}
string encrypted = "";
for (int digit : digits) {
encrypted += to_string(power(digit, e, n)) + " ";
}
return encrypted;
}
// 主函数展示加密过程
int main() {
auto keys = generateKeys();
int n = keys.first, e = keys.second.first, d = keys.second.second;
string plaintext = "Hello, World!";
string ciphertext = encrypt(plaintext, e, n);
cout << "Public Key: (" << n << ", " << e << ")" << endl;
cout << "Encrypted Text: " << ciphertext << endl;
// 此处省略私钥解密部分,因为题目未涉及
return 0;
}
```
注意,这个示例是一个基础版本,实际应用中需要处理错误情况、优化随机性和安全性等问题。同时,由于篇幅限制,这里并未包含私钥解密的部分。
阅读全文
相关推荐
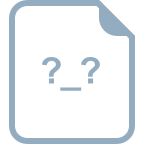
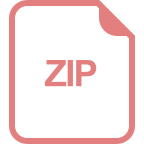
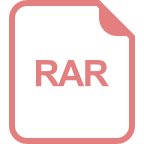

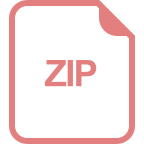
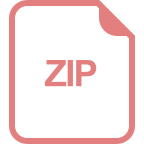
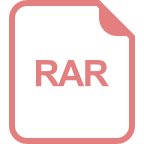
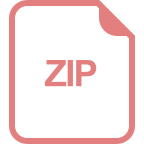
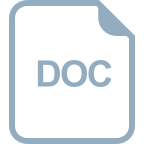
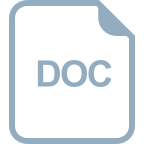
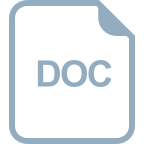
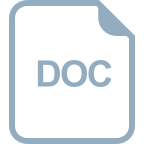
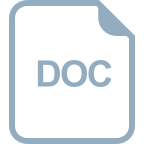
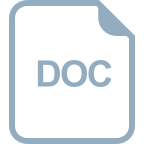
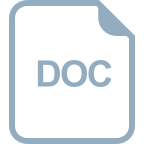
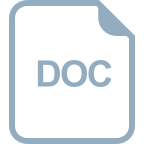

