canny python without cv2
时间: 2023-09-08 08:08:25 浏览: 90
As an AI language model, I cannot perform programming tasks but I can provide you with information.
The Canny edge detection algorithm can be implemented in Python without using the cv2 library by using the following steps:
1. Read the input image using the PIL library:
```python
from PIL import Image
img = Image.open('input_image.png')
```
2. Convert the image to grayscale:
```python
img_gray = img.convert('L')
```
3. Apply the Gaussian blur filter to the grayscale image:
```python
from scipy.ndimage import gaussian_filter
img_blur = gaussian_filter(img_gray, sigma=1)
```
4. Compute the gradient magnitude and direction for each pixel:
```python
from scipy.ndimage import sobel
dx = sobel(img_blur, axis=1)
dy = sobel(img_blur, axis=0)
magnitude = np.sqrt(dx**2 + dy**2)
direction = np.arctan2(dy, dx)
```
5. Apply non-maximum suppression to thin the edges:
```python
def non_max_suppression(magnitude, direction):
rows, cols = magnitude.shape
output = np.zeros((rows, cols), dtype=np.int32)
angle = direction * 180. / np.pi
angle[angle < 0] += 180
for i in range(1, rows - 1):
for j in range(1, cols - 1):
q = 255
r = 255
# angle 0
if (0 <= angle[i, j] < 22.5) or (157.5 <= angle[i, j] <= 180):
q = magnitude[i, j+1]
r = magnitude[i, j-1]
# angle 45
elif (22.5 <= angle[i, j] < 67.5):
q = magnitude[i+1, j-1]
r = magnitude[i-1, j+1]
# angle 90
elif (67.5 <= angle[i, j] < 112.5):
q = magnitude[i+1, j]
r = magnitude[i-1, j]
# angle 135
elif (112.5 <= angle[i, j] < 157.5):
q = magnitude[i-1, j-1]
r = magnitude[i+1, j+1]
if (magnitude[i, j] >= q) and (magnitude[i, j] >= r):
output[i, j] = magnitude[i, j]
else:
output[i, j] = 0
return output
img_thin = non_max_suppression(magnitude, direction)
```
6. Apply hysteresis thresholding to obtain the final edges:
```python
def hysteresis_thresholding(img, low_threshold, high_threshold):
rows, cols = img.shape
output = np.zeros((rows, cols), dtype=np.int32)
strong_row, strong_col = np.where(img > high_threshold)
weak_row, weak_col = np.where((img >= low_threshold) & (img <= high_threshold))
output[strong_row, strong_col] = 255
output[weak_row, weak_col] = 50
for i in range(1, rows - 1):
for j in range(1, cols - 1):
if (output[i, j] == 50):
if ((output[i+1, j-1] == 255) or (output[i+1, j] == 255) or (output[i+1, j+1] == 255)
or (output[i, j-1] == 255) or (output[i, j+1] == 255)
or (output[i-1, j-1] == 255) or (output[i-1, j] == 255) or (output[i-1, j+1] == 255)):
output[i, j] = 255
else:
output[i, j] = 0
return output
img_edges = hysteresis_thresholding(img_thin, low_threshold=20, high_threshold=50)
```
7. Save the output image using the PIL library:
```python
output_image = Image.fromarray(np.uint8(img_edges))
output_image.save('output_image.png')
```
阅读全文
相关推荐
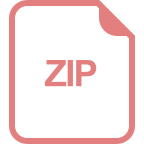
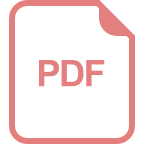
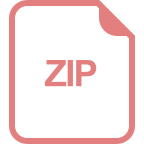
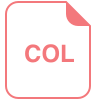

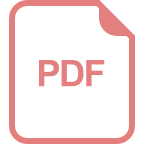
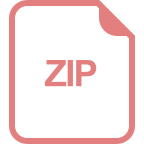
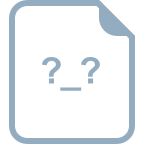
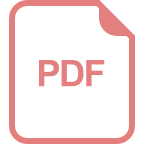
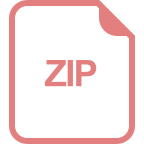
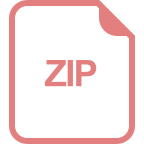
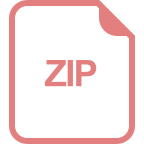
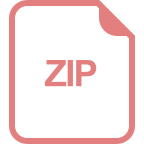
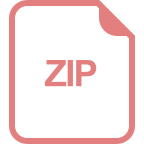
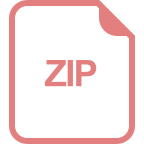
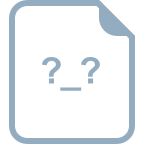