[Advanced] How to Call Python from MATLAB
发布时间: 2024-09-13 16:54:45 阅读量: 31 订阅数: 43 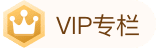
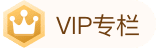
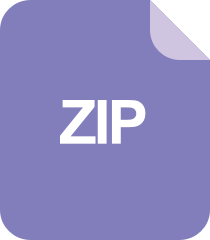
基于遗传算法的动态优化物流配送中心选址问题研究(Matlab源码+详细注释),遗传算法与免疫算法在物流配送中心选址问题的应用详解(源码+详细注释,Matlab编写,含动态优化与迭代,结果图展示),遗传
# **Advanced篇:How to Call Python from MATLAB**
# 1. **Python Interpreter Launch and Initialization**
Interoperability between MATLAB and Python requires the launching and initialization of the Python interpreter. In MATLAB, the `pyenv` function can be used to manage the Python interpreter. The `pyenv` function provides the following main functionalities:
- **Creating a Python interpreter:** `pyenv('Python')` creates a new Python interpreter.
- **Setting the Python version:** `pyenv('Version', '3.9')` specifies the Python version to be used.
- **Retrieving Python interpreter information:** `pyenv('Version')` returns the currently used Python version.
- **Destroying the Python interpreter:** `pyenv('delete')` destroys the current Python interpreter.
For instance, the following code creates a Python 3.9 interpreter:
```matlab
pyenv('Python', '3.9');
```
After creating the interpreter, the `py.init` function can be used to initialize the Python environment. The `py.init` function loads the necessary modules and sets environment variables, enabling MATLAB to interact with Python.
```matlab
py.init;
```
# 2. Practical Tips for Calling Python from MATLAB
### 2.1 Python Interpreter Launch and Initialization
Before calling Python from MATLAB, the Python interpreter must be launched. The `py.init()` function can be used to start the interpreter, which creates a Python session and returns a `py.interface` object. The `py.interface` object can be used to interact with the Python interpreter.
```matlab
% Launch Python interpreter
py.init();
```
### 2.2 Data Type Conversion Between MATLAB and Python
MATLAB and Python use different data type systems. When calling Python functions or passing parameters, data types need to be converted. In MATLAB, the `py.cast()` function can be used to convert MATLAB data to Python data types, and vice versa.
```matlab
% Convert MATLAB matrix to Python list
python_list = py.list(matlab_matrix);
% Convert Python list to MATLAB matrix
matlab_matrix = double(py.array(python_list));
```
### 2.3 Calling Functions and Scripts and Passing Parameters
MATLAB can call Python functions and scripts using the `py.run()` and `py.runfile()` functions. The `py.run()` function directly executes Python code, while the `py.runfile()` function executes the specified file.
When passing parameters, the `py.args()` function can be used to create a Python tuple containing the parameters to be passed.
```matlab
% Call Python function and pass parameters
result = py.run('my_function.py', py.args('arg1', 'arg2'));
% Execute Python script
py.runfile('my_script.py');
```
### 2.4 Error Handling and Debugging
Errors may occur when calling Python from MATLAB. The `try-catch` block can be used to catch errors and handle them.
```matlab
try
% Call Python function
result = py.run('my_function.py', py.args('arg1', 'arg2'));
catch ME
% Handle error
disp(ME.message);
end
```
# 3. Advanced Applications of Calling Python from MATLAB
### 3.1 Importing Python Modules and Packages
Calling Python modules and packages from MATLAB can extend MATLAB's functionality, leveraging Python's rich ecosystem.
**Module Import**
```matlab
importlib.import_module('numpy');
```
**Package Import**
```matlab
importlib.import_module('scipy.stats');
```
**Parameter Explanation:**
* `importlib.import_module`: MATLAB function used to import Python modules or packages.
* `'numpy'`: Name of the Python module to be imported.
* `'scipy.stats'`: Name of the Python package to be imported.
### 3.2 Calling Python Classes and Objects
MATLAB can call Python classes and objects through the Python gateway, enabling cross-language object interaction.
**Class Instantiation**
```matlab
% Create an instance of a Python class
my_class = py.my_module.MyClass();
```
**Method Call**
```matlab
% Call a Python class method
result = my_class.my_method(10, 20);
```
**Property Access**
```matlab
% Access a Python class property
my_property = my_class.my_property;
```
**Parameter Explanation:**
* `py.my_module.MyClass()`: Create an instance of a Python class, where `my_module` is the name of the Python module and `MyClass` is the class name.
* `my_class.my_method(10, 20)`: Call a Python class method, where `my_method` is the method name and `10` and `20` are parameters.
* `my_class.my_property`: Access a Python class property, where `my_property` is the property name.
### 3.3 Parallel Computing and Distributed Processing
Interoperability between MATLAB and Python supports parallel computing and distributed processing, enhancing computational efficiency.
**Parallel Computing**
```matlab
% Create a parallel pool in Python
pool = py.multiprocessing.Pool(4);
% Assign tasks
tasks = cell(1, 100);
for i = 1:100
tasks{i} = @(x) x^2;
end
% Execute tasks in parallel
results = pool.map(tasks, 1:100);
```
**Distributed Processing**
```matlab
% Create a distributed client in Python
client = py.dask.distributed.Client();
% Submit a task
future = client.submit(lambda x: x^2, 100)
% Retrieve the result
result = future.result()
```
**Parameter Explanation:**
* `py.multiprocessing.Pool(4)`: Create a Python parallel pool, where `4` specifies the number of parallel processes.
* `tasks`: List of tasks to be executed in parallel.
* `pool.map(tasks, 1:100)`: Map tasks to the parallel pool and execute them in parallel.
* `py.dask.distributed.Client()`: Create a Python distributed client.
* `future = client.submit(lambda x: x^2, 100)`: Submit a task to the distributed client.
* `future.result()`: Retrieve the result of the distributed task.
# 4. Best Practices for MATLAB and Python Inte
0
0