[Advanced Chapter] Key Points Detection for Facial Images in MATLAB: Using Dlib for Facial Image Key Points Detection
发布时间: 2024-09-15 03:29:40 阅读量: 18 订阅数: 39 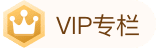
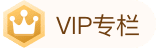
# 1. Introduction to Facial Landmark Detection in Images
Facial landmark detection in images is a computer vision technique that identifies and locates key feature points on a human face, such as eyes, nose, mouth, etc., to understand and analyze facial images. These landmarks provide rich feature information for applications such as facial expression recognition, identity verification, and emotional analysis.
# 2. Application of Dlib Library in Facial Landmark Detection
### 2.1 Installation and Configuration of Dlib Library
**Installing Dlib Library**
The Dlib library is a cross-platform C++ library that can be used on operating systems such as Windows, Linux, and macOS. The installation steps are as follows:
1. Download the latest version of the Dlib library: ***
***
***
```
mkdir build
cd build
cmake ..
make
```
**Configuring Dlib Library**
After installation, you need to configure the Dlib library path so that other programs can access it.
**Windows System:**
1. Open the control panel
2. Select "System and Security"
3. Select "System"
4. Click "Advanced System Settings"
5. In the "Environment Variables" tab, find the "Path" variable
6. Add the installation directory of the Dlib library to the "Path" variable, for example: `C:\path\to\dlib\build`
**Linux System:**
1. Open the terminal
2. Run the following command:
```
export LD_LIBRARY_PATH=$LD_LIBRARY_PATH:/path/to/dlib/build
```
### 2.2 Facial Detection and Landmark Detection Modules in Dlib Library
The Dlib library provides two main modules for facial detection and landmark detection:
**dlib::get_frontal_face_detector():** Used to detect faces in an image.
**dlib::shape_predictor():** Used to predict the landmarks of the detected faces.
**Facial Detection**
The facial detection module uses a sliding window detector based on HOG features. It can detect faces in an image and return a list of rectangles containing the face bounding boxes.
**Landmark Detection**
The landmark detection module uses a cascaded regression model to predict facial landmarks. It can predict 68 landmarks, including eyes, nose, mouth, and chin.
**Using Dlib Library for Facial and Landmark Detection**
The following code demonstrates how to use the Dlib library for facial and landmark detection:
```cpp
#include <dlib/opencv.h>
#include <dlib/image_processing/frontal_face_detector.h>
#include <dlib/image_processing/shape_predictor.h>
int main() {
// Load face detector
dlib::frontal_face_detector face_detector = dlib::get_frontal_face_detector();
// Load landmark detector
dlib::shape_predictor shape_predictor;
dlib::deserialize("shape_predictor_68_face_landmarks.dat") >> shape_predictor;
// Load image
cv::Mat image = cv::imread("image.jpg");
// Convert image format
dlib::cv_image<dlib::bgr_pixel> dlib_image(image);
// Detect faces
std::vector<dlib::rectangle> faces = face_detector(dlib_image);
// Predict landmarks
for (const auto& face : faces) {
dlib::full_object_detection shape = shape_predictor(dlib_image, face);
// Draw landmarks
for (int i = 0; i < shape.num_parts(); i++) {
cv::circle(image, cv::Point(shape.part(i).x(), shape.part(i).y()), 2, cv::Scalar(0, 255, 0), -1);
}
}
// Display image
cv::imshow("Image", image);
cv::waitKey(0);
return 0;
}
```
**Code Logic Analysis:**
1. Load face and landmark detectors.
2. Load image and convert it to Dlib image format.
3. Use face detector to detect faces in the image.
4. For each detected face, use landmark detector to predict landmarks.
5. Draw landmarks on the image.
6. Display image.
# 3. Using Dlib in MATLAB for Facial Landmark Detection
### 3.1 Interface Between MATLAB and Dlib Library
The interface between MATLAB and the Dlib library can be implemented through MATLAB mex files. A mex file is a MATLAB executable that allows MATLAB to call C++ code. The Dlib library provides precompiled mex files, which can be easily integrated into MATLAB.
To use the mex files of the Dlib library, the following steps need to be executed:
***
***pile the Dlib library's mex files. This can be done by running the following commands:
```
mex -setup C++
mex -I<dlib_include_path> -L<dlib_lib_path> -l<dlib_lib_name> <mex_file_path>
```
Where `<dlib_include_path>` is the header file directory of the Dlib library, `<dlib_lib_path>` is the library file directory of the Dlib library, `<dlib_lib_name>` is the library file name of the Dlib library, and `<mex_file_path>` is the path of the mex file.
3. Add the compiled mex file to the MATLAB path. This can be done using the `addpath` function:
```
addpath('<mex_file_path>')
```
### 3.2 MATLAB Code Implementation for Facial Landmark Detection
The MATLAB code implementation for using Dlib library for facial landmark detection is as follows:
```matlab
% Load image
image = imread('image.jpg');
% Create Dlib face detector
detector = dlib.get_frontal
```
0
0
相关推荐
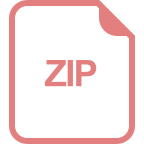
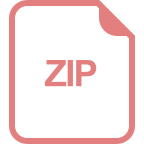






