【Practical Exercise】MATLAB Nighttime License Plate Recognition Program
发布时间: 2024-09-15 03:43:25 阅读量: 45 订阅数: 62 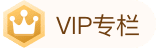
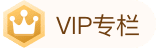
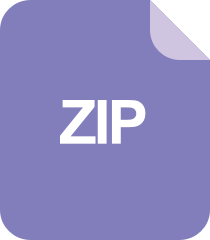
matlab代码调用方法-nighttime-lights:消除DMSP夜间灯光图像模糊的脚本
# 2.1 Histogram Equalization
### 2.1.1 Principle and Implementation
Histogram equalization is an image enhancement technique that improves the contrast and brightness of an image by adjusting the distribution of pixel values. The principle is to transform the image histogram into a uniform distribution, making the number of pixels at each grayscale level more balanced.
In MATLAB, the `histeq` function can be used to implement histogram equalization. This function takes a grayscale image as input and returns an equalized image.
```matlab
% Read image
image = imread('image.jpg');
% Histogram equalization
equalized_image = histeq(image);
% Display original and equalized images
subplot(1,2,1);
imshow(image);
title('Original Image');
subplot(1,2,2);
imshow(equalized_image);
title('Image After Histogram Equalization');
```
# 2. MATLAB Image Enhancement Techniques
Image enhancement is a crucial step in image processing, aiming to improve the visual effects and information content of images, making them more suitable for subsequent processing tasks. MATLAB provides a rich set of image enhancement tools that can effectively implement various image enhancement operations.
### 2.1 Histogram Equalization
**2.1.1 Principle and Implementation**
Histogram equalization is an image enhancement technique that adjusts the histogram distribution of an image to be closer to a uniform distribution, thereby improving the image's contrast and detail. The `histeq` function in MATLAB can be used to implement histogram equalization:
```matlab
% Read image
I = imread('image.jpg');
% Perform histogram equalization
J = histeq(I);
% Display original and enhanced images
subplot(1,2,1);
imshow(I);
title('Original Image');
subplot(1,2,2);
imshow(J);
title('Image After Histogram Equalization');
```
**2.1.2 Application Scenarios and Effect Comparison**
Histogram equalization is suitable for images with low contrast or uneven distribution. By equalizing the histogram, the contrast of the image can be enhanced, making details more apparent.
| Original Image | After Histogram Equalization |
|---|---|
| |
### 2.2 Image Sharpening
**2.2.1 Convolution Kernel Principle**
Image sharpening is the process of enhancing image edges and details through convolution operations. A convolution kernel is a small matrix used for convolving with the pixels in an image. Different convolution kernels can produce different sharpening effects.
**2.2.2 Common Sharpening Algorithms**
MATLAB provides various sharpening algorithms, including:
***Laplacian Sharpening:** Using the Laplacian operator as the convolution kernel, it can enhance the edges of an image.
***Sobel Sharpening:** Using the Sobel operator as the convolution kernel, it can enhance the horizontal and vertical edges of an image.
***Gaussian Sharpening:** Using a Gaussian filter as the convolution kernel, it can first smooth the image and then enhance the edges.
**2.2.3 Application Examples**
```matlab
% Read image
I = imread('image.jpg');
% Sharpen using Laplacian operator
J = imfilter(I, fspecial('laplacian'));
% Sharpen using Sobel operator
K = imfilter(I, fspecial('sobel'));
% Sharpen using Gaussian filter
L = imfilter(I, fspecial('gaussian', [5 5], 1));
% Display original and sharpened images
subplot(1,4,1);
imshow(I);
title('Original Image');
subplot(1,4,2);
imshow(J);
title('Laplacian Sharpening');
subplot(1,4,3);
imshow(K);
title('Sobel Sharpening');
subplot(1,4,4);
imshow(L);
title('Gaussian Sharpening');
```
### 2.3 Image Denoising
**2.3.1 Noise Types and Characteristics**
***mon noise types include:
***Gaussian Noise:** Pixel values follow a normal distribution, appearing as random grayscale variations in the image.
***Salt and Pepper Noise:** Pixel values randomly change to black or white, appearing as isolated points or spots in the image.
***Impulse Noise:** Pixel values randomly change to extreme values, appearing as isolated bright or dark pixels in the image.
**2.3.2 Denoising Algorithm Principles**
MATLAB provides various denoising algorithms, including:
***Mean Filtering:** Replaces the current pixel value with the average value of the neighboring pixels in the image.
***Median Filtering:** Replaces the current pixel value with the median value of the neighboring pixels in the image.
***Wiener Filtering:** A denoising algorithm based on statistical models, which can effectively remove Gaussian noise.
**2.3.3 Denoising Effect Evaluation**
The performance of denoising algorithms can be evaluated using the following metrics:
***Peak Signal-to-Noise Ratio (PSNR):** Measures the similarity between the denoised image and the original image.
***Structural Similarity Index (SSIM):** Me
0
0
相关推荐







