[Basic] 3D Image Plotting in MATLAB: Plotting 3D Surface Graphs
发布时间: 2024-09-15 02:13:32 阅读量: 41 订阅数: 60 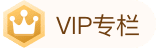
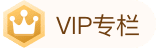
# 1. Overview of MATLAB 3D Graph Plotting
MATLAB, as a powerful tool for scientific computation and data visualization, offers rich functionalities and flexible features for plotting three-dimensional graphics. Three-dimensional plotting enables us to visually present and analyze complex data, finding extensive applications in scientific research, engineering design, medical imaging, and many other fields. This chapter will provide an overview of the basic concepts and advantages of MATLAB 3D graph plotting, laying the groundwork for in-depth discussions in subsequent chapters.
# 2.1 Three-Dimensional Coordinate Systems and Projection Transformations
### Three-Dimensional Coordinate Systems
A three-dimensional coordinate system consists of three mutually perpendicular axes: the x-axis, y-axis, and z-axis. The origin is the point where all three axes intersect.
```matlab
% Creating a three-dimensional coordinate system
figure;
axis equal;
xlabel('x');
ylabel('y');
zlabel('z');
grid on;
```
### Projection ***
***mon types of projection transformations include:
- **Orthographic Projection:** Projects along each axis, parallel to the other two axes.
- **Perspective Projection:** Projects from a viewpoint, with objects appearing smaller the further they are.
In MATLAB, the `view` function is used to set the projection type:
```matlab
% Setting orthographic projection
view(3);
% Setting perspective projection
view(30, 30);
```
### Projection Transformation Matrices
Projection transformation matrices convert three-dimensional coordinates into two-dimensional coordinates. An orthographic projection matrix is:
```matlab
P_ortho = [
***
***
***
***
];
```
A perspective projection matrix is:
```matlab
P_persp = [
***
***
*** -1/d
0 0 0 1
];
```
Here, `d` is the distance from the observer to the projection plane.
### Summary
Three-dimensional coordinate systems and projection transformations are the foundation of 3D surface plot drawing. Understanding these concepts is crucial for accurately representing and visualizing three-dimensional scenes.
# 3.1 Usage and Parameter Details of the surf Function
**Introduction to the surf Function**
The surf function is used to plot three-dimensional surface graphs. It creates a mesh by specifying the x, y, and z coordinate data for the surface and then renders the mesh as a surface. The syntax for the surf function is as follows:
```matlab
surf(X, Y, Z)
surf(X, Y, Z, C)
surf(X, Y, Z, C, 'PropertyName', PropertyValue, ...)
```
**Parameter Details**
***X, Y, Z:** The x, y, and z coordinate data for the surface, which can be in matrix, vector, or scalar form.
***C:** The color data for the surface, which can be in matrix, vector, or scalar form. If omitted, the default colormap is used.
***'PropertyName', PropertyValue:** Optional property-value pairs to control the appearance and behavior of the surface.
**Common Properties**
| Property | Description |
|---|---|
| EdgeColor | The color of the mesh lines |
| FaceColor | The color of the surface |
| FaceLighting | Whether lighting is enabled |
| LineWidth | The width of the mesh lines |
| Marker | The type of marker for vertices |
| MarkerFaceColor | The fill color for vertices |
| MarkerSize | The size of the vertices |
| Shading | The shading mode |
**Code Example**
```matlab
% Creating a sine surface
[X, Y] = meshgrid(-2:0.1:2);
Z = sin(X.^2 + Y.^2);
% Plotting the surface graph
surf(X, Y, Z);
```
**Logical Analysis**
This code uses the `meshgrid` function to create the x and y coordinate data for a sine surface, and then uses the `surf` function to plot the surface graph.
### 3.2 Usage and Parameter Details of the mesh Function
**Introduction to the mesh Function**
The `mesh` function is similar to the `surf` function in plotting three-dimensional surface graphs. However, the `mesh` function draws a grid made of triangles instead of the quadrilaterals drawn by the `surf` function. The syntax for the `mesh` function is as follows:
```matlab
mesh(X, Y, Z)
mesh(X, Y, Z, C)
mesh(X, Y, Z, C, 'PropertyName', PropertyValue, ...)
```
**Parameter Details**
***X, Y, Z:** The x, y, and z coordinate data for the surface, which can be in matrix, vector, or scalar form.
***C:** The color data for the surface, which can be in matrix, vector, or scalar form. If omitted, the default colormap is used.
***'PropertyName', PropertyValue:** Optional property-value pairs to control the appearance and behavior of the surface.
**Common Properties**
| Property | Description |
|---|---|
| EdgeColor | The color of the mesh lines |
| FaceColor | The color of the surface |
| FaceLighting | Whether lighting is enabled |
| LineWidth | The width of the mesh lines |
| Marker | The type of marker for vertices |
| MarkerFaceColor | The fill color for vertices |
| MarkerSize | The size of the vertices |
| Shading | The shading mode |
**Code Example**
```matlab
% Creating a sine surface
[X, Y] = meshgrid(-2:0.1:2);
Z = sin(X.^2 + Y.^2);
% Plotting the surface graph
mesh(X, Y, Z);
```
**Logical Analysis**
This code uses the `meshgrid` function to create the x and y coordinate data for a sine surface, and then uses the `mesh` function to plot the surface graph.
# 4. Advanced Techniques in 3D Surface Plotting
### 4.1 Surface Shading and Texture Mapping
#### 4.1.1 Surface Shading
MATLAB provides various methods for shading surfaces, including:
- **Single-Color Shading:** Specify a single color using the `facecolor` property.
- **Gradient Shading:** Create a color gradient using the `colormap` function and specify the color range with the `caxis` function.
- **Vertex Shading:** Specify the color for each vertex using the `vertexcolors` property.
- **Normal Shading:** Shade according to the normal direction of the surface for a more realistic effect.
#### 4.1.2 Texture Mapping
Texture mapping is a technique that applies images or textures onto surfaces to enhance their visual appearance. MATLAB uses the `texturemap` function for texture mapping.
**Steps:**
1. Load the texture image.
2. Enable texture mapping using the `texturemapping` property.
3. Specify the color mapping using the `colormap` function.
4. Specify the color range using the `caxis` function.
### 4.2 Surface Lighting and Shadow Effects
Lighting and shadow effects can make surface graphs more realistic and three-dimensional. MATLAB uses the `lighting` function and the `material` function to control lighting and shadows.
#### 4.2.1 Types of Lighting
MATLAB supports the following types of lighting:
- **Parallel Light:** Parallel rays coming from an infinite distance.
- **Point Light:** A light source that radiates from a single point.
- **Spot Light:** Light that radiates from within a conical area.
#### 4.2.2 Material Properties
Material properties control how surfaces reflect light, including:
- **Ambient Light:** The amount of light a surface receives from the environment.
- **Diffuse Reflection:** The ability of a surface to reflect light from various directions.
- **Specular Reflection:** The ability of a surface to reflect light from a specific direction.
### 4.3 Surface Animation and Interaction
MATLAB provides various methods for creating 3D surface plot animations and interactions, including:
#### 4.3.1 Surface Animation
- **Changing Surface Parameters:** Dynamically alter the parameters of the surface, such as equations or parametrization.
- **Rotating the Surface:** Rotate the surface around any axis.
- **Scaling the Surface:** Zoom in or out of the surface.
#### 4.3.2 Surface Interaction
- **Interactive Rotation:** Rotate the surface interactively using the mouse or keyboard.
- **Interactive Scaling:** Scale the surface interactively using the mouse or keyboard.
- **Interactive Translation:** Translate the surface interactively using the mouse or keyboard.
# 5. Application Cases of MATLAB 3D Surface Plotting
### 5.1 Three-Dimensional Visualization in Scientific Computing
In the field of scientific computing, three-dimensional surface plotting is widely used for visualizing complex datasets. For example, in fluid dynamics, 3D surface plots can represent the distribution of fluid velocity and pressure. In electromagnetism, 3D surface plots can be used to visualize the intensity and direction of electromagnetic fields.
### 5.2 Three-Dimensional Modeling in Engineering Design
In engineering design, three-dimensional surface plotting is a valuable tool for creating and visualizing complex geometric models. For instance, in mechanical engineering, 3D surface plots can be used for designing and simulating mechanical components. In architectural engineering, 3D surface plots can be used to create virtual models of buildings.
### 5.3 Three-Dimensional Reconstruction in Medical Imaging
In the field of medical imaging, three-dimensional surface plotting is used to reconstruct three-dimensional structures from two-dimensional images. For example, in computerized tomography (CT) and magnetic resonance imaging (MRI), 3D surface plots can be used to reconstruct detailed models of organs and tissues. These models can be utilized for diagnosis, treatment planning, and surgical simulation.
0
0