【Practical Exercise】MATLAB Practical: Extracting Image Contours Using Edge Detection Algorithms
发布时间: 2024-09-15 03:39:41 阅读量: 23 订阅数: 52 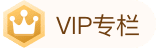
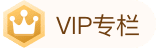
# 2.1 Sobel Operator
The Sobel operator is an edge detection algorithm based on gradient calculation. It utilizes two 3x3 convolution kernels to compute the gradients of an image in the horizontal and vertical directions, respectively.
```
Gx = [ -1, 0, 1;
-2, 0, 2;
-1, 0, 1 ];
Gy = [ 1, 2, 1;
0, 0, 0;
-1, -2, -1 ];
```
Where:
* `Gx`: Convolution kernel for horizontal gradient
* `Gy`: Convolution kernel for vertical gradient
Applying these two convolution kernels to convolve with an image results in a horizontal gradient map `Gx` and a vertical gradient map `Gy`. The intensity of edges can be calculated using the following formula:
```
G = sqrt(Gx.^2 + Gy.^2)
```
# 2. Principles of Edge Detection Algorithms
### 2.1 Sobel Operator
The Sobel operator is a first-order differential operator used for detecting horizontal and vertical edges in an image. It employs two 3x3 convolution kernels to detect gradients in the horizontal and vertical directions:
```
Gx = [1 0 -1; 2 0 -2; 1 0 -1]
Gy = [1 2 1; 0 0 0; -1 -2 -1]
```
**Parameter Explanation:**
* `Gx`: Convolution kernel for horizontal gradient
* `Gy`: Convolution kernel for vertical gradient
**Code Logic:**
1. Convolve the kernels with the image to obtain horizontal and vertical gradient images.
2. Calculate the gradient magnitude: `G = sqrt(Gx^2 + Gy^2)`
3. Determine the edges in the image based on the gradient magnitude and thresholds.
### 2.2 Canny Operator
The Canny operator is a multi-stage edge detection algorithm that combines techniques such as noise reduction, gradient computation, and non-maximum suppression. The main steps are as follows:
1. **Noise Reduction:** Smooth the image using a Gaussian filter to remove noise.
2. **Gradient Computation:** Use the Sobel operator to calculate the horizontal and vertical gradients of the image.
3. **Non-Maximum Suppression:** Along the gradient direction, retain only the points with the maximum gradient magnitude and suppress others.
4. **Thresholding:** Use two thresholds to classify the gradient magnitudes into strong edges, weak edges, and non-edges.
5. **Edge Linking:** Connect strong and weak edges to form complete edges.
**Code Logic:**
```python
import cv2
def canny(image, sigma=1.4, low_threshold=0.05, high_threshold=0.1):
# Noise Reduction
blurred_image = cv2.GaussianBlur(image, (5, 5), sigma)
# Gradient Computation
edges = cv2.Canny(blurred_image, low_threshold * 255, high_threshold * 255)
return edges
```
**Parameter Explanation:**
* `image`: Input image
* `sigma`: Standard deviation of the Gaussian filter
* `low_threshold`: Lower threshold used to suppress noise
* `high_threshold`: Higher threshold used to preserve strong edges
### 2.3 Laplace Operator
The Laplace operator is a second-order differential operator used for detecting edges and spots in an image. It uses the following 3x3 convolution kernel:
```
Laplacian = [0 1 0; 1 -4 1; 0 1 0]
```
**Parameter Explanation:**
* `Laplacian`: Laplace operator convolution kernel
**Code Logic:**
1. Convolve the Laplace operator with the image to obtain the second-order derivative.
2. Determine the edges and spots in the image based on the sign of the second-order derivative.
**Table: Comparison of Edge Detection Algorithms**
| Algorithm | Pros | Cons |
|---|---|---|
| Sobel | Simple computation, fast | Sensitive to noise |
| Canny | Good edge detection effect, strong noise resistance | Complex computation, slow |
| Laplace | Can detect edges and spots | Sensitive to noise, prone to produce false edges |
**Mermaid Flowchart: Edge Detection Algorithm Process**
```mermaid
graph LR
subgraph Sobel
Sobel[Sobel operator] --> Gx[Horizontal Gradient]
Sobel[Sobel operator] --> Gy[Vertical Gradient]
Gx[Horizontal Gradient] --> G[Gradient Magnitude]
Gy[Vertical Gradient] --> G[Gradient Magnitude]
G[Gradient Magnitude] --> Edges[Edges]
end
subgraph Canny
Canny[Canny operator] --> Blurred[Noise Reduction]
Blurred[Noise Reduction] --> Edges[Gradient Computation]
Edges[Gradient Computation] --> NonMax[Non-Maximum Suppression]
NonMax[Non-Maximum Suppression] --> Threshold[Thresholding]
Threshold[Thresholding] --> Edges[Edge Linking]
end
subgraph Laplace
Laplace[Laplace operator] --> D2[
```
0
0
相关推荐
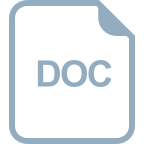
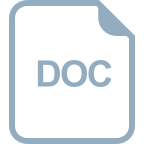
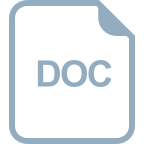
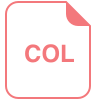
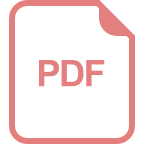
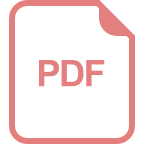
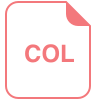
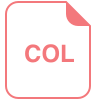
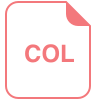
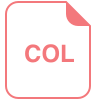