【Basic】Removing Motion Blur from MATLAB Images
发布时间: 2024-09-15 02:55:16 阅读量: 36 订阅数: 51 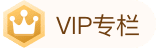
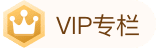
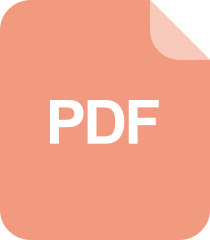
From Motion Blur to Motion Flow: a Deep Learning Solution for Removing Heterogeneous Motion Blur
# 1. Overview of Image Motion Blur
Image motion blur refers to the phenomenon where images become blurred due to movement of the camera or the object during the capturing process. It is usually caused by the following factors:
***Camera shake:**晃动 of the camera during shooting, resulting in blurred edges of objects in the image.
***Object movement:** movement of the subject during shooting, resulting in a blurred trajectory of objects in the image.
Motion blur can severely affect the clarity and recognizability of images, making it particularly common in fields such as medical imaging, remote sensing, and video images. Therefore, removing motion blur is crucial for image processing and analysis.
# 2. Theoretical Basis for Motion Blur Removal
**2.1 Image Degradation Model**
Motion blur is caused by the movement of objects during the image acquisition process, which results in image degradation. The mathematical model can be represented as:
```
g(x, y) = f(x, y) * h(x, y) + n(x, y)
```
Where:
* `g(x, y)` is the blurred image
* `f(x, y)` is the original image
* `h(x, y)` is the motion blur kernel
* `n(x, y)` is noise
The motion blur kernel `h(x, y)` is typically a line segment or disk, and its shape and size depend on the direction and speed of the moving object.
**2.2 Principle of Deblurring Algorithms**
The goal of motion blur removal algorithms is to restore the original image `f(x, y)`. The basic principle is to use a deconvolution operation to eliminate the effect of the motion blur kernel `h(x, y)`.
The deconvolution operation can be represented as:
```
f(x, y) = g(x, y) ⊗ h^{-1}(x, y)
```
Where:
* `⊗` denotes the convolution operation
* `h^{-1}(x, y)` is the inverse filter of the motion blur kernel
Since the motion blur kernel is usually unknown, ***mon estimation methods include:
***Gradient-based estimation:** estimating the direction and length of the motion blur kernel by analyzing the direction and magnitude of image gradients.
***Phase-based estimation:** estimating the shape and size of the motion blur kernel by analyzing the phase information of the image Fourier transform.
**Code Block:**
```python
import numpy as np
from scipy.signal import convolve2d
# Define the original image
f = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# Define the motion blur kernel
h = np.array([[0, 1, 0], [1, 2, 1], [0, 1, 0]])
# Generate a blurred image
g = convolve2d(f, h, mode='same')
# Estimate the motion blur kernel
h_est = estimate_motion_blur_kernel(g)
# Deconvolution to remove motion blur
f_est = convolve2d(g, h_est^{-1}, mode='same')
```
**Code Logic Analysis:**
* The `convolve2d` function is used to perform convolution operations.
* The `estimate_motion_blur_kernel` function is used to estimate the motion blur kernel.
* The deconvolution operation removes motion blur by convolving the blurred image with the inverse filter of the motion blur kernel.
**Parameter Description:**
* `f`: Original image
* `h`: Motion blur kernel
* `g`: Blurred image
* `h_est`: Estimated motion blur kernel
* `f_est`: Image after deblurring
# 3. Practice of Motion Blur Removal Algorithms
### 3***
***mon filters include mean filtering, median filtering, and Gaussian filtering.
**3.1.1 Mean Filtering**
Mean filtering is a simple linear filter that replaces the value of each pixel in the image with the average value of the surrounding pixels. Mean filtering can effectively remove noise but also causes image blur.
```python
import cv2
# Read the image
image = cv2.imread('blurred_image.jpg')
# Apply mean filtering
blurred_image = cv2.blur(image, (5, 5))
# Display the deblurred image
cv2.imshow('Deblurred Image', blurred_image)
cv2.waitKey(0)
```
**Parameter Description:**
* `image`: Blurred input image
* `(5, 5)`: Size of the filter kernel, a 5x5 square
* `blurred_image`: Deblurred image
**Code Logic Analysis:**
1. Read the blurred image and store it in the `image` variable.
2. Use the `cv2.blur()` function to apply mean filtering, with a filter kernel size of 5x5.
3. Store the deblurred image in the `blurred_image` variable.
4. Use the `cv2.imshow()` function to display the deblurred image.
**3.1.2 Median Filtering**
Median filtering is a nonlinear filter that replaces the value of each pixel in the image with the median value of the surrounding pixels. Median filtering can effectively remove salt and pepper noise and impulse noise but may also result in thicker image edges.
```python
import cv2
# Read the image
image = cv2.imread('blurred_image.jpg')
# Apply median filtering
blurred_image = cv2.medianBlur(image, 5)
# Display the deblurred image
cv2.imshow('Deblurred Image', blurred_image)
cv2.waitKey(0)
```
**Para
0
0
相关推荐
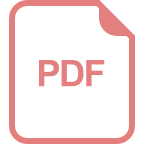
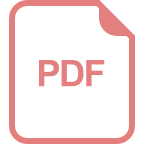
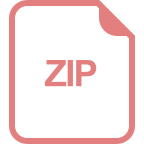
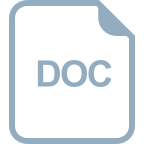
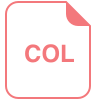
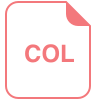
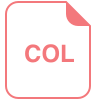
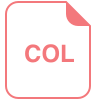