【Basic】Image Thresholding in MATLAB: Implementing the Otsu Thresholding Method
发布时间: 2024-09-15 02:23:54 阅读量: 34 订阅数: 50 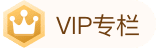
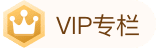
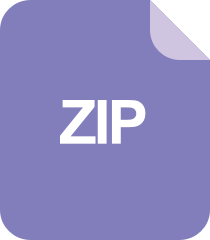
Hyperspectral Image Classification via Basic Thresholding Classifier:Hyperspectral Image Classification via Basic Thresholding Classifier-matlab开发
# 2.1 The Theoretical Foundation of the Otsu Thresholding Method
### 2.1.1 Intra-Class Variance and Inter-Class Variance
The Otsu thresholding method is based on the concepts of intra-class variance and inter-class variance. Intra-class variance measures the extent of differences between pixel values and the class mean within each class, while inter-class variance measures the extent of mean differences between different classes.
### 2.1.2 Otsu Threshold Calculation Formula
The Otsu threshold calculation formula aims to maximize the ratio of inter-class variance to intra-class variance:
```
σ²_B / σ²_W = (μ_1 - μ_2)² / (σ_1² + σ_2²)
```
Where:
* σ²_B is the inter-class variance
* σ²_W is the intra-class variance
* μ_1 and μ_2 are the means of the two classes
* σ_1² and σ_2² are the variances of the two classes
# 2. The Principle and Implementation of the Otsu Thresholding Method
### 2.1 The Theoretical Foundation of the Otsu Thresholding Method
#### 2.1.1 Intra-Class Variance and Inter-Class Variance
The Otsu thresholding method is an automatic image thresholding method. Its basic principle is to maximize the inter-class variance between the target object and the background in the image, while minimizing the intra-class variance.
***Intra-Class Variance (Within-Class Variance)**: Measures the dispersion degree of pixel grayscale values within the same class (target object or background).
***Inter-Class Variance (Between-Class Variance)**: Measures the extent of difference in pixel grayscale values between different classes (target object and background).
#### 2.1.2 Otsu Threshold Calculation Formula
The Otsu thresholding method calculates the intra-class variance and inter-class variance for each grayscale level in the image, and selects the grayscale value that maximizes the inter-class variance as the threshold.
The Otsu threshold calculation formula is as follows:
```
Otsu Threshold = argmax(σ_B^2(t))
```
Where:
* σ_B^2(t) is the inter-class variance at threshold t
* t is the range of grayscale values in the image
### 2.2 The MATLAB Implementation of the Otsu Thresholding Method
#### 2.2.1 Image Preprocessing
Before applying the Otsu thresholding method, it is usually necessary to preprocess the image to improve the accuracy of threshold segmentation. Preprocessing steps include:
* Grayscale conversion: Convert a color image to a grayscale image.
* Noise removal: Use filters to remove noise from the image.
#### 2.2.2 Otsu Threshold Calculation
The process of calculating the Otsu threshold using MATLAB is as follows:
```matlab
% Read image
image = imread('image.jpg');
% Grayscale conversion
grayImage = rgb2gray(image);
% Calculate Otsu threshold
otsuThreshold = graythresh(grayImage);
% Binarize image
binaryImage = imbinarize(grayImage, otsuThreshold);
```
#### 2.2.3 Binary Image Generation
After calculating the Otsu threshold, the image can be binarized based on the threshold to generate a binary image. In the binary image, pixels with grayscale values greater than or equal to the threshold are set to 1 (white), otherwise set to 0 (black).
```matlab
% Display binary image
figure;
imshow(binaryImage);
title('Binary Image');
```
### Code Logic Analysis
**Code block 1:**
* `grayImage = rgb2gray(image);`: Convert a color image to a grayscale image.
* `otsuThreshold = graythresh(grayImage);`: Calculate the Otsu threshold.
* `binaryImage = imbinarize(grayImage, otsuThreshold);`: Binarize the image based on the threshold.
**Code block 2:**
* `figure;`: Create a new figure window.
* `imshow(binaryImage);`: Display the binary image.
* `title('Binary Image');`: Set the title of the figure window.
### Parameter Explanation
* `image`: The input color image.
* `grayImage`: The converted grayscale image.
* `otsuThreshold`: The calculated Otsu threshold.
* `binaryImage`: The generated binary image.
# 3. Practical Application of the Otsu Thresholding Method
### 3.1 Denoising Noisy Images
#### 3.1.1 Characteristics of Noisy Images
Noisy images refer to images that have been affected by noise during image acquisition or transmission. Noise can degrade image quality and affect subsequent image processing and analysis tasks. Noisy images typically have the following characteristics:
- **Uneven pixel value distribution**: In noisy images, pixel values are unevenly distributed, resulting in some isolated bright or dark pixels.
- **Blurred image details**: Noise can obscure image details, making the image appear blurry.
- **Irregular texture**: The texture of noisy images is irregular, appearing with some random spots or stripes.
#### 3.1.2 Otsu Thresholding Method for Denoising Principle
The Otsu thresholding method can be used to denoise noisy images. Its principle is: by calculating the intra-class variance and inter-class variance at different grayscale levels in the image, find an optimal threshold that divides the image into two categories, one being background noise and the other being the image target. Then, set the background noise
0
0
相关推荐
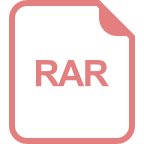
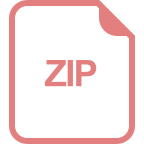
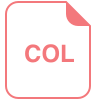
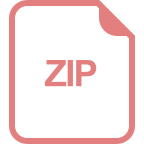
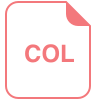
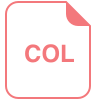
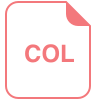
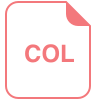