【Basic】Grayscale and Binarization Processing of Images
发布时间: 2024-09-15 02:59:27 阅读量: 15 订阅数: 42 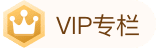
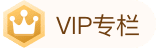
# 2.1 Implementation of Grayscale Processing Algorithms
The primary grayscale processing algorithms are as follows:
**2.1.1 Average Method**
The average method is the simplest grayscale processing algorithm. Its principle is to replace the color value of each pixel with the average value of all the pixels around that pixel. The formula for calculating the average method is as follows:
```python
gray_value = (sum(pixel_values) / num_pixels)
```
Where `gray_value` is the grayscale value, `pixel_values` is the list of color values of the pixel, and `num_pixels` is the number of pixels.
**2.1.2 Weighted Average Method**
The weighted average method is similar to the average method, but it assigns different weights to different pixels. Weights are usually determined based on the distance of the pixels or other factors. The formula for calculating the weighted average method is as follows:
```python
gray_value = (sum(weight * pixel_value) / sum(weight))
```
Here, `weight` is the list of weights, and `pixel_value` is the list of color values of the pixel.
# 2. Practical Application of Image Grayscale Processing
### 2.1 Implementation of Grayscale Processing Algorithms
#### 2.1.1 Average Method
The average method is the simplest grayscale algorithm, setting the grayscale value of each pixel to the average of the grayscale values of all pixels around that pixel.
```python
import cv2
import numpy as np
def grayscale_mean(image):
"""
Grayscale processing using the average method.
Args:
image: Input color image
Returns:
gray_image: Grayscale image after processing
"""
# Get the width and height of the image
height, width = image.shape[:2]
# Create a grayscale image
gray_image = np.zeros((height, width), dtype=np.uint8)
# Loop through each pixel
for i in range(height):
for j in range(width):
# Get the 3x3 neighborhood around the pixel
neighborhood = image[i-1:i+2, j-1:j+2]
# Calculate the average grayscale value around the pixel
mean_value = np.mean(neighborhood)
# Set the pixel's grayscale value
gray_image[i, j] = mean_value
return gray_image
```
**Logical Analysis:**
1. The `grayscale_mean` function takes a color image as input and returns a grayscale image.
2. It first gets the width and height of the image, then creates a grayscale image.
3. Then, it loops through each pixel in the image and gets the 3x3 neighborhood around the pixel.
4. Next, it calculates the average grayscale value around the pixel and sets it as the pixel's grayscale value.
5. Finally, it returns the grayscale image.
#### 2.1.2 Weighted Average Method
The weighted average method is an improved grayscale algorithm that assigns different weights to the grayscale values of different pixels around a pixel. Weights are usually assigned based on the distance of the pixels from the center pixel.
```python
import cv2
import numpy as np
def grayscale_weighted_mean(image):
"""
Grayscale processing using the weighted average method.
Args:
image: Input color image
Returns:
gray_image: Grayscale image after processing
"""
# Get the width and height of the image
height, width = image.shape[:2]
# Create a grayscale image
gray_image = np.zeros((height, width), dtype=np.uint8)
# Define the weight matrix
weights = np.array([[1, 2, 1],
[2, 4, 2],
[1, 2, 1]])
# Loop through each pixel
for i in range(height):
for j in range(width):
# Get the 3x3 neighborhood around the pixel
neighborhood = image[i-1:i+2, j-1:j+2]
# Calculate the weighted average grayscale value around the pixel
weighted_mean_value = np.sum(neighborhood * weights) / np.sum(weights)
# Set the pixel's grayscale value
gray_image[i, j] = weighted_mean_value
return gray_image
```
**Logical Analysis:**
1. The `grayscale_weighted_mean` function takes a color image as input and returns a grayscale image.
2. It first gets the width and height of the image, then creates a grayscale image.
3. Next, it defines a weight matrix, which is used to assign different weights to the grayscale values of different pixels around a pixel.
4. Then, it loops through each pixel in the image and gets the 3x3 neighborhood around the pixel.
5. Next, it calculates the weighted average grayscale value around the pixel and sets it as the pixel's grayscale value.
6. Finally, it returns the grayscale image.
### 2.2 Image Enhancement through Grayscale Processing
#### 2.2.1 Histogram Equalization
Histogram equalization is an image enhancement technique that improves image contrast by adjusting the image's histogram. It redistributes the grayscale values so that each grayscale value occurs with a more uniform frequency.
```python
import cv2
import numpy as np
def histogram_equalization(image):
"""
Histogram equalization for image enhancement.
Args:
image: Input grayscale image
Returns:
enhanced_image: Image after histogram equalization
"""
# Calculate the image histogram
histogram = cv2.calcHist([image], [0], None, [256], [0, 256])
# Calculate the cumulative histogram
cdf = np.cumsum(histogram)
# Normalize the cumulative histogram
cdf_normalized = cdf / cdf[-1]
# Create a mapping table
map_table = np.zeros(256, dtype=np.uint8)
for i in range(256):
map_table[i] = np.round(cdf_normalized[i] * 255)
# Apply the mapping table
enhanced_image = cv2.LUT(image, map_table)
return enhanced_image
```
**Logical Analysis:**
1. The `histogram_equalization` function takes a grayscale image as input and returns an image after histogram equalization.
2. It first calculates the image histogram, then calculates the cumulative histogram.
3. Next, it normalizes the cumulative histogram and creates a mapping table.
4. Then, it applies the mapping table to the image and returns the image after histogram equalization.
#### 2.2.2 Local Contrast Enhancement
Local contrast enhancement is an image enhancement technique that improves image details by adjusting the contrast of local areas of the image. It uses a convolution kernel to calculate the local gradient of each p
0
0
相关推荐
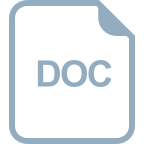
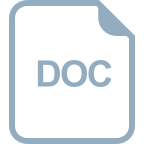
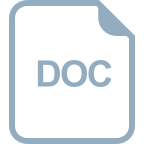
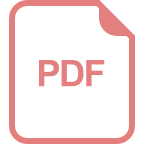
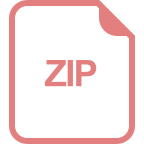
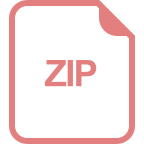
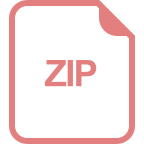
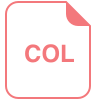
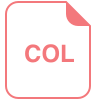