【Basic】Feature Extraction and Matching of MATLAB Images
发布时间: 2024-09-15 02:52:28 阅读量: 32 订阅数: 50 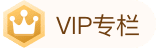
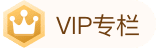
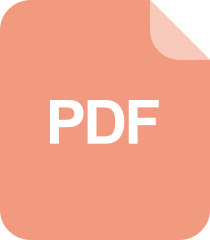
Deep Feature Extraction and Classification of Hyp.pdf
# 1. Basics of Image Feature Extraction
Image feature extraction is a critical technology in the field of computer vision. It provides a foundation for image analysis and understanding by extracting representative information from images. Image features are typically divided into global features and local features. Global features describe the statistical characteristics of an entire image, such as histograms and color covariance matrices; local features describe the characteristics of local regions of an image, such as feature points extracted by SIFT and SURF algorithms.
# 2. Theories of Image Feature Matching
### 2.1 Feature Matching Algorithms
Image ***mon feature matching algorithms include:
#### 2.1.1 Correlation Matching
Correlation matching is based on the similarity of local regions of an image. It calculates the correlation coefficient between two image blocks and finds the matching point with the highest correlation coefficient.
**Code Block:**
```python
import numpy as np
from scipy.ndimage import correlate
def correlate_match(img1, img2):
"""
Correlation matching algorithm
Parameters:
img1 (ndarray): Image 1
img2 (ndarray): Image 2
Returns:
matches (list): Matching point pairs
"""
# Calculate the correlation coefficient
corr = correlate(img1, img2)
# Find the maximum correlation coefficient
max_corr = np.max(corr)
max_idx = np.argmax(corr)
# Get matching points
matches = []
for i in range(max_idx.shape[0]):
matches.append((max_idx[i, 0], max_idx[i, 1]))
return matches
```
**Logical Analysis:**
This code uses the `scipy.ndimage.correlate` function to calculate the correlation coefficient between image blocks. The higher the correlation coefficient, the more similar the image blocks are. The code finds the maximum correlation coefficient and returns the corresponding matching points.
#### 2.1.2 Feature Point Matching
Feature p***mon feature point matching algorithms include SIFT and SURF.
**Code Block:**
```python
import cv2
def feature_match(img1, img2):
"""
Feature point matching algorithm
Parameters:
img1 (ndarray): Image 1
img2 (ndarray): Image 2
Returns:
matches (list): Matching point pairs
"""
# Detect feature points and descriptors
sift = cv2.SIFT_create()
kp1, des1 = sift.detectAndCompute(img1, None)
kp2, des2 = sift.detectAndCompute(img2, None)
# Match feature points
bf = cv2.BFMatcher()
matches = bf.knnMatch(des1, des2, k=2)
# Filter matching points
good_matches = []
for m, n in matches:
if m.distance < 0.75 * n.distance:
good_matches.append(m)
return good_matches
```
**Logical Analysis:**
This code uses the SIFT algorithm from OpenCV to detect feature points and descriptors. It uses `cv2.BFMatcher` to match feature points and filters matching points based on a distance threshold.
### 2.2 Feature Matching Evaluation Metrics
To evaluate the performance of featur
0
0
相关推荐
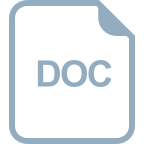
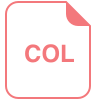
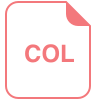
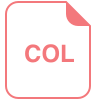
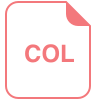
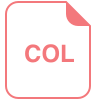
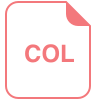
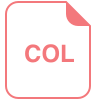
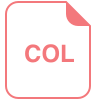