【Advanced Chapter】Image Denoising in MATLAB: Using BM3D Algorithm for Image Denoising
发布时间: 2024-09-15 03:13:54 阅读量: 38 订阅数: 62 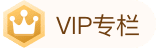
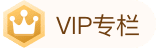
# 2.1 Theoretical Foundation of the BM3D Algorithm
The BM3D algorithm is built upon the concepts of image block matching and collaborative filtering. Its core idea is to decompose the image into overlapping blocks, perform matching and collaborative filtering on each block to remove noise.
BM3D algorithm assumes that the noise is additive Gaussian white noise with a mean of 0 and variance of σ². During the block matching phase, the algorithm uses similarity measures (such as normalized cross-correlation) to find the best matching block for each block in the image.
In the collaborative filtering phase, the algorithm groups the matched blocks and performs collaborative filtering on each group of blocks. Collaborative filtering involves transforming the blocks (e.g., wavelet transform) and then filtering in the transform domain to remove the noise.
# 2. The Principle of the BM3D Image Denoising Algorithm
### 2.1 Theoretical Foundation of the BM3D Algorithm
BM3D (Block-Matching and 3D Transform) algorithm is an image denoising algorithm based on collaborative filtering. It removes noise from the image by dividing the image into small blocks and then performing collaborative filtering on these blocks in the three-dimensional transform domain (spatial domain and transform domain).
The theoretical foundation of the BM3D algorithm is based on the following assumptions:
- The noise in the image is usually additive noise, meaning that the noise is independent of the original image signal.
- Similar blocks in the image have similar noise distributions.
- In the three-dimensional transform domain, similar blocks have sparse representations, meaning that their majority of energy is concentrated in a few transform coefficients.
### 2.2 Steps and Process of the BM3D Algorithm
The BM3D algorithm mainly includes the following steps:
1. **Image Block Division:** Divide the image into overlapping blocks of size 8×8.
2. **Collaborative Filtering:** For each block, find a set of blocks most similar to it in the three-dimensional transform domain (called collaborative blocks).
3. **Three-Dimensional Transform:** Perform three-dimensional transforms (usually wavelet transforms) on each collaborative block.
4. **Collaborative Domain Hard Thresholding:** In the three-dimensional transform domain, apply hard thresholding to the transform coefficients of each collaborative block to remove noise.
5. **Inverse Three-Dimensional Transform:** Perform inverse three-dimensional transforms on the thresholded collaborative blocks to obtain the denoised blocks.
6. **Block Fusion:** Fuse the denoised blocks together to obtain the final denoised image.
**Code Block:**
```matlab
% Image Reading
image = imread('noisy_image.jpg');
% Image Block Division
blocks = im2col(image, [8 8], 'sliding');
% Collaborative Filtering
similar_blocks = find_similar_blocks(blocks);
% Three-Dimensional Transform
transformed_blocks = fft2(blocks);
% Collaborative Domain Hard Thresholding
thresholded_blocks = hard_threshold(transformed_blocks);
% Inverse Three-Dimensional Transform
denoised_blocks = ifft2(thresholded_blocks);
% Block Fusion
denoised_image = col2im(denoised_blocks, [size(image, 1), size(image, 2)], [8 8], 'sliding');
```
**Logical Analysis:**
* The `im2col` function divides the image into overlapping blocks of size 8×8.
* The `find_similar_blocks` function finds the set of blocks most similar to each block.
* The `fft2` function performs two-dimensional fast Fourier transforms on each block.
* The `hard_threshold` function applies hard thresholding to the transform coefficients.
* The `ifft2` function performs inverse two-dimensional fast Fourier transforms on the thresholded blocks.
* The `col2im` function fuses the denoised blocks together to obtain the final denoised image.
**Parameter Description:**
* `'noisy_image.jpg'`: The input noisy image.
* `[8 8]`: The size of the blocks.
* `'sliding'`: The mode of block division, indicating overlapping blocks.
* `'similar_blocks'`: The set of similar blocks.
* `'hard_threshold'`: The hard thresholding function.
* `'denoised_image'`: The image after denoising.
# 3.1 BM3D Algorithm MATLAB Function
0
0
相关推荐








