Image Filtering in MATLAB: Applying Mean Filtering and Median Filtering
发布时间: 2024-09-15 02:19:43 阅读量: 54 订阅数: 60 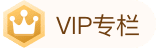
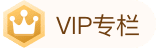
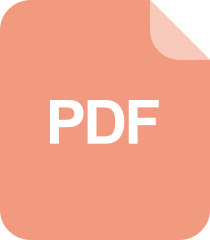
Kalman Filtering Theory and Practice Using MATLAB (2015, 4th).pdf

# 2.1 Principle and Mathematical Foundation of Mean Filtering
Mean filtering is a linear filtering technique that smooths an image by calculating the average value of the pixel values in the neighborhood around each pixel in the image. The mathematical foundation is as follows:
Let the image f(x, y) be a two-dimensional function, with the pixel value of f(x, y). The mean filter uses a convolution kernel h(x, y) to convolve with the image. The convolution kernel is usually a symmetric square or circular window. The convolution operation is as follows:
```
g(x, y) = f(x, y) * h(x, y)
= ∑∑ f(i, j) * h(x - i, y - j)
```
where g(x, y) is the filtered image, h(x, y) is the convolution kernel, and (i, j) are the coordinates of the pixels in the convolution kernel.
For mean filtering, the convolution kernel h(x, y) is usually a constant, indicating that all pixels in the convolution kernel have equal weights. Therefore, the value of each pixel in the output image g(x, y) is the average of the pixel values in its neighborhood.
# 2. Theory and Practice of Mean Filtering
### 2.1 Principle and Mathematical Foundation of Mean Filtering
Mean filtering is a linear filtering technique that replaces the grayscale value of each pixel in the image with the average of the grayscale values of all pixels in the neighborhood around that pixel. This operation can effectively eliminate noise in the image while preserving the main features of the image.
The mathematical foundation of mean filtering can be expressed as:
```
g(x, y) = (1 / (2k + 1)^2) * ΣΣ f(x + i, y + j)
```
where:
* `g(x, y)` is the filtered pixel value
* `f(x + i, y + j)` is the pixel value in the neighborhood
* `k` is the neighborhood radius
### 2.2 Implementation of Mean Filtering in MATLAB
#### 2.2.1 Using the imfilter Function for Mean Filtering
MATLAB provides the `imfilter` function, which can conveniently implement mean filtering. The syntax is as follows:
```
imout = imfilter(imin, h)
```
where:
* `imin` is the input image
* `h` is the filter kernel
* `imout` is the output image
The filter kernel for mean filtering is a square matrix, with all elements valued at 1/((2k + 1)^2). For example, for a 3x3 mean filter kernel, the elements would be:
```
h = [1/9, 1/9, 1/9;
1/9, 1/9, 1/9;
1/9, 1/9, 1/9]
```
Here's an example of using the `imfilter` function for mean filtering:
```
% Read the input image
I = imread('image.jpg');
% Create a mean filter kernel
h = fspecial('average', 3);
% Apply mean filtering
J = imfilter(I, h);
% Display the filtered image
imshow(J);
```
#### 2.2.2 Optimizing the Algorithm and Parameters of Mean Filtering
To improve the efficiency and effectiveness of mean filtering, the algorithm and parameters can be optimized.
**Algorithm Optimization:**
***Fast Fourier Transform (FFT):** Using FFT can convert the image convolution operation into frequency domain multiplication, significantly improving computational efficiency.
***Integral Image:** By pre-calculating the integral image of the image, the pixel sum of any rectangular area can be quickly calculated, thus optimizing the mean filtering computation process.
**Parameter Optimization:**
***Filter Kernel Size:** The larger the filter kernel, the better the noise removal effect, but the image details may also be
0
0
相关推荐







