【Basic】Basic Image Plotting in MATLAB: Plotting 2D Function Images
发布时间: 2024-09-15 02:12:28 阅读量: 25 订阅数: 60 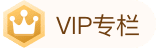
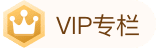
# 2.1 Fundamental Principles of Function Graph Plotting
### 2.1.1 Coordinate Systems and Pixel Coordinates
Graph plotting in MATLAB is based on the Cartesian coordinate system, with the x-axis horizontal and the y-axis vertical. Each pixel in an image is uniquely determined by its position in the coordinate system. Pixel coordinates are represented by integers, with the top-left pixel coordinate being (1, 1), and the bottom-right pixel coordinate being (width, height), where width and height are the width and height of the image, respectively.
### 2.1.2 The Process of Graph Plotting
The process of graph plotting involves the following steps:
1. **Data Preparation:** Convert function data into MATLAB variables.
2. **Coordinate Transformation:** Convert data points from Cartesian coordinates to pixel coordinates.
3. **Pixel Coloring:** Set the color of each pixel based on the value of the data points.
4. **Image Generation:** Combine the colored pixels to form a complete image.
# 2. Theory and Practice of 2D Function Graph Plotting
### 2.1 Fundamental Principles of Function Graph Plotting
#### 2.1.1 Coordinate Systems and Pixel Coordinates
In MATLAB, graph plotting is based on the Cartesian coordinate system. The origin of the coordinate system is located at the bottom-left of the image, with the x-axis extending to the right and the y-axis extending upwards.
An image is composed of pixels, each with its own coordinate value. Pixel coordinates are relative to the top-left corner of the image, with the top-left pixel's coordinates being (1, 1).
#### 2.1.2 The Process of Graph Plotting
The process of plotting a function graph can be divided into the following steps:
1. **Define the Function:** Use MATLAB syntax to define the function to be plotted.
2. **Create the Canvas:** Use the `figure` command to create a canvas, specifying the size and position of the image.
3. **Draw the Image:** Use the `plot` function to draw the function data onto the canvas.
4. **Set Attributes:** Use the attributes of the `plot` function (such as `xlabel`, `ylabel`) to customize the appearance of the image.
5. **Display the Image:** Use the `imshow` command to display the image on the canvas.
### 2.2 MATLAB Syntax for Function Graph Plotting
#### 2.2.1 Basic Usage of the `plot()` Function
The `plot` function is the basic function in MATLAB for plotting function graphs. Its syntax is as follows:
```
plot(x, y)
```
Where:
* `x`: Data for the x-axis.
* `y`: Data for the y-axis.
For example, to plot the graph of the function `y = x^2`:
```
x = linspace(-5, 5, 100);
y = x.^2;
plot(x, y)
```
#### 2.2.2 Attribute Settings for the `plot()` Function
The `plot` ***mon attribute setting functions include:
* `xlabel`: Sets the x-axis label.
* `ylabel`: Sets the y-axis label.
* `title`: Sets the title of the image.
* `LineWidth`: Sets the line width.
* `Color`: Sets the line color.
For example, setting the image title to "Quadratic Function Graph":
```
plot(x, y)
title('Quadratic Function Graph')
```
# 3.1 Image Scaling and Translation
#### 3.1.1 Scaling of Coordinate Axes
MATLAB provides various methods for scaling coordinate axes, including:
- `xlim()` and `ylim()` functions: Set the range of the x-axis and y-axis.
- `axis()` function: Set the range and scale of the coordinate axes.
- `zoom()` function: Interactively scale the coordinate axes.
**Code Block:**
```
% Set the range of the x-axis to [0, 10]
xlim([0, 10]);
% Set the range of the y-axis to [-5, 5]
ylim([-5, 5]);
% Set the scale of the x-axis to 1
set(gca, 'XTick', 0:1:10);
% Set the scale of the y-axis to 2
set(gca, 'YTick', -5:2:5);
% Interactive scaling
zoom on;
```
**Logical Analysis:**
* The `xlim()` and `ylim()` functions set the range of the coordinate axes, specifying the minimum and maximum values.
* The `axis()` function sets the range and scale of the coordinate axes, allowing for the specification of scale intervals and scale labels.
* The `set(gca, 'XTick')` and `set(gca, 'YTick')` functions set the scale of the coordinate axes, specifying the scale values.
* The `zoom on` command enables interactive scaling, allowing users to zoom in on the coordinate axes using a mouse.
#### 3.1.2 Image Translation
MATLAB provides the `pan()` function for translating images.
**Code Block:**
```
% Translate the image 2 units to the right
pan x 2;
% Translate the image 1 unit upwards
pan y 1;
% Interactive translation
pan on;
```
**Logical Analysis:**
* The `pan x` and `pan y` functions translate the image, specifying the distance to translate along the x-axis or y-axis.
* The `pan on` command enables interactive translation, allowing users to translate the image using a mouse.
# 4. Practical Applications of Function Graph Plotting
### 4.1 Image Plotting in Scientific Computing
#### 4.1.1 Time Domain and Frequency Domain Images of Signals
In scientific computing, image plotting is often used to visualize the time domain and frequency domain characteristics of signals.
**Time Domain Image** represents the changes in a signal over time. In MATLAB, the `plot()` function can be used to draw time domain images. For example, to draw the time domain image of a sine signal:
```matlab
t = 0:0.01:10;
y = sin(2*pi*1*t);
plot(t, y);
xlabel('Time (s)');
ylabel('Amplitude');
title('Time Domain Image of a Sine Signal');
```
**Frequency Domain Image** represents the distribution of a signal in the frequency domain. In MATLAB, the `fft()` function can be used to compute the signal's spectrum, and then the `plot()` function can be used to draw the frequency domain image. For example, to draw the frequency domain image of a sine signal:
```matlab
Y = fft(y);
f = (0:length(Y)-1)*(1/t(end));
plot(f, abs(Y));
xlabel('Frequency (Hz)');
ylabel('Amplitude');
title('Frequency Domain Image of a Sine Signal');
```
#### 4.1.2 Distribution Images of Statistical Data
Image plotting can also be used to visualize the distribution of statistical data. For example, to draw the probability density function (PDF) image of a normal distribution:
```matlab
mu = 0;
sigma = 1;
x = -3:0.01:3;
y = normpdf(x, mu, sigma);
plot(x, y);
xlabel('x');
ylabel('Probability Density');
title('Probability Density Function Image of a Normal Distribution');
```
### 4.2 Image Plotting in Image Processing
#### 4.2.1 Image Grayscale Level Histogram
The image grayscale level histogram shows the number of pixels for each grayscale level in an image. In MATLAB, the `imhist()` function can be used to plot the grayscale level histogram. For example, to plot the grayscale level histogram of an image:
```matlab
I = imread('image.jpg');
imhist(I);
xlabel('Grayscale Level');
ylabel('Number of Pixels');
title('Grayscale Level Histogram of an Image');
```
#### 4.2.2 Image Edge Detection and Contour Extraction
Image edge detection and contour extraction are important techniques in image processing. In MATLAB, the `edge()` function can be used for edge detection, and then the `bwboundaries()` function can be used to extract contours. For example, to detect the edges of an image and extract contours:
```matlab
I = imread('image.jpg');
edges = edge(I, 'canny');
[B, L] = bwboundaries(edges);
figure;
imshow(I);
hold on;
for i = 1:length(B)
boundary = B{i};
plot(boundary(:,2), boundary(:,1), 'r', 'LineWidth', 2);
end
title('Image Edge Detection and Contour Extraction');
```
# 5.1 3D Function Graph Plotting
### 5.1.1 `surf()` Function and `mesh()` Function
In MATLAB, the `surf()` and `mesh()` functions can be used to plot 3D function graphs. The `surf()` function generates a colored surface, while the `mesh()` function generates a mesh surface.
```
% Define a 3D function
[X, Y] = meshgrid(-2:0.1:2);
Z = X.^2 + Y.^2;
% Use `surf()` to plot a 3D surface
figure;
surf(X, Y, Z);
title('3D Surface Plotted with `surf()`');
xlabel('X');
ylabel('Y');
zlabel('Z');
% Use `mesh()` to plot a 3D mesh
figure;
mesh(X, Y, Z);
title('3D Mesh Plotted with `mesh()`');
xlabel('X');
ylabel('Y');
zlabel('Z');
```
### 5.1.2 Rotation and Scaling of 3D Images
The plotted 3D images can be rotated using the `view()` function and scaled using the `campos()` function.
```
% Rotate a 3D image
figure;
surf(X, Y, Z);
view(3); % Rotate the image to show it from a 3D perspective
title('Rotated 3D Surface');
% Scale a 3D image
figure;
surf(X, Y, Z);
campos([10, 10, 10]); % Scale the image to be displayed from the perspective of [10, 10, 10]
title('Scaled 3D Surface');
```
### 5.1.3 Illumination of 3D Images
MATLAB provides the `light` and `lighting` functions to control the illumination effects of 3D images.
```
% Add a light source
figure;
surf(X, Y, Z);
light('Position', [10, 10, 10]); % Add a light source at position [10, 10, 10]
title('3D Surface with Added Light Source');
% Set the lighting model
figure;
surf(X, Y, Z);
lighting phong; % Set the lighting model to Phong
title('3D Surface with Set Lighting Model');
```
# 6. Performance Optimization of MATLAB Image Plotting
### 6.1 Optimization of Image Plotting Algorithms
#### 6.1.1 Sparse Matrix Plotting
For sparse matrices (i.e., matrices with most elements being zero), specialized sparse matrix plotting algorithms can be used to improve performance. MATLAB provides the `spy()` function, which can quickly plot the distribution of non-zero elements in a sparse matrix.
```
% Create a sparse matrix
A = sparse(1000, 1000, 0.01);
% Use `spy()` to plot the sparse matrix
spy(A);
```
#### 6.1.2 Block Plotting
For large images, they can be divided into multiple smaller blocks and then plotted individually. This method of block plotting can reduce the memory overhead of plotting all pixels at once, thereby improving performance.
```
% Create a large image
image = randn(10000, 10000);
% Divide the image into 100 blocks
blocks = mat2cell(image, 100 * ones(1, 100), 100 * ones(1, 100));
% Plot the image block by block
for i = 1:100
for j = 1:100
subplot(10, 10, i + (j - 1) * 10);
imshow(blocks{i, j});
end
end
```
### 6.2 Selection of Image File Formats
#### 6.2.1 Pros and Cons of Different Image Formats
Different image file formats have different pros and cons, and the choice depends on the intended use of the image and performance requirements.
| Format | Pros | Cons |
|---|---|---|
| PNG | Lossless compression, supports transparency | Larger file size |
| JPEG | Lossy compression, smaller file size | Introduces distortion |
| GIF | Lossless compression, supports animation | Limited color range |
| TIFF | Lossless compression, supports multiple layers | Larger file size |
#### 6.2.2 Image File Compression and Optimization
By compressing and optimizing image files, the file size can be reduced, thereby speeding up loading and transmission. MATLAB provides various image compression and optimization functions, such as `imwrite()` and `imresize()`.
```
% Compress the image into PNG format
imwrite(image, 'image.png', 'Quality', 90);
% Resize the image
image_resized = imresize(image, 0.5);
```
0
0