Edge Detection in MATLAB: Utilizing Sobel and Canny Operators
发布时间: 2024-09-15 02:21:05 阅读量: 24 订阅数: 38 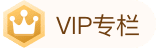
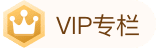
# 1. Overview of Image Edge Detection
Image edge detection is a technique in image processing that identifies the boundaries and contours of objects within an image. It achieves this by detecting sudden changes in the pixel brightness or color values. Edge detection is crucial for computer vision tasks such as image segmentation, object detection, and feature extraction.
Image edges can be categorized into two types: step edges and roof edges. Step edges are where pixel brightness or color values experience abrupt changes, while roof edges occur where pixel values change gradually. Edge detection operators typically use convolution kernels to detect edges in an image. A convolution kernel is a small matrix with elements representing weights used to perform a convolution operation with the pixels in the image.
# 2. Sobel Edge Detection Operator
### 2.1 Principle of the Sobel Operator
The Sobel operator is a first-order differential operator used to detect edges in an image. It employs two 3x3 kernels to calculate the gradients in the horizontal and vertical directions.
**Horizontal direction kernel:**
```
[-1, 0, 1]
[-2, 0, 2]
[-1, 0, 1]
```
**Vertical direction kernel:**
```
[-1, -2, -1]
[0, 0, 0]
[1, 2, 1]
```
The principle of the Sobel operator involves convolving these kernels with the image to perform convolution operations. The convolution operation multiplies each pixel with the kernel elements and sums up the results to obtain the gradient value of that pixel.
**Horizontal gradient:**
```
Gx = I * Hx
```
**Vertical gradient:**
```
Gy = I * Hy
```
Where:
* I is the input image
* Hx and Hy are the horizontal and vertical Sobel kernels
* Gx and Gy are the gradients in the horizontal and vertical directions
### 2.2 Implementation of the Sobel Operator
The implementation of the Sobel operator can be divided into the following steps:
1. Convolve the Sobel kernel with the image to obtain horizontal and vertical gradients.
2. Calculate the gradient magnitude:
```
G = sqrt(Gx^2 + Gy^2)
```
3. Calculate the gradient direction:
```
theta = arctan(Gy / Gx)
```
4. Perform non-maximum suppression on the gradient magnitude:
```
G_nms = NMS(G, theta)
```
5. Apply double thresholding to the suppressed gradient magnitude:
```
G_thresh = Threshold(G_nms, T1, T2)
```
Where:
* G_nms is the gradient magnitude after non-maximum suppression
* T1 and T2 are the double thresholds
* G_thresh is the gradient magnitude after double thresholding
**Code block:**
```python
import cv2
import numpy as np
def sobel_edge_detection(image):
# Convert to grayscale image
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Sobel kernels
sobel_x = np.array([[-1, 0, 1],
[-2, 0, 2],
[-1, 0, 1]])
sobel_y = np.array([[-1, -2, -1],
[0, 0, 0],
[1, 2, 1]])
# Convolution operation
Gx = cv2.filter2D(gray, -1, sobel_x)
Gy = cv2.filter2D(gray, -1, sobel_y)
# Gradient magnitude and direction
G = np.sqrt(Gx**2 + Gy**2)
theta = np.arctan2(Gy, Gx)
# Non-maximum suppression
G_nms = non_max_suppression(G, theta)
# Double thresholding
G_thresh = double_thresholding(G_nms, 0.05, 0.1)
# Return edge image
return G_thresh
```
**Code Logic Analysis:**
* The `sobel_edge_detection` function takes an image as input and returns an edge image.
* The function first converts the image to a grayscale image.
* It then uses the `cv2.filter2D` function with Sobel kernels to perform convolution operations, obtaining horizontal and vertical gradients.
* Next, it calculates the gradient magnitude and direction.
* It then performs non-maximum suppression on the gradient magnitude to eliminate non-edge pixels.
* Finally, it applies double thresholding to the suppressed gradient magnitude to generate the final edge image.
**Parameter Description:**
* `image`: Input image
* `T1`: Low threshold
* `T2`: High threshold
# 3.1 Principle of the Canny Operator
The Canny edge detection operator is a multi-stage edge detection algorithm that aims to detect edges in images with a low error rate and good localization. Proposed by John Canny in 1986, this algorithm has been widely adopted in image processing and computer vision due to its excellent performance.
The principle of the Canny operator mainly involves the following steps:
1. **Gaussian Filtering:** First, apply Gaussian filtering to the image to smooth it and remove noise. The Gaussian filter is a linear filter that smooths the image through a convolution operation, reducing the differences between pixels.
2. **Compute Image Gradients:** Use the Sobel operator or other gradient operators to c
0
0
相关推荐
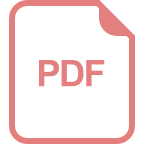
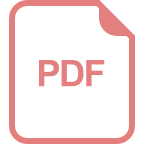
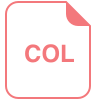
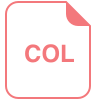
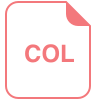
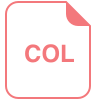
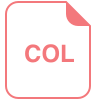
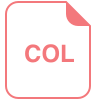