【Advanced】Image Segmentation in MATLAB: Using GrabCut Algorithm for Image Segmentation
发布时间: 2024-09-15 03:07:18 阅读量: 65 订阅数: 60 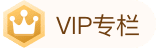
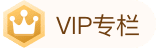
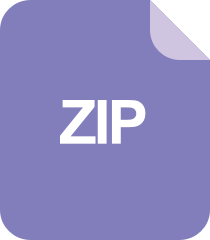
Object segmentation using graph cuts based active contours相关文章
# 2.1 Basic Concepts of Image Segmentation
Image segmentation is a crucial task in computer vision, aimed at dividing an image into regions or objects with distinct attributes. It is widely applied in various fields such as medical image analysis, object detection, and video segmentation.
Image segmentation algorithms typically categorize pixels based on their similarity or differences. Algorithms focusing on similarity group pixels with similar features together, like color, texture, or shape. In contrast, ***
***monly used image segmentation algorithms include threshold segmentation, region growing segmentation, clustering segmentation, and graph-based segmentation. Threshold segmentation classifies pixels into two categories based on their grayscale values or other features. Region growing segmentation starts with a seed pixel and progressively adds neighboring pixels with similar features to the region. Clustering segmentation groups pixels into different clusters based on their similarities. Graph-based segmentation represents an image as a graph, where pixels are nodes, and similarities between pixels are edges.
# 2.2 Principles of the GrabCut Algorithm
### 2.1 Basic Concepts of Image Segmentation
Image segmentation is the process of decomposing an image into multiple regions or objects, with each region or object representing a set of pixels with similar features in the image. It has broad applications in computer vision, medical imaging, remote sensing, and other areas.
### 2.2 Workflow of the GrabCut Algorithm
The GrabCut algorithm is an interactive image segmentation algorithm that allows users to guide the segmentation process by designating foreground and background pixels. The workflow of the GrabCut algorithm is as follows:
1. **Initialization:** The user specifies a set of foreground pixels and a set of background pixels on the image.
2. **Graph-Theoretic Modeling:** Represent image pixels as nodes in a graph and establish edges based on pixel similarities.
3. **Energy Minimization:** Define an energy function to assess the quality of the segmentation result. The energy function consists of a data term and a regularization term. The data term penalizes pixels inconsistent with the user-specified labels, while the regularization term penalizes the complexity of the segmentation result.
4. **Graph Cuts:** Utilize graph cuts to minimize the energy function, thereby obtaining the image segmentation result.
### 2.3 Energy Function in GrabCut Algorithm
The energy function in the GrabCut algorithm is defined as:
```
E(L) = αE_d(L) + βE_s(L)
```
Where:
- `E(L)` is the energy function
- `L` is the segmentation label, where `L(i) = 1` indicates pixel `i` is the foreground, and `L(i) = 0` indicates pixel `i` is the background
- `E_d(L)` is the data term, which measures the consistency of the segmentation result with user-specified labels
- `E_s(L)` is the regularization term, which penalizes the complexity of the segmentation result
- `α` and `β` are weight parameters
**Data Term:**
```
E_d(L) = ∑_i V(L(i), L_u(i))
```
Where:
- `V(L(i), L_u(i))` is the data term for pixel `i`
- `L_u(i)` is the user-specified label for pixel `i`
**Regularization Term:**
```
E_s(L) = ∑_i ∑_j w(i, j) |L(i) - L(j)|
```
Where:
- `w(i, j)` is the weight between pixels `i` and `j`
- `|L(i) - L(j)|` is the label difference between pixels `i` and `j`
The weight parameters `α` and `β` control the importance of the data term and the regularization term in the energy function. By adjusting these parameters, the smoothness of the segmentation result and the fidelity to user-specified labels can be controlled.
# 3.1 Implementation of GrabCut Algorithm in MATLAB
#### MATLAB Code Implementation
```matlab
% Read in the image
I = imread('image.jpg');
% Initialize the GrabCut algorithm
gc = GrabCut();
% Perform interactive segmentation
gc.InteractiveSegmentation(I);
% Obtain the segmentation result
segmentation = gc.Segmentation;
% Display the segmentation result
figure;
imshow(segmentation);
```
#### Code Logic Analysis
- **imread('image.jpg')**: Reads in the im
0
0
相关推荐







