【Advanced Chapter】Image Fusion in MATLAB: Using Multiscale Transform for Image Fusion
发布时间: 2024-09-15 03:06:08 阅读量: 45 订阅数: 59 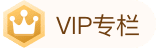
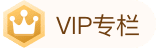
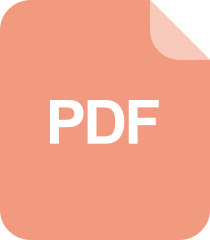
Multifocus image fusion scheme based on features of multiscale products and PCNN in lifting stationary wavelet domain
# 2.1 Wavelet Transform
### 2.1.1 Basic Principles of Wavelet Transform
Wavelet transform is a time-frequency analysis technique that analyzes signals by decomposing them into a series of small-scale wave functions known as wavelets. Wavelet functions are characterized by localization and oscillation, enabling them to effectively capture the local features and time-frequency information of signals.
The mathematical expression for wavelet transform is:
```
W(a, b) = \int_{-\infty}^{\infty} f(t) \psi_{a, b}(t) dt
```
Where:
* `W(a, b)` is the wavelet transform coefficient, representing the correlation of the signal `f(t)` with the wavelet function `\psi_{a, b}(t)` at scale `a` and translation `b`.
* `a` is the scale parameter, controlling the width and frequency of the wavelet function.
* `b` is the translation parameter, controlling the position of the wavelet function on the time axis.
# 2. Multiscale Transform Theory
### 2.1 Wavelet Transform
#### 2.1.1 Basic Principles of Wavelet Transform
Wavelet transform is a time-frequency analysis tool that decomposes signals into a weighted sum of wavelet functions. The wavelet function is a localized, oscillating function with good time-frequency characteristics.
The mathematical definition of wavelet transform is:
```
WT(a, b) = ∫f(t)ψ(a, b - t)dt
```
Where:
* `WT(a, b)` is the wavelet transform coefficient.
* `f(t)` is the signal to be analyzed.
* `ψ(a, b)` is the wavelet function.
* `a` is the scale parameter, controlling the scaling of the wavelet function.
* `b` is the translation parameter, controlling the translation of the wavelet function on the time axis.
#### 2.1.2 Applications of Wavelet Transform
Wavelet transform has a wide range of applications in the field of image processing, including:
* Image denoising
* Image enhancement
* Image compression
* Image fusion
### 2.2 Laplacian Pyramid
#### 2.2.1 Principles of the Laplacian Pyramid
The Laplacian pyramid is a multiscale image representation method, which is constructed through Gaussian smoothing and difference operations on images.
The process of constructing the Laplacian pyramid is as follows:
1. Apply Gaussian smoothing to the image to obtain a low-frequency image.
2. Perform a difference operation on the low-frequency image to obtain a Laplacian image.
3. Use the Laplacian image as the next layer's low-frequency image, and repeat steps 1 and 2.
#### 2.2.2 Applications of the Laplacian Pyramid
The Laplacian pyramid has a wide range of applications in the field of image processing, including:
* Image fusion
* Image edge detection
* Image texture analysis
* Image super-resolution
**Code Example:**
```python
import cv2
# Constructing a Laplacian pyramid
def build_laplacian_pyramid(image):
pyramid = []
for i in range(5):
image = cv2.pyrDown(image)
laplacian = cv2.Laplacian(image, cv2.CV_64F)
pyramid.append(laplacian)
return pyramid
# Reconstructing the image
def reconstruct_image(pyramid):
image = pyramid[-1]
for i in range(len(pyramid) - 2, -1, -1):
image = cv2.pyrUp(image)
image = image + pyramid[i]
return image
# Application
image = cv2.imread('image.jpg')
pyramid = build_laplacian_pyramid(image)
reconstructed_image = reconstruct_image(pyramid)
cv2.imshow('Reconstructed Image', reconstructed_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**Logical Analysis:**
* `build_laplacian_pyramid()` function constructs the Laplacian pyramid through iterative Gaussian smoothing and differentiation operations.
* `reconstruct_image()` function reconstructs the original image through iterative image upsampling and weighted summation.
* `imshow()` function displays the reconstructed image.
**Parameter Explanation:**
* `image`: Input image
* `pyramid`: Laplacian pyramid
* `reconstructed_image`: Reconstructed image
# 3.1 Application of Wavelet Transform in Image Fusion
#### 3.1.1 Wavelet Transform-Based Image Fusion Algorithm
Wavelet transform is mainly applied in image fusion for multiscale decomposition and reconstruction. Multiscale decomposition breaks the image down into subbands at different scales, each containing information within a different frequency range. By fusing these subbands, the fusion of different image features can be achieved.
The steps of the wavelet transform-based image fusion algorithm are as follows:
1. **Image Decomposition:** Decompose the source image using wavelet transform into subbands at different scales.
2. **Subband Fusion:** Fuse each subband using specific fusion rules. Fusion rules can include average, weighted average, maximum, minimum, etc.
3. **Image Reconstruction:** Reconstruct the fused subbands into a fused image using inverse wavelet transform.
#### 3.1.2 Examples of Wavelet Transform-Based Image Fusion
The following table presents specific examples of wavelet transform-based image fusion algorithms:
| Algorithm | Fusion Rule | Applicable Scenarios |
|---|---|---|
| Average Fusion | Arithmetic average of subbands | Suitable for fusing images under different lighting conditions |
| Weighted Average Fusion | Weighted average of subbands, weights determined by the importance of subbands | Suitable for fusing images with different resolutions |
| Maximum Value Fusion | Select the maximum value from each subband | Suitable for fusing images with rich texture features |
| Minimum Value Fusion | Select the minimum value from each subband | Suitable for fusing images with high
0
0
相关推荐







