【Advanced】Image Dehazing in MATLAB: Application of the Dark Channel Prior Method in Image Dehazing
发布时间: 2024-09-15 03:04:24 阅读量: 7 订阅数: 41 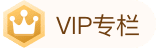
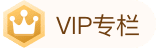
# 2.1 Dark Channel Prior Theory Foundation
### 2.1.1 Definition of Dark Channel Prior
The Dark Channel Prior (DCP) is a foundational theory for an image dehazing algorithm. It posits that in natural images, most non-sky regions contain small pixel values within their local patches. This pixel value is referred to as the dark channel, representing the pixels in the image that are least affected by haze.
### 2.1.2 Mathematical Model of Dark Channel Prior
The mathematical model of the dark channel prior can be expressed as:
```
J_d(x) = min_{y \in \Omega(x)}[I(y)]
```
Where:
* `J_d(x)` is the dark channel value at pixel `x`
* `I(y)` is the original image value at pixel `y`
* `Ω(x)` is the local patch of pixel `x`
# 2. The Principle and Algorithm of Dark Channel Prior Method
### 2.1 Dark Channel Prior Theory Foundation
#### 2.1.1 Definition of Dark Channel Prior
The Dark Channel Prior (DCP) is an image dehazing algorithm based on the atmospheric scattering model. The core assumption is that in most natural images, the minimum value channel (the dark channel) of local image patches is usually close to zero. This is because atmospheric scattering primarily affects the luminance component of the image, without significantly altering its color components.
#### 2.1.2 Mathematical Model of Dark Channel Prior
The mathematical model of the dark channel prior can be represented as:
```
J^d(x) = min_\{y \in \Omega(x)}[min_\{c \in \{r, g, b\}} I^c(y)]
```
Where:
* `J^d(x)` represents the dark channel of the image `I` at point `x`
* `\Omega(x)` represents the local image patch centered at `x`
* `I^c(y)` represents the value of the `c` channel of image `I` at point `y` (`c` being `r`, `g`, or `b`)
### 2.2 Dark Channel Prior Algorithm Process
The dark channel prior algorithm process mainly includes the following steps:
#### 2.2.1 Image Preprocessing
First, convert the original image to grayscale and perform normalization to eliminate the effects of lighting variation.
#### 2.2.2 Dark Channel Map Calculation
Based on the dark channel prior, calculate the dark channel map of the image:
```python
import cv2
import numpy as np
def dark_channel(image, window_size=7):
"""Calculate the dark channel map of the image.
Args:
image: Input image.
window_size: Local window size.
Returns:
Dark channel map.
"""
# Convert to grayscale image
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Normalize
gray_image = gray_image.astype(np.float32) / 255.0
# Calculate dark channel map
dark_channel = cv2.erode(gray_image, np.ones((window_size, window_size)))
return dark_channel
```
#### 2.2.3 Atmospheric Light Estimation
Atmospheric light is the strongest scattered light in the haze, which can be estimated through the global maximum value of the dark channel map:
```python
def estimate_airlight(dark_channel, percentile=0.999):
"""Estimate atmospheric light.
Args:
dark_channel: Dark channel map.
percentile: Percentile for atmospheric light estimation.
Returns:
Estimated atmospheric light.
"""
# Calculate the global maximum value of the dark channel map
max_value = np.max(dark_channel)
# Estimate atmospheric light based on percentile
airlight = np.percentile(dark_channel, percentile * 100)
return airlight
```
#### 2.2.4 Image Restoration
Finally, restore the hazy image based on the atmospheric light and dark channel map:
```python
def dehaze(image, dark_channel, airlight, omega=0.95):
"""Image dehazing.
Args:
image: Input hazy image.
dark_channel: ***
**rlight: Atmospheric light.
omega: Dehazing coefficient.
Returns:
Dehazed image.
"""
# Convert to grayscale image
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Normalize
gray_image = gray_image.astype(np.float32) / 255.0
# Image restoration
dehazed_image = (gray_image - omega * dark_channel) / (1 - omega * dark_channel / airlight)
# Denormalize
dehazed_image = np.clip(dehazed_image * 255.0, 0, 255).astype(np.uint8)
return dehazed_image
```
# 3. Application of Dark Channel Prior Method in Image Dehazing
### 3.1 Evaluation of Image Dehazing Effect
#### 3.1.1 Dehazing Effect Evaluation Indicators
The i
0
0
相关推荐
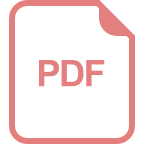
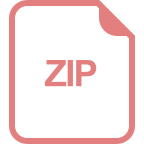
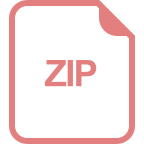





