【Fundamental】Geometric Correction and Registration of MATLAB Images
发布时间: 2024-09-15 02:53:57 阅读量: 36 订阅数: 53 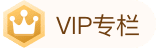
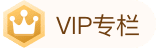
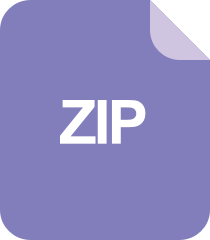
Theory and Applications of Image Registration
# 1. Basic Concepts of MATLAB Image Geometric Correction
Image geometric correction is a vital image processing technique used to rectify geometric distortions in images caused by factors such as camera distortion, motion blur, or others. MATLAB offers a wealth of functions and toolboxes that facilitate image geometric correction with ease.
Geometric correction involves various transformations of images, including translation, rotation, scaling, and affine transformations. These transformations can be achieved by applying appropriate transformation matrices, which define the relationship between the original coordinates and the transformed coordinates for each pixel in the image.
In MATLAB, the imtranslate function can be used for translation transformations, imrotate for rotation transformations, imresize for scaling transformations, and imcrop for cropping transformations. These functions provide flexible parameter settings, allowing users to precisely control the transformation parameters.
# 2. Image Geometric Correction Algorithms
Image geometric correction algorithms aim to rectify geometric distortions present in an image, such as translation, rotation, scaling, and cropping. These distortions may result from camera movement, lens distortion, or other factors. By applying appropriate geometric correction algorithms, the original geometric shape of an image can be restored.
### 2.1 Image Translation and Rotation
#### 2.1.1 Translation Transformation Matrix
A translation transformation moves the image along the x-axis or y-axis by a specified distance. The translation transformation matrix is as follows:
```
T = [1 0 tx;
0 1 ty;
0 0 1]
```
Where:
- `tx` and `ty` are the translation distances along the x-axis and y-axis, respectively.
**Code Block:**
```
% Image Translation
I = imread('image.jpg');
tx = 50; % Translate 50 pixels along the x-axis
ty = -20; % Translate -20 pixels along the y-axis
T = [1 0 tx;
0 1 ty;
0 0 1];
I_translated = imwarp(I, T, 'OutputView', 'same');
imshow(I_translated);
```
**Logical Analysis:**
This code translates the image 50 pixels along the x-axis and -20 pixels along the y-axis. The `imwarp` function uses the translation transformation matrix `T` to translate the image.
#### 2.1.2 Rotation Transformation Matrix
A rotation transformation rotates the image around its center by a specified angle. The rotation transformation matrix is as follows:
```
R = [cos(theta) -sin(theta) 0;
sin(theta) cos(theta) 0;
0 0 1]
```
Where:
- `theta` is the rotation angle in radians.
**Code Block:**
```
% Image Rotation
I = imread('image.jpg');
theta = pi/6; % Rotate by 30 degrees
R = [cos(theta) -sin(theta) 0;
sin(theta) cos(theta) 0;
0 0 1];
I_rotated = imwarp(I, R, 'OutputView', 'same');
imshow(I_rotated);
```
**Logical Analysis:**
This code rotates the image 30 degrees around its center. The `imwarp` function uses the rotation transformation matrix `R` to rotate the image.
### 2.2 Image Scaling and Cropping
#### 2.2.1 Scaling Transformation Matrix
Scaling transformation resizes the image by a specified ratio. The scaling transformation matrix is as follows:
```
S = [sx 0 0;
0 sy 0;
0 0 1]
```
Where:
- `sx` and `sy` are the scaling factors along the x-axis and y-axis, respectively.
**Code Block:**
```
% Image Scaling
I = imread('image.jpg');
sx = 0.5; % Scale 50% along the x-axis
sy = 1.2; % Scale 120% along the y-axis
S = [sx 0 0;
0 sy 0;
0 0 1];
I_scaled = imwarp(I, S, 'OutputView', 'same');
imshow(I_scaled);
```
**Logical Analysis:**
This code scales the image 50% along the x-axis and 120% along the y-axis. The `imwarp` function uses the scaling transformation matrix `S` to scale the image.
#### 2.2.2 Cropping Operation
The cropping operation removes a specified region from the image. The cropping operation can be performed using the `imcrop` function.
**Code Block:**
```
% Image Cropping
I = imread('image.jpg');
rect = [x1 y1 width height]; % Cropping area
I_cropped = imcrop(I, rect);
imshow(I_cropped);
```
**Logical Analysis:**
This code crops a specified area from the image, defined by the `rect` parameter. The `imcrop` function returns the cropped image.
### 2.3 Image Affine Transformation
#### 2.3.1 Affine T
0
0
相关推荐







