【Basic】Image Segmentation in MATLAB: Using K-means Clustering for Image Segmentation
发布时间: 2024-09-15 02:32:53 阅读量: 32 订阅数: 42 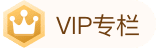
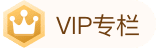
## 2.1 K-Means Clustering Algorithm Principle
The K-means clustering algorithm is an unsupervised machine learning technique that partitions data points into K clusters. The principle of the algorithm is as follows:
***Initialization:** Randomly select K data points as the initial cluster centers.
***Assignment:** Assign each data point to the cluster center closest to it.
***Update:** Calculate the average of all data points in each cluster and use it as the new cluster center.
***Repeat:** Repeat the assignment and update steps until the cluster centers no longer change or a predefined number of iterations is reached.
Ultimately, the algorithm divides the data points into K clusters, with each cluster consisting of data points closest to its center.
## 2. K-Means Clustering Algorithm
### 2.1 K-Means Clustering Algorithm Principle
The K-means clustering algorithm is an unsupervised learning technique used to partition data points into K clusters. The goal is to find a set of cluster centers such that the sum of distances from each data point to its nearest cluster center is minimized.
**Algorithm Principle:**
1. **Initialization:** Randomly select K data points as the initial cluster centers.
2. **Assignment:** Assign each data point to the nearest cluster center.
3. **Update:** Recalculate the center of each cluster as the average of all data points within it.
4. **Repeat:** Repeat steps 2 and 3 until the cluster centers no longer change or the maximum number of iterations is reached.
### 2.2 K-Means Clustering Algorithm Steps
**Steps:**
1. **Determine the K value:** Decide the number of clusters to divide into.
2. **Initialize cluster centers:** Randomly select K data points as the initial cluster centers.
3. **Calculate distances:** Compute the distance from each data point to each cluster center.
4. **Assign clusters:** Assign each data point to the nearest cluster center.
5. **Update cluster centers:** Recalculate the center of each cluster as the average of all its data points.
6. **Repeat:** Repeat steps 3-5 until the cluster centers no longer change or the maximum number of iterations is reached.
**Code Block:**
```python
import numpy as np
import matplotlib.pyplot as plt
# Data points
data = np.array([[1, 2], [3, 4], [5, 6], [7, 8], [9, 10]])
# K value
K = 2
# Initialize cluster centers
centroids = np.array([[1, 2], [9, 10]])
# Distance calculation function
def distance(x, y):
return np.linalg.norm(x - y)
# Cluster assignment function
def assign_cluster(data, centroids):
clusters = np.zeros(len(data))
for i in range(len(data)):
distances = [distance(data[i], centroid) for centroid in centroids]
clusters[i] = np.argmin(distances)
return clusters
# Cluster center update function
def update_centroids(data, clusters, K):
new_centroids = np.zeros((K, 2))
for i in range(K):
cluster_data = data[clusters == i]
new_centroids[i] = np.mean(cluster_data, axis=0)
return new_centroids
# Maximum iterations
max_iterations = 10
# Iterate
for i in range(max_iterations):
clusters = assign_cluster(data, centroids)
centroids = update_centroids(data, clusters, K)
# Visualization
plt.scatter(data[:, 0], data[:, 1], c=clusters)
plt.scatter(centroids[:, 0], centroids[:, 1], c='r', marker='x')
plt.show()
```
**Code Logic Analysis:**
***Initialization:** Randomly initialize K cluster centers.
***Distance calculation:** Calculate the Euclidean distance from each data point to each cluster center.
***Cluster assignment:** Assign each data point to the
0
0