【Basic】Morphological Operations in MATLAB: Implementation of Erosion and Dilation
发布时间: 2024-09-15 02:25:26 阅读量: 43 订阅数: 50 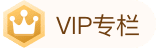
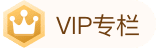
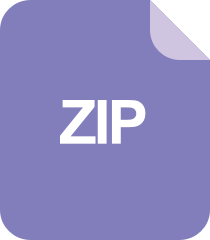
Morphological-Operations:承担
# 2.1 Fundamental Concepts of Morphology
Morphology is a powerful tool in image processing used to analyze the shapes and structures of images. It is based on a set of basic operations called morphological operators, which can modify the size, shape, and topological structure of objects within an image.
The fundamental concepts of morphology include:
- **Structuring Element (SE):** A small binary image used to perform morphological operations within an image. The shape and size of the SE determine the effect of the operation.
- **Erosion:** An operation that uses the SE to shrink objects within an image.
- **Dilation:** An operation that uses the SE to enlarge objects within an image.
- **Opening:** Erosion followed by dilation.
- **Closing:** Dilation followed by erosion.
# 2. Theoretical Foundation of Morphological Operations
### 2.1 Fundamental Concepts of Morphology
Morphology is an important technique in image processing that extracts useful information by analyzing the shapes and structures of objects in an image. Morphological operations are based on concepts from set theory and topology, considering an image as a set composed of a group of pixels.
The fundamental concepts of morphology include:
***Structuring Element (SE):** A small, fixed-shape template used for convolution operations with an image.
***Erosion:** Each pixel in the image is compared with the structuring element; if the structuring element is completely contained within the pixel's neighborhood, the pixel is retained, otherwise, it is removed.
***Dilation:** Each pixel in the image is compared with the structuring element; if the structuring element overlaps with any part of the pixel's neighborhood, the pixel is retained, otherwise, it is removed.
### 2.2 Definition and Properties of Erosion and Dilation
**Erosion**
The definition of the erosion operation is as follows:
```
Erosion(A, B) = {z | B_z ⊆ A}
```
Where:
* A is the input image
* B is the structuring element
* B_z is the translation of B at point z
* ⊆ denotes a subset
The erosion operation has the following properties:
***Reduces objects:** Erosion removes small objects and fine lines from the image.
***Smooths boundaries:** Erosion smooths the boundaries in the image, removing spikes and noise.
***Maintains topological structure:** Erosion does not change the topological structure of the image, such as connectivity.
**Dilation**
The definition of the dilation operation is as follows:
```
Dilation(A, B) = {z | B_z ∩ A ≠ ∅}
```
Where:
* A is the input image
* B is the structuring element
* B_z is the translation of B at point z
* ∩ denotes intersection
* ≠ denotes not equal to
The dilation operation has the following properties:
***Enlarges objects:** Dilation enlarges objects and fine lines in the image.
***Fills holes:** Dilation fills holes and gaps in the image.
***Maintains topological structure:** Dilation does not change the topological structure of the image, such as connectivity.
**Complementarity of erosion and dilation**
Erosion and dilation are complementary operations that can be combined to achieve various image processing tasks. For example, erosion can remove noise from an image, while dilation can fill in holes within an image.
# 3.1 Implementation of Erosion Operation
### Definition of Erosion Operation
The erosion operation is a morphological process that removes small objects from an image by using a kernel known as a structuring element. The structuring element is typically a binary matrix with a central element of 1 and all other elements being 0.
### Implementation of Erosion Operation in MATLAB
In MATLAB, the erosion operation can be performed using the `imerode` function. The syntax for this function is as follows:
```
BW2 = imerode(BW, SE)
```
Where:
* `BW` is the input binary image.
* `SE` is the structuring element.
* `BW2` is the output image after erosion.
### Logical Analysis of Erosion Operation
The logic of the erosion operation is as follows:
***
***pare all elements of the structuring element with the corresponding pixels in the input image.
3. If all elements of the structuring element match the corresponding pixels in the input image, set the corresponding pixel in the output image to 1.
4. Otherwise, set the corresponding pixel in the output image to 0.
### Parameter Description of `imerode` Function
The parameters for the `imerode` fu
0
0
相关推荐
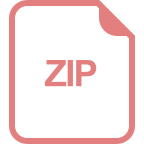
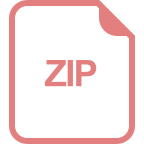
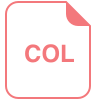
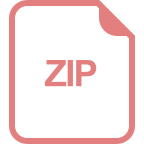
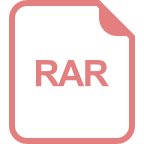
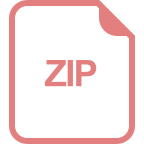
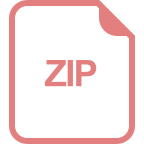
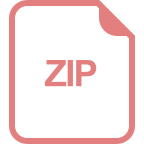
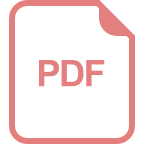