Comprehensive Explanation of MATLAB Program File Types
发布时间: 2024-09-13 15:42:41 阅读量: 23 订阅数: 34 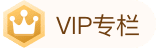
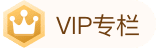
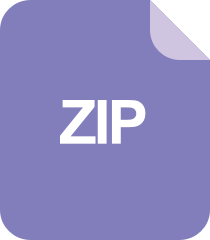
果壳处理器研究小组(Topic基于RISCV64果核处理器的卷积神经网络加速器研究)详细文档+全部资料+优秀项目+源码.zip
# Understanding MATLAB Program File Types
## 1. Script Files (.m)
### 1.1 Structure and Execution of Script Files
#### 1.1.1 Components of Script Files
A MATLAB script file (.m extension) is a text file that contains a series of MATLAB commands. A script file consists of the following parts:
- **Function Declaration (Optional):** Declares the main function within the file, typically starting with the `function` keyword.
- **Variable Definitions and Assignments:** Defines variables and assigns values to them.
- **Commands:** Executes MATLAB commands such as calculations, data processing, and graph plotting.
- **Comments (Optional):** Lines starting with the `%` symbol, used to provide explanations and notes for the code.
#### 1.1.2 Execution Process of Script Files
The MATLAB interpreter executes the commands in the script file line by line. When it encounters a function declaration, it defines the function and stores it in memory. When it encounters variable definitions and assignments, it creates variables and stores their values in memory. When it encounters commands, it executes them and produces results.
## 2. Function Files (.m)
### 2.1 Structure and Calling of Function Files
#### 2.1.1 Components of Function Files
Function files with a `.m` extension are composed of the following parts:
- **Function Header:** Specifies the function name, input parameters, and output parameters.
- **Function Body:** Contains the code of the function, which performs specific tasks.
- **Comments:** Provides documentation about the function's functionality, usage, and parameters.
```
% Function Header
function [output1, output2] = myFunction(input1, input2)
% Function Body
% Code...
% Comments
% Describes the function's purpose and usage
```
#### 2.1.2 Calling Method of Function Files
To call a function file, use the following syntax:
```
[output1, output2] = myFunction(input1, input2);
```
Where:
- `myFunction` is the function name.
- `input1` and `input2` are the input parameters.
- `output1` and `output2` are the output parameters (optional).
### 2.2 Parameter Passing in Function Files
#### 2.2.1 Input Parameters and Output Parameters
Functions can accept input parameters and return output parameters. Input parameters are used to provide data to the function, while output parameters are used to retrieve results from the function.
#### 2.2.2 Method of Parameter Passing
Parameter passing in MATLAB is by value, meaning that the values of variables passed to the function are copied into the function. The function operates on these copies without affecting the original variables.
#### 2.2.3 Example of Parameter Passing
The following code example demonstrates parameter passing within a function file:
```
% Function Header
function [sum, product] = calculate(num1, num2)
% Function Body
sum = num1 + num2;
product = num1 * num2;
% Comments
% Calculates the sum and product of two numbers
```
To call this function, use the following syntax:
```
[sum, product] = calculate(10, 5);
```
Here, the
0
0
相关推荐
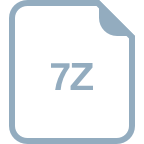
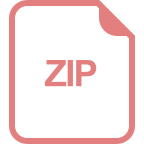
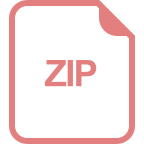
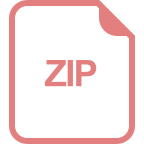
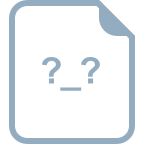
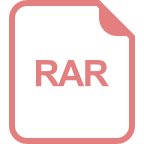
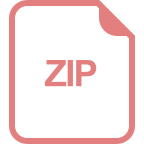