【Advanced Edition】MATLAB Database Toolbox: Database Toolbox User Guide
发布时间: 2024-09-13 16:20:52 阅读量: 32 订阅数: 43 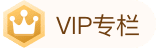
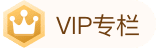
# [Advanced Guide] MATLAB Database Toolbox: A Guide to Database Toolbox Usage
## 1. Introduction to MATLAB Database Toolbox
MATLAB Database Toolbox is a toolkit designed for accessing and manipulating relational databases. It offers a suite of functions that enable MATLAB users to connect to a variety of databases, execute SQL queries, manage data, and perform data analysis. The Database Toolbox is widely used in fields such as data science, machine learning, and financial modeling. It allows MATLAB users to interact with relational databases, thereby extending MATLAB's capabilities to become a more powerful platform for data analysis and processing.
## 2. Database Connection and Operations
### 2.1 Connecting to a Database
The MATLAB Database Toolbox provides multiple methods for connecting to databases, including ODBC, JDBC, and ADO. The basic steps for connecting to a database are as follows:
1. Create a database connection object:
```matlab
conn = database('my_database', 'my_username', 'my_password', 'my_driver');
```
| Parameter | Description |
|---|---|
| `my_database` | Database name |
| `my_username` | Database username |
| `my_password` | Database password |
| `my_driver` | Database driver (e.g., 'ODBC Driver 17 for SQL Server') |
2. Open the connection:
```matlab
open(conn);
```
3. Check the connection status:
```matlab
isopen(conn)
```
### 2.2 Executing SQL Queries
After connecting to the database, you can execute SQL queries using the `exec` function. The syntax for the `exec` function is as follows:
```matlab
result = exec(conn, 'sql_query');
```
| Parameter | Description |
|---|---|
| `conn` | Database connection object |
| `sql_query` | SQL query to be executed |
| `result` | Query result |
The query result is a structure that contains the following fields:
| Field | Description |
|---|---|
| `Data` | Query result data |
| `Fields` | Query result field names |
| `RowCount` | Number of rows in the query result |
### 2.3 Handling Query Results
Query results can be accessed through the `Data` field. The `Data` field is a cell array where each element corresponds to a row in the query result. For instance, to retrieve the data from the first row and first column of the query result, you can use the following code:
```matlab
first_row_first_column = result.Data{1, 1};
```
You can also use the `Fields` field to get the field names of the query result. The `Fields` field is a string array where each element corresponds to a column in the query result. For example, to retrieve the field name of the first column, you can use the following code:
```matlab
first_column_name = result.Fields{1};
```
If the query result contains no data, the `Data` field will be empty, and the `RowCount` field will be 0.
## 3. Data Management
### 3.1 Creating and Dropping Tables
**Creating a Table**
```matlab
% Create a table named "customers"
CREATE TABLE customers (
id INTEGER PRIMARY KEY,
name VARCHAR(255),
email VARCHAR(255),
phone VARCHAR(255)
);
```
**Parameter Explanation:**
* `INTEGER PRIMARY KEY`: Specifies the `id` column as the primary key and enforces it to be an integer type.
* `VARCHAR(255)`: Specifies the `name`, `email`, and `phone` columns as variable-length strings with a maximum length of 255 characters.
**Dropping a Table**
```matlab
% Drop the table named "customers"
DROP TABLE customers;
```
### 3.2 Inserting, Updating, and Deleting Data
**Inserting Data**
```matlab
% Insert a record into the "customers" table
INSERT INTO customers (name, email, phone)
VALUES ('John Doe', 'john.***',
```
0
0
相关推荐








