【Basic】Detailed Explanation of MATLAB Matrix Operations
发布时间: 2024-09-13 15:48:23 阅读量: 41 订阅数: 35 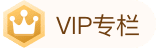
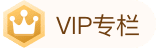
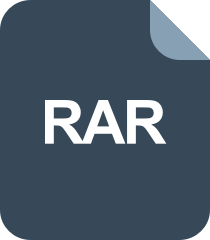
OSI model.rar_OSI Reference_OSI Reference Model_explanation of O
# **1. Basic MATLAB Matrix Operations**
Matrices in MATLAB are powerful data structures used for storing and manipulating numerical arrays. Matrix elements can be scalars (individual numbers), vectors (one-dimensional arrays), or other matrices. MATLAB provides a wide range of functions and operators for handling matrices, making it an ideal tool for scientific computing and data analysis.
Basic matrix operations include creating, accessing, and modifying elements, as well as performing arithmetic and logical operations. MATLAB uses square brackets ([]) to create matrices and commas (,) and semicolons (;) to separate rows and columns. For example, the following code creates a 3x3 matrix:
```
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
```
Matrix elements can be accessed using indexing, starting from 1. For example, the following code accesses the element in the second row, third column of matrix A:
```
A(2, 3)
```
# **2. Theoretical Background of MATLAB Matrix Operations**
### **2.1 Matrix Addition, Subtraction, Multiplication, and Division**
#### **2.1.1 Matrix Addition and Subtraction**
Matrix addition and subtraction are similar to scalar addition and subtraction. For two matrices **A** and **B** of the same size, their addition and subtraction are defined as:
```
C = A + B
C = A - B
```
Where, **C** is the result matrix, and its element **C(i, j)** is the sum or difference of **A(i, j)** and **B(i, j)**.
**Code Block:**
```matlab
% Define matrices A and B
A = [1 2 3; 4 5 6; 7 8 9];
B = [10 11 12; 13 14 15; 16 17 18];
% Matrix addition
C = A + B;
% Matrix subtraction
D = A - B;
% Output results
disp('Matrix addition result:')
disp(C)
disp('Matrix subtraction result:')
disp(D)
```
**Logical Analysis:**
* Define matrices **A** and **B**.
* Use `+` and `-` operators to perform matrix addition and subtraction.
* The result matrices **C** and **D** contain elements that are the sum or difference of corresponding elements in **A** and **B**.
#### **2.1.2 Matrix Multiplication**
Matrix multiplication differs from scalar multiplication in that it involves multiplying elements row by column and summing the products. For two matrices **A** and **B**, their multiplication is defined as:
```
C = A * B
```
Where, **C** is the result matrix, and its element **C(i, j)** is the inner product of the **i**-th row of **A** and the **j**-th column of **B**.
**Code Block:**
```matlab
% Define matrices A and B
A = [1 2 3; 4 5 6];
B = [7 8; 9 10; 11 12];
% Matrix multiplication
C = A * B;
% Output results
disp('Matrix multiplication result:')
disp(C)
```
**Logical Analysis:**
* Define matrices **A** and **B**.
* Use `*` operator to perform matrix multiplication.
* The result matrix **C** contains elements that are the inner product of the row vector of **A** and the column vector of **B**.
#### **2.1.3 Matrix Division**
Matrix division, unlike scalar division, involves the inverse of the matrix. For a matrix **A**, its division is defined as:
```
X = A \ B
```
Where, **X** is the result matrix, satisfying **A * X = B**.
**Code Block:**
```matlab
% Define matrices A and B
A = [2 1; 3 4];
B = [5; 6];
% Matrix division
X = A \ B;
% Output results
disp('Matrix division result:')
disp(X)
```
**Logical Analysis:**
* Define matrices **A** and **B**.
* Use `\` operator to perform matrix division.
* The result matrix **X** satisfies the equation **A * X = B**.
### **2.2 Matrix Determinant and Inverse**
#### **2.2.1 Calculation of Matrix Determinant**
The determinant of a matrix is a scalar value that describes the relationship between the rows and columns of the matrix. For an **n x n** matrix **A**, its determinant is defined as:
```
det(A) = ∑(i=1 to n) a(i, j) * C(i, j)
```
Where, **a(i, j)** is the element in the **i**-th row and **j**-th column of **A**, and **C(i, j)** is the algebraic cofactor of the element in the **i**-th row and **j**-th column of **A**.
**Code Block:**
```matlab
% Define matrix A
A = [2 1; 3 4];
% Calculate determinant
detA = det(A);
% Output results
disp('Determinant of matrix A:')
disp(detA)
```
**Logical Analysis:**
* Define matrix **A**.
* Use the `det` function to calculate the determinant of the matrix.
* The result is the determinant of matrix **A**.
#### **2.2.2 Calculation of Matrix Inverse**
The inverse of a matrix is a matrix that, when multiplied by the original matrix, yields the identity matrix. For a non-singular matrix **A**, its inverse is defined as:
```
A^-1 = 1 / det(A) * C^T
```
Where, **det(A)** is the determinant of **A**, and **C^T** is the transpose of the cofactor matrix of **A**.
**Code Block:**
```matlab
% Define matrix A
A = [2 1; 3 4];
% Calculate inverse matrix
invA = inv(A);
% Output results
disp('Inverse matrix of matrix A:')
disp(invA)
```
**Logical Analysis:**
* Define matrix **A**.
* Use the `inv` function to calculate t
0
0