[Advanced Chapter] Methods for Combining the Use of C and MATLAB (Mutual Invocation)
发布时间: 2024-09-13 16:57:19 阅读量: 26 订阅数: 43 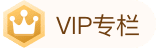
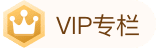
# Quick Start Guide to MATLAB Learning
C and MATLAB are two widely-used programming languages, each playing a significant role in system programming and scientific computing. C is renowned for its efficiency and flexibility, while MATLAB is acclaimed for its powerful numerical computation and data visualization capabilities. This chapter will introduce the foundational knowledge of both C and MATLAB, laying the groundwork for subsequent sections.
* A brief overview of C: syntax, data types, control flow, and functions.
* An introduction to MATLAB: variables, arrays, matrices, and fundamental operations.
* Similarities and differences between C and MATLAB.
# 2. Fundamentals of C and MATLAB Interaction
### 2.1 C Invoking MATLAB Functions
#### 2.1.1 Declaration and Invocation of MATLAB Functions
Invoking MATLAB functions in C requires the use of the MATLAB Engine API. Initially, one must declare the prototype of the MATLAB function, including the function name, parameter types, and the type of the return value. For example:
```c
#include "engine.h"
// MATLAB function prototype
int myMATLABFunction(int n, double *x, double *y);
```
Then, use the `engOpen` function to initialize the MATLAB Engine and `engEvalString` function to invoke MATLAB functions.
```c
// Open the MATLAB Engine
Engine *ep = engOpen(NULL);
// Invoke MATLAB functions
int n = 10;
double x[n], y[n];
engEvalString(ep, "x = linspace(0, 1, n);");
engEvalString(ep, "y = sin(x);");
myMATLABFunction(n, x, y);
// Close the MATLAB Engine
engClose(ep);
```
#### 2.1.2 Data Type Conversion
When passing data between C and MATLAB, data type conversion is necessary. MATLAB utilizes its own data type system, ***mon conversions include:
| MATLAB Data Type | C Data Type |
|---|---|
| double | double |
| int32 | int |
| char | char |
### 2.2 MATLAB Invoking C Functions
#### 2.2.1 Declaration and Export of C Functions
To allow MATLAB to invoke C functions, one must use MATLAB MEX (executable) files. MEX files contain the implementation of C functions and are compiled into executables using MATLAB's compiler.
The declaration of C functions should follow MATLAB MEX function specifications, including the function name, parameter types, and return value types. For example:
```c
#include "mex.h"
// C function declaration
void mexFunction(int nlhs, mxArray *plhs[], int nrhs, const mxArray *prhs[])
{
// Function implementation
}
```
Then, use the `mex` command to compile the C function, generating a MEX file.
```
mex myCFunction.c
```
#### 2.2.2 Function Parameter Passing
When MATLAB calls a C function, parameters are passed through the `mxArray` structure. The `mxArray` structure contains the type, dimensions, and actual data of the data.
```matlab
% Create a MATLAB array
x = [1, 2, 3];
% Convert the MATLAB array to an.mxArray
mxArray *mx = mxCreateDoubleMatrix(1, 3, mxREAL);
memcpy(mx->data, x, sizeof(double) * 3);
% Call the C function
myCFunction(1, &mx);
% Extract data from the.mxArray
double *y = (double *)mx->data;
```
# 3.1 Passing Array Data
#### 3.1.1 Types and Sizes of Arrays
In C and MATLAB interaction, passing array data involves two different data types: numeric arrays and structure arrays.
***Numeric Arrays:** Containing elements of the same data type, such as integers, floating points, or characters. MATLAB uses square brackets `[]` to denote numeric arrays, for example:
```
>> a = [1, 2, 3; 4, 5, 6]
a =
1 2 3
4 5 6
```
***Structure Arrays:** Containing elements of different data types, each element is called a structure. MATLAB uses the `struct` keyword to define structures, for example:
```
>> student = struct('name', {'John', 'Mary'}, 'age', {20, 21})
student =
struct with fields:
name: {'John', 'Mary'}
age: {20, 21}
```
C does not have a directly corresponding type to MATLAB structure arrays, so they must be converted into equivalent data structures in C.
#### 3.1.2 Data Copying and Memory Management
In C and MATLAB interaction, array data passing involves data copying and memory management.
***Data Copying:** When MATLAB arrays are passed to C functions, MATLAB copies the array data to the memory space of the C function. Similarly, when C arrays are passed to MATLAB functions, C copies the array data to MATLAB's memory space.
***Memory Management:** MATLAB is responsible for managing its memory space, while C is responsible for its own memory space. When MATLAB arrays are passed to C functions, MATLAB keeps a copy of the original.
0
0
相关推荐








