[Advanced Chapter] Detailed Explanation of GUI Design and Interactive Applications in MATLAB
发布时间: 2024-09-13 16:52:58 阅读量: 28 订阅数: 43 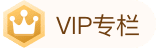
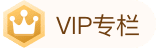
# **Advanced Section** GUI Design and Interactive Applications in MATLAB Explained
## 2.1.1 Concepts and Components of a Graphical User Interface (GUI)
A Graphical User Interface (GUI) is an interface that allows users to interact with computer programs through graphical elements such as buttons, menus, and text boxes. The main components of a GUI include:
- **Window:** A rectangular area containing GUI elements and content.
- **Controls:** Interactive elements such as buttons, text boxes, and check boxes that allow users to input and manipulate data.
- **Layout:** The arrangement of controls within a window, affecting the usability and aesthetics of the GUI.
- **Event Handling:** Actions triggered by user interactions with controls, such as clicking a button or entering text.
## 2. MATLAB GUI Programming in Practice
### 2.1 Creation and Layout of GUI Components
#### 2.1.1 Concepts and Components of a Graphical User Interface (GUI)
A GUI (Graphical User Interface) is a type of human-computer interaction interface that allows users to interact with computer programs through graphical elements such as buttons, text boxes, menus, etc. A MATLAB GUI consists of a series of components including:
- **Controls:** Elements for user input and interaction, such as buttons, text boxes, check boxes, etc.
- **Containers:** Elements for organizing and laying out controls, such as panels, tabs, etc.
- **Menu:** A list that provides application functions and options.
- **Toolbar:** Shortcuts for common commands and functions.
- **Status Bar:** Displays application status and messages.
#### 2.1.2 Types and Uses of Common GUI Components
MATLAB offers a rich set of GUI components, each with specific uses:
| Component Type | Usage |
|---|---|
| Button | Triggers events or performs actions |
| Text Box | Inputs and displays text |
| Check Box | Allows users to select or deselect options |
| Radio Button | Allows users to choose one option from a set |
| Drop-down List | Allows users to select a value from a predefined list |
| List Box | Allows users to select multiple values from a list |
| Table | Displays and edits data |
| Chart | Visualizes data |
| Axis | Plots graphics |
#### 2.1.3 Principles and Techniques of GUI Layout
GUI layout is crucial for user experience. Here are some principles and techniques for GUI layout:
- **Consistency:** Maintain consistent layout and appearance of controls and containers throughout the application.
- **Hierarchy:** Use containers to organize controls, forming a clear hierarchy.
- **Alignment:** Align controls to create visual balance and order.
- **Spacing:** Leave sufficient spacing between controls to enhance readability and accessibility.
- **Grouping:** Group related controls together to improve user understanding.
### 2.2 GUI Event Handling and Interaction
#### 2.2.1 Event Handling Mechanism and Types
Event handling is the core of GUI programming. Events are actions generated by the user or the system, such as clicking a button or moving the mouse. MATLAB uses an event listener mechanism to handle events.
MATLAB supports the following event types:
- **Mouse Events:** Click, double-click, move, etc.
- **Keyboard Events:** Key press, key release, etc.
- **Control Events:** Control-specific events, such as button clicks, text box changes, etc.
- **Timer Events:** Events triggered at regular intervals.
#### 2.2.2 Adding and Removing Event Listeners
To handle events, event listeners need to be added to controls. The following code can be used to add a listener:
```matlab
button = uibutton('Text', 'Click Me');
addlistener(button, 'ButtonPushed', @buttonPushedCallback);
function buttonPushedCallback(~, ~)
disp('Button was pushed!');
end
```
To remove a listener, the following code can be used:
```matlab
removelistener(button, 'ButtonPushed', buttonPushedCallback);
```
#### 2.2.3 Implementation of an Interactive GUI
Through event handling, an interactive GUI can be created. For example, the following code creates a button that updates the text in a text box when clicked:
```matlab
button = uibutton('Text', 'Click Me');
textBox = uieditfield('Value', 'Initial Text');
addlistener(button, 'ButtonPushed', @buttonPushedCallback);
function buttonPushedCallback(~, ~)
textBox.Value = 'Updated Text';
end
```
### 2.3 GUI Data Binding and Dynamic Updates
#### 2.3.1 Concepts and Advantages of Data Binding
Data binding is a technique that connects GUI controls to data sources, such as variables or databases. When data in the source changes, GUI controls will automatically update, and vice versa.
The advantages of data binding include:
- **Simplified Code:** No need for manual updates to control values.
- **Improved Efficiency:** Changes in the data source are automatically reflected in the GUI.
- **Enhanced User Experience:** Users can see data changes in real-time.
#### 2.3.2 Types and Implementation of Data Binding
MATLAB supports two types of data binding:
- **One-way Data Binding:** Changes in the data source are reflected in GUI controls, but changes in controls are not reflected in the data source.
- **Two-way Data Binding:** Changes in both the data source and GUI controls are mutually reflected.
The following code can be used to implement one-way data binding:
```matlab
variable = 10;
textBox = uieditfield('Value', variable);
% Bind variable and text box
bindprop(textBox, 'Value', variable);
```
The following code can be used to implement two-way data binding:
```matlab
variable = 10;
textBox = uieditfield('Value', variable);
% Bind variable and text box with two-way binding specified
bindprop(textBox, 'Value', variable, 'Direction', 'both');
```
#### 2.3.3 Techniques for Dynamically Updating the GUI Interface
Data binding can be combined with other techniques to dynamically update the GUI interface. For example, a timer can be used to periodically update the data source, thereby triggering updates to GUI controls.
The following code creates a timer that updates the text in a text box every second:
```matlab
timer = timer('Period', 1, 'ExecutionMode', 'fixedRate');
textBox = uieditfield('Value', 'Initial Text');
addlistener(timer, 'TimerFcn', @timerCallback);
start(timer);
function timerCallback(~, ~)
textBox.Value = ['Updated Text: ' num2str(rand)];
end
```
# 3.1 Development of Custom GUI Components
#### 3.1.1 Creation and Use of Custom Components
Custom GUI components allow you to create unique components that meet specific needs, which may not be achievable with standard MATLAB components. To create a custom component, follow these steps:
1. **Create a Class ***'s properties, methods, and events.
2. **Define Properties:** Use the `properties` keyword to define the component's properties. Properties are values representing the component's state or data.
3. **Define Methods:** Use the `methods` keyword to define the component's methods. Methods are functions that perform speci
0
0
相关推荐








