Detailed Explanation of Procedural Statements and Branching Structures in Verilog
发布时间: 2024-09-14 03:15:20 阅读量: 36 订阅数: 28 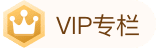
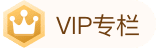
# Introduction to Verilog
Verilog is a Hardware Description Language (HDL) used for modeling, simulating, and synthesizing digital circuits. Within the realm of digital circuit design, Verilog is extensively used in various fields including integrated circuit design, FPGA programming, and digital signal processing.
## Brief Introduction to Verilog
Developed by Gateway Design Automation in 1984, Verilog was later acquired and promoted by Cadence Design Systems. It is an event-driven language that can describe the behavior, structure, and timing characteristics of digital systems.
## Applications of Verilog
Verilog is widely applied in the field of digital circuit design, including but not limited to:
- ASIC Design: Used for the design and verification of custom integrated circuits.
- FPGA Programming: Utilized to configure FPGA chips to perform specific functions.
- Digital Signal Processing: Employed to describe and verify various digital signal processing algorithms and circuits.
## Advantages and Characteristics of Verilog
The advantages and characteristics of Verilog include:
- High abstraction: Allows for the description of complex digital circuit behaviors.
- Easy to learn: Syntax similar to C language, making it easy to grasp and utilize.
- Maintainability: Modular design style facilitates organization and maintenance of code.
In the following chapters, we will delve into the basic concepts, procedural statements, and branch structures in Verilog, as well as how to apply Verilog for digital circuit design.
# Basic Concepts in Verilog
As a hardware description language, Verilog plays a crucial role in digital circuit design. Understanding the basic concepts in Verilog is essential for mastering Verilog programming. This chapter will introduce modules, ports, signals, data types, and concepts related to sequential and combinational logic in Verilog.
### Modules and Ports
In Verilog, a module is an independent functional unit that may contain combinational logic, sequential logic, etc. Modules communicate with other modules or the external environment through ports. Ports are categorized into input ports (input), output ports (output), bidirectional ports (inout), etc.
```verilog
module my_module (
input wire A, // Input port A
input wire B, // Input port B
output reg Y // Output port Y
);
always @(*) begin
Y = A & B; // Implementing AND gate logic
end
endmodule
```
### ***
***mon data types include wire, reg, integer, real, etc. Wire is used for signals with continuous assignments, while reg is used for registers in sequential logic.
```verilog
module data_flow (
input wire clk, // Clock signal
input wire [3:0] data_input, // 4-bit input data
output reg [3:0] data_output // 4-bit output data
);
always @(posedge clk) begin
data_output <= data_input; // Output input data on the clock rising edge
end
endmodule
```
### Sequential and Combinational Logic
In Verilog, logic can be divided into sequential logic and combinational logic. Sequential logic is logic controlled by clock signals, typically assigned using <=; combinational logic is logic not controlled by clock signals, assigned using =.
```verilog
module logic (
input wire A, B, // Input signals A, B
output reg Y_seq, Y_comb // Sequential logic output Y_seq, combinational logic output Y_comb
);
reg internal_reg; // Internal register
always @(posedge clk) begin
internal_reg <= A & B; // AND gate in sequential logic
end
assign Y_comb = A | B; // OR gate in combinational logic
assign Y_seq = internal_reg; // Output internal register value
endmodule
```
Mastering the basic concepts in Verilog is crucial for further study and application of the language. In practical digital circuit design, the proper use of modules, ports, signals, and logic types can significantly enhance the efficiency of design tasks.
# Procedural Statements in Verilog
Procedural statements in Verilog are an important way to describe the behavior of digital circuits. They can be used to simulate sequential logic and combinational logic within hardware. Through procedural statements, we can perform signal assignment, computation, and state transitions. This chapter will provide a detailed introduction to procedural statements in Verilog, including their functions, characteristics, and different types of procedural statements.
#### Functions and Characteristics of Procedural Statements
Procedural statements are primarily used to describe behavioral models in digital circuits, enabling signal assignment, logical operations, and state transitions. They are commonly used to simulate sequential logic, such as state machines driven by clock signals or sequential circuits. Characteristics of procedural statements include:
- Procedural statements are executed in an event-driven manner during simulation.
- They can be synchronous (executed on clock edges) or asynchronous (executed when signals change).
- Procedural statements can use blocking and non-blocking assignments.
#### Synchronous and Asynchronous Processes
In Verilog, procedural statements can be categorized into synchronous and asynchronous processes. Synchronous processes execute on the rising or falling edges of clock signals, and are often used to describe sequential logic; asynchronous processes execute immediately when external signals change, and are commonly used to describe combinational logic.
In synchronous processes, we typically use `always @(posedge clk)` to indicate that the process is executed on the rising edge of the clock signal. In asynchronous processes, `always @(*)` can be used to denote that the process executes immediately when signals change.
#### Detailed Explanation of the `always @` Statement
The `always @` statement is one of the key constructs in Verilog for describing procedural statements, ***mon uses include:
- `always @ (posedge clk)`: Execute process on the rising edge of the clock signal.
- `always @ (negedge rst)`: Execute process on the falling edge of the reset signal.
- `always @ (*)`: Execute process whenever any sensitive signal changes.
By properly utilizing the `always @` statement, we can clearly describe state transitions and logical operations within digital circuits. In practical applications, it is necessary to select the appropriate process type based on the specific scenario to ensure the accuracy and stability of circuit behavior.
Hopefully, this content will help you better understand procedural statements in Verilog. In the next section, we will introduce branch structures in Verilog.
# Branch Structures in Verilog
In Verilog, branch structures are a ***mon branch structures include if-else statements and case statements, which play a vital role in designing digital circuits. The following will detail the application methods and optimization techniques for branch structures in Verilog.
#### Application of if-else Statements
In Verilog, if-else statements are used to execute different blocks of code based on conditions. The basic syntax is as follows:
```verilog
if (condition1) begin
// Code block 1
end
else if (condition2) begin
// Code block 2
end
else begin
// Default code block
end
```
The condition can be a signal comparison or logical operation, etc. Below is a simple example showing the application of if-else statements:
```verilog
module if_else_example (
input logic a,
input logic b,
output logic y
);
always_comb begin
if (a & b) begin
y = 1;
end
else begin
y = 0;
end
end
endmodule
```
Code interpretation:
- If both input signals a and b are 1, then the output signal y is 1; otherwise, the output is 0.
#### Usage of case Statements
The case statement is another common branch structure suitable for multi-condition judgment scenarios. The basic syntax is as follows:
```verilog
case (expression)
pattern1: code block 1;
pattern2: code block 2;
...
default: default code block;
endcase
```
Below is a simple example demonstrating the usage of the case statement:
```verilog
module case_example (
input [1:0] sel,
output reg [3:0] y
);
always @* begin
case (sel)
2'b00: y = 4'b0001;
2'b01: y = 4'b0010;
2'b10: y = 4'b0100;
2'b11: y = 4'b1000;
default: y = 4'b0000;
endcase
end
endmodule
```
Code interpretation:
- Choose the corresponding assignment operation based on different values of the input signal sel.
#### Optimizing Code Logic with Branch Structures
When designing Verilog modules, the proper use of branch structures can simplify the logic, enhance readability, and maintainability of the code. Choosing between if-else statements or case statements wisely can make the code more clear and understandable.
This chapter has provided a detailed introduction to branch structures in Verilog, including the basic syntax and application methods of if-else statements and case statements. Reasonable use of branch structures is one of the keys to designing efficient digital circuits.
# In-depth Understanding of Verilog Procedural Statements and Branch Structures
In Verilog design, understanding and utilizing procedural statements and branch structures is crucial. This chapter will delve into the advanced applications of Verilog procedural statements and branch structures, aiding readers in better understanding and applying these concepts.
### Detailed Explanation of Sensitivity Lists in Procedural Statements
In Verilog, the sensitivity list of a procedural statement defines when the execution of the procedural block is triggered. In an `always @` statement, the sensitivity list specifies a list of signals to determine when any of the signals in the list change, the procedural block is executed. This flexible sensitivity mechanism enables the Verilog language to accurately capture signal changes and respond accordingly.
Here is a simple example:
```verilog
always @(posedge clk or posedge reset)
begin
if (reset)
count <= 0;
else
count <= count + 1;
end
```
In this example, the procedural block is executed on the rising edge of the clock signal (clk) or the rising edge of the reset signal (reset). If the reset signal is high, the count is reset to 0; otherwise, the count is incremented by 1.
### Combining Branch Structures for Complex Logic Implementation
In actual digital circuit design, there is often a need to implement complex logic. By combining procedural statements and branch structures, we can express various logical relationships more flexibly, thus realizing the design of complex circuit functions.
For example, the following is a Verilog code snippet that implements a simple selector logic using a case statement:
```verilog
always @ (sel)
begin
case(sel)
2'b00: out = in0;
2'b01: out = in1;
2'b10: out = in2;
2'b11: out = in3;
default: out = 4'b1111;
endcase
end
```
In this code, based on the different values of the sel signal, the corresponding input signal is selected and output to the out signal. If the value of sel exceeds the range defined in the case statement, the default statement will execute, assigning out to 4'b1111.
### Verilog Simulation Debugging Tips
During the Verilog design process, simulation and debugging are a crucial part. Using simulation tools, we can verify the correctness of the design, identify potential issues, and ultimately achieve the desired functionality.
Some commonly used Verilog simulation debugging tips include:
- Adding appropriate test vectors to cover various cases and validate the design as extensively as possible.
- Using simulation waveforms to view signal waveforms and ensure the timing and logic of the design are correct.
- Incorporating assertions (assertion) to verify certain assumptions about the design, ensuring the design behavior matches expectations.
In summary, a thorough understanding of Verilog procedural statements and branch structures, along with mastering simulation debugging techniques, will help design high-quality digital circuits and accelerate the entire design verification process.
# Case Studies and Applications
The field of digital circuit design is one of the most widely applied areas for Verilog language. This chapter will demonstrate the application of procedural statements and branch structures in Verilog through specific examples.
### The Application of Verilog Procedural Statements and Branch Structures in Digital Circuit Design
In digital circuit design, the procedural statements and branch structures in Verilog language play a crucial role. By properly utilizing these constructs, complex digital logic functions can be implemented, and the design's flexibility and maintainability can be enhanced.
### Designing a Simple Verilog Module
Next, we will present a simple Verilog module to illustrate the application of procedural statements and branch structures:
```verilog
module simple_module(
input a,
input b,
output reg c
);
always @ (a, b) begin
if(a & b) begin
c <= 1;
end else begin
c <= 0;
end
end
endmodule
```
**Explanation:**
- The `simple_module` has two input ports `a` and `b`, and one output port `c`.
- `always @ (a, b)` indicates that the internal logic of the `always` block is triggered when `a` or `b` changes.
- The `if-else` statement determines the value of output `c` based on the values of inputs `a` and `b`.
### Case Study: Implementing a Digital Clock Module
Finally, we will implement a digital clock module through a case study, where procedural statements and branch structures are combined to realize the functionality of a digital clock.
```verilog
module digital_clock(
output reg[3:0] hour,
output reg[5:0] minute,
output reg[5:0] second
);
reg[3:0] hour_reg;
reg[5:0] minute_reg;
reg[5:0] second_reg;
always @ (posedge clk) begin
if(second_reg == 59) begin
second_reg <= 0;
if(minute_reg == 59) begin
minute_reg <= 0;
if(hour_reg == 23) begin
hour_reg <= 0;
end else begin
hour_reg <= hour_reg + 1;
end
end else begin
minute_reg <= minute_reg + 1;
end
end else begin
second_reg <= second_reg + 1;
end
end
assign hour = hour_reg;
assign minute = minute_reg;
assign second = second_reg;
endmodule
```
**Explanation:**
- The `digital_clock` module implements a digital clock function, displaying hours, minutes, and seconds.
- The timing functionality of the clock is achieved through `always @ (posedge clk)`, updating the displayed time based on the changes in seconds, minutes, and hours.
- Nested `if-else` statements are used to implement the increment and carry operations for hours, minutes, and seconds.
Through the above examples, we can see the flexible application of procedural statements and branch structures in Verilog, capable of realizing complex digital logic functionality, making it highly suitable for applications in the field of digital circuit design.
0
0
相关推荐
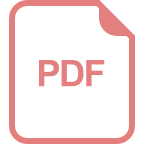
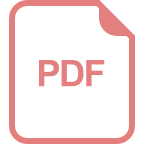
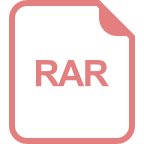
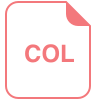
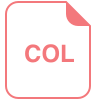
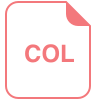
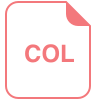
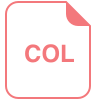
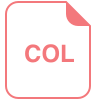