我想要一个esp32使用u8g2驱动ssd1306显示温度曲线的程序
时间: 2024-01-21 08:19:40 浏览: 165
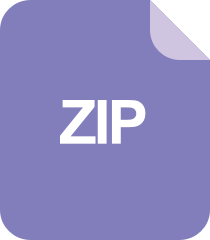
U8G2(SSD1306)移植例程 ESP01S(硬件IIC) ESP8266_RTOS_SDK 非arduino

好的,以下是一个基于esp32和u8g2库的示例代码,可以用于显示温度曲线:
```C++
#include <Adafruit_Sensor.h>
#include <DHT.h>
#include <u8g2.h>
#define DHTPIN 4
#define DHTTYPE DHT11
DHT dht(DHTPIN, DHTTYPE);
u8g2_t u8g2;
float temperature;
int x = 0, y = 0;
void setup() {
u8g2.begin();
dht.begin();
}
void loop() {
temperature = dht.readTemperature();
u8g2.firstPage();
do {
u8g2.drawPixel(x, y, 1);
x++;
y = (int) ((temperature + 20) * 2.5);
if (x >= 128) {
x = 0;
u8g2.clearBuffer();
}
} while (u8g2.nextPage());
delay(1000);
}
```
这个程序使用DHT11传感器读取温度,并将温度转换为y坐标值,然后在128x64 OLED屏幕上绘制温度曲线。每秒钟更新一次曲线。你需要将代码中的DHTPIN替换为你的DHT11传感器连接到ESP32的引脚。
阅读全文
相关推荐
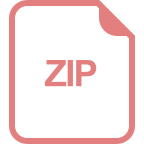
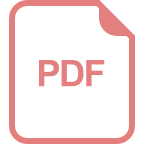














