定义一个交通工具(Vehicle)的类,定义私有属性: 速度(speed)、方向(direction),定义方法:设置速度setSpeed(int speed))、改变速度changeSpeed(int speed),设置方向setDirection(int direction),改变速度changeDirection(int direction)。最后在测试类 Vehicle 中的 main()中实例化一个交通工具对象 direction: 1:North 2:South 3:East 4:West 输入:一行两个数 第一个数表示调用哪个方法(1:setSpeed 2:changeSpeed 3:setDirection 4:changeDirection) 第二个数表示输入的值 输出:方法名和私有属性的值 初始化速度为0,方向为North 例 输入: 1 1 输出: setSpeed speed = 1 direction = North
时间: 2023-06-04 09:05:02 浏览: 130
好的,我可以帮您回答这个问题。
以下是定义一个交通工具(Vehicle)的类的代码:
```
public class Vehicle {
private int speed;
private int direction;
public void setSpeed(int speed) {
this.speed = speed;
}
public void changeSpeed(int speed) {
this.speed += speed;
}
public void setDirection(int direction) {
this.direction = direction;
}
public void changeDirection(int direction) {
this.direction += direction;
}
public int getSpeed() {
return speed;
}
public int getDirection() {
return direction;
}
}
```
在测试类Vehicle的main()中实例化一个交通工具对象并进行操作,代码如下:
```
import java.util.Scanner;
public class VehicleTest {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
Vehicle vehicle = new Vehicle();
int method = scanner.nextInt();
int value = scanner.nextInt();
switch (method) {
case 1:
vehicle.setSpeed(value);
System.out.println("setSpeed speed = " + vehicle.getSpeed() + " direction = " + getDirection(vehicle.getDirection()));
break;
case 2:
vehicle.changeSpeed(value);
System.out.println("changeSpeed speed = " + vehicle.getSpeed() + " direction = " + getDirection(vehicle.getDirection()));
break;
case 3:
vehicle.setDirection(value);
System.out.println("setDirection speed = " + vehicle.getSpeed() + " direction = " + getDirection(vehicle.getDirection()));
break;
case 4:
vehicle.changeDirection(value);
System.out.println("changeDirection speed = " + vehicle.getSpeed() + " direction = " + getDirection(vehicle.getDirection()));
break;
default:
System.out.println("Invalid method");
}
}
public static String getDirection(int direction) {
switch (direction) {
case 1:
return "North";
case 2:
return "South";
case 3:
return "East";
case 4:
return "West";
default:
return "Unknown";
}
}
}
```
使用时,输入数字1到4表示调用哪个方法,后面输入一个参数作为方法的参数。程序会根据输入的参数执行相应的方法并输出私有属性的值以及方法名。
这是一个简单的交通工具类,可以通过改变速度和方向来模拟交通工具的运动。注意,这里只提供了基本的方法,实际开发中可能需要添加更多的属性和方法。
阅读全文
相关推荐
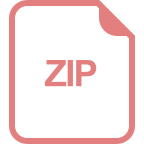
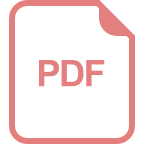



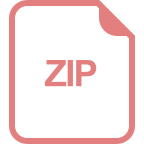
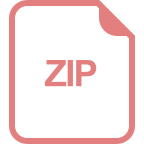
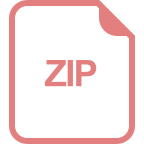
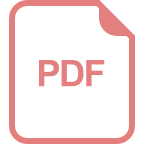
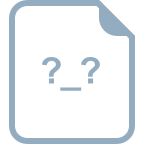
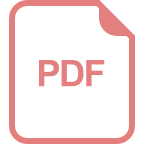
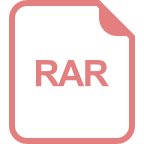
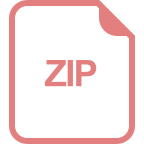
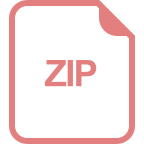
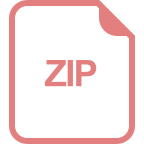
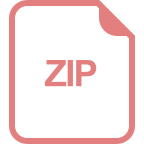
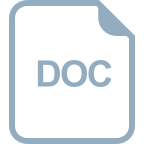

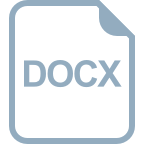