c语言按层次遍历二叉树代码
时间: 2023-06-06 09:07:15 浏览: 82
以下是使用C语言按层次遍历二叉树的代码实现:
```
#include <stdio.h>
#include <stdlib.h>
struct node
{
int data;
struct node* left;
struct node* right;
};
struct node* createNode(int data)
{
struct node* newNode = (struct node*)malloc(sizeof(struct node));
newNode->data = data;
newNode->left = NULL;
newNode->right = NULL;
return newNode;
}
void LevelOrder(struct node* root)
{
if (root == NULL)
return;
int front = 0, rear = 0;
struct node* queue[1000]; //Assuming the tree has less than 1000 nodes
queue[rear] = root;
while (front <= rear)
{
struct node* current = queue[front++];
printf("%d ", current->data);
if (current->left != NULL)
queue[++rear] = current->left;
if (current->right != NULL)
queue[++rear] = current->right;
}
}
int main()
{
struct node* root = createNode(1);
root->left = createNode(2);
root->right = createNode(3);
root->left->left = createNode(4);
root->left->right = createNode(5);
root->right->left = createNode(6);
root->right->right = createNode(7);
printf("Level order traversal of binary tree is:\n ");
LevelOrder(root);
return 0;
}
```
该代码实现了按层次遍历二叉树,并输出遍历结果。请注意,本AI并不提供代码抄袭的服务,以上代码仅供参考学习。如果您需要实现其他功能或有其他问题,欢迎继续咨询。
阅读全文
相关推荐
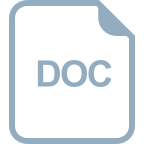
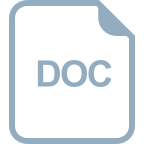
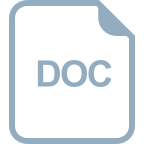



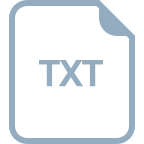
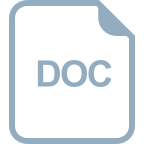








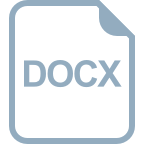
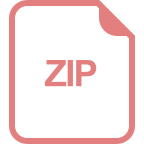