Can't call numpy() on Tensor that requires grad. Use tensor.detach().numpy() instead.这个错误怎么解决
时间: 2023-12-12 08:09:15 浏览: 29
这个错误通常发生在将需要梯度的`Tensor`转换为`numpy`数组时。由于`Tensor`需要梯度,因此直接使用`numpy()`方法将其转换为`numpy`数组会导致错误。
要解决这个问题,可以使用`detach()`方法将`Tensor`分离出来,然后再使用`numpy()`方法将其转换为`numpy`数组。下面是一个例子:
```python
import torch
# 创建一个需要梯度的Tensor
x = torch.randn(3, 3, requires_grad=True)
# 尝试将x转换为numpy数组
y = x.numpy() # 这里会抛出错误
# 使用detach()方法将x分离出来,然后转换为numpy数组
z = x.detach().numpy()
```
在上面的例子中,我们首先创建了一个需要梯度的`Tensor`,然后尝试将其转换为`numpy`数组,这会抛出错误。然后我们使用`detach()`方法将`Tensor`分离出来,然后再使用`numpy()`方法将其转换为`numpy`数组,这样就不会再出现错误了。
需要注意的是,`detach()`方法返回的是一个新的`Tensor`,与原始的`Tensor`共享数据,但是不再具有梯度。如果需要保留梯度信息,可以使用`clone()`方法创建一个新的`Tensor`,然后再调用`detach()`方法。
相关问题
Can't call numpy() on Tensor that requires grad. Use tensor.detach().numpy() instead
This error occurs when you try to call the numpy method on a PyTorch tensor that requires gradient computation. To fix this error, you need to detach the tensor from the computation graph before calling the numpy method. You can do this by calling the detach() method on the tensor before calling numpy().
For example:
```
import torch
# create a tensor
x = torch.tensor([1, 2, 3], requires_grad=True)
# detach the tensor from the computation graph
x_np = x.detach().numpy()
# print the numpy array
print(x_np)
```
In the code above, we first create a tensor and set the requires_grad flag to True, indicating that we want to compute gradients with respect to this tensor. We then detach the tensor from the computation graph by calling the detach() method on it. Finally, we call the numpy method on the detached tensor to get the corresponding numpy array.
Can't call numpy() on Tensor that requires grad. Use tensor.detach().numpy() instead.
This error occurs when you try to call the `numpy()` method on a PyTorch tensor that requires gradient computation. To fix this, you can use the `detach()` method to create a new tensor that does not require gradient computation and then call the `numpy()` method on the detached tensor. Here's an example:
```
import torch
# create a tensor and set requires_grad=True
x = torch.ones(2, 2, requires_grad=True)
# perform some operations on the tensor
y = x + 2
z = y * y * 3
out = z.mean()
# call the numpy() method on the tensor
# this will raise an error
# np_array = out.numpy()
# detach the tensor and call the numpy() method on the detached tensor
np_array = out.detach().numpy()
print(np_array)
```
In this example, we create a tensor `x` with `requires_grad=True`, perform some operations on it, and compute a scalar value `out`. When we try to call the `numpy()` method on `out`, we get the error message. To fix this, we detach `out` using the `detach()` method and then call the `numpy()` method on the detached tensor.
相关推荐
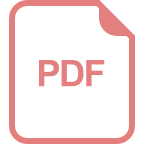




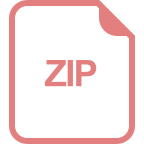
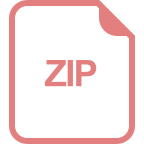
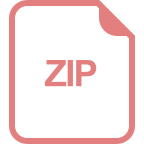
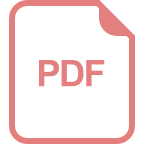