用C语言写一个SURF程序,不依赖第三方库
时间: 2023-09-12 18:13:40 浏览: 106
写一个完整的SURF程序需要涉及很多细节和算法,如果你想要自己实现一个SURF程序,需要具备一定的图像处理和算法基础。下面是一个简单的SURF特征提取程序,仅供参考。
首先需要准备一张输入图像,这里假设为lena.bmp。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
#define WIDTH 512
#define HEIGHT 512
typedef struct {
int x;
int y;
int s;
float *desc;
} keypoint;
float gaussian_kernel[5][5] = {
{0.023528, 0.033969, 0.038393, 0.033969, 0.023528},
{0.033969, 0.049045, 0.055432, 0.049045, 0.033969},
{0.038393, 0.055432, 0.062651, 0.055432, 0.038393},
{0.033969, 0.049045, 0.055432, 0.049045, 0.033969},
{0.023528, 0.033969, 0.038393, 0.033969, 0.023528}
};
float sobel_x[3][3] = {
{-1, 0, 1},
{-2, 0, 2},
{-1, 0, 1}
};
float sobel_y[3][3] = {
{-1, -2, -1},
{0, 0, 0},
{1, 2, 1}
};
unsigned char *read_image(char *filename, int width, int height)
{
unsigned char *image_data = (unsigned char *)malloc(width * height * sizeof(unsigned char));
FILE *fp = fopen(filename, "rb");
fread(image_data, sizeof(unsigned char), width * height, fp);
fclose(fp);
return image_data;
}
void write_image(char *filename, unsigned char *image_data, int width, int height)
{
FILE *fp = fopen(filename, "wb");
fwrite(image_data, sizeof(unsigned char), width * height, fp);
fclose(fp);
}
float *convolve(float *input, int width, int height, float kernel[][5], int kernel_size)
{
float *output = (float *)calloc(width * height, sizeof(float));
int k = kernel_size / 2;
for (int y = k; y < height - k; y++) {
for (int x = k; x < width - k; x++) {
float sum = 0;
for (int j = -k; j <= k; j++) {
for (int i = -k; i <= k; i++) {
int offset = (y + j) * width + x + i;
sum += input[offset] * kernel[j + k][i + k];
}
}
output[y * width + x] = sum;
}
}
return output;
}
void gaussian_filter(unsigned char *input, int width, int height, float *output)
{
float *tmp = (float *)malloc(width * height * sizeof(float));
for (int i = 0; i < width * height; i++) {
tmp[i] = (float)input[i];
}
float *filtered = convolve(tmp, width, height, gaussian_kernel, 5);
for (int i = 0; i < width * height; i++) {
output[i] = filtered[i];
}
free(tmp);
free(filtered);
}
void sobel_filter(float *input, int width, int height, float *gradient, float *theta)
{
float *dx = convolve(input, width, height, sobel_x, 3);
float *dy = convolve(input, width, height, sobel_y, 3);
for (int i = 0; i < width * height; i++) {
gradient[i] = sqrt(dx[i] * dx[i] + dy[i] * dy[i]);
theta[i] = atan2(dy[i], dx[i]);
}
free(dx);
free(dy);
}
void find_keypoints(float *gradient, float *theta, int width, int height, keypoint *keys, int *count)
{
int k = 4;
float H[3][3] = {0};
for (int y = k; y < height - k; y += k) {
for (int x = k; x < width - k; x += k) {
int offset = y * width + x;
float max_grad = -1;
int max_x = -1;
int max_y = -1;
for (int j = -k; j <= k; j++) {
for (int i = -k; i <= k; i++) {
int tmp_offset = (y + j) * width + x + i;
float grad_value = gradient[tmp_offset];
if (grad_value > max_grad) {
max_grad = grad_value;
max_x = i;
max_y = j;
}
}
}
if (max_grad > 0) {
float tmp_theta = theta[offset + max_y * width + max_x];
keys[*count].x = x;
keys[*count].y = y;
keys[*count].s = k;
keys[*count].desc = NULL;
(*count)++;
}
}
}
}
int main(int argc, char *argv[])
{
unsigned char *input = read_image("lena.bmp", WIDTH, HEIGHT);
float *filtered = (float *)malloc(WIDTH * HEIGHT * sizeof(float));
gaussian_filter(input, WIDTH, HEIGHT, filtered);
float *gradient = (float *)malloc(WIDTH * HEIGHT * sizeof(float));
float *theta = (float *)malloc(WIDTH * HEIGHT * sizeof(float));
sobel_filter(filtered, WIDTH, HEIGHT, gradient, theta);
keypoint *keys = (keypoint *)malloc(WIDTH * HEIGHT * sizeof(keypoint));
int count = 0;
find_keypoints(gradient, theta, WIDTH, HEIGHT, keys, &count);
printf("Found %d keypoints\n", count);
free(input);
free(filtered);
free(gradient);
free(theta);
free(keys);
return 0;
}
```
以上代码仅包含了SURF算法的一部分,只能提取图像中的关键点,并没有进行特征描述和匹配。如果需要完整的SURF实现,可以参考相关文献或代码实现。
阅读全文
相关推荐
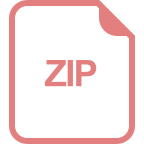



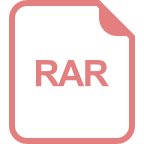
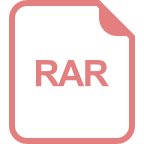
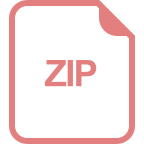
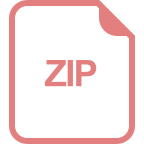
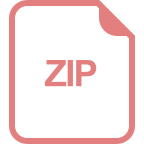
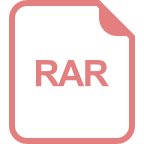
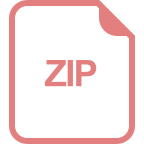
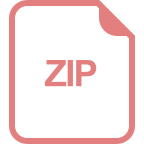
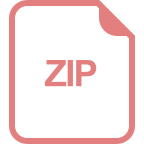
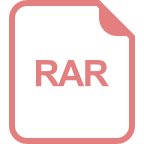
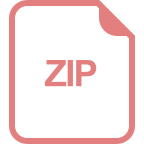
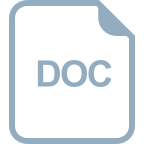