tensorrt python部署
时间: 2023-08-29 08:10:33 浏览: 53
TensorRT是一个高性能深度学习推理引擎,可以将训练好的模型优化并部署在GPU上进行推理。在Python中使用TensorRT进行深度学习模型的部署,可以通过以下步骤实现:
1. 安装TensorRT
在官网上下载适合自己系统的TensorRT安装包,然后按照官方文档进行安装。
2. 利用TensorFlow或PyTorch训练好模型
使用TensorFlow或PyTorch训练好模型,保存模型文件。
3. 使用TensorRT优化模型
使用TensorRT提供的工具将训练好的模型优化成可在GPU上进行推理的模型。具体操作可以参考TensorRT官方文档。
4. 在Python中加载TensorRT模型
使用TensorRT提供的Python API,在Python中加载优化后的模型,进行推理操作。具体操作可以参考TensorRT官方文档。
下面是一个使用TensorRT进行图像分类的Python示例代码:
```python
import tensorrt as trt
import pycuda.driver as cuda
import pycuda.autoinit
import numpy as np
import cv2
# 加载优化后的模型
def load_engine(engine_path):
with open(engine_path, 'rb') as f:
engine_data = f.read()
engine = trt.lite.Engine(None, engine_data)
return engine
# 进行推理操作
def inference(engine, input_image):
# 获取输入输出张量
input_tensor = engine.get_input_tensor(0)
output_tensor = engine.get_output_tensor(0)
# 将输入图像转换为模型输入张量格式
input_image = cv2.cvtColor(input_image, cv2.COLOR_BGR2RGB)
input_image = cv2.resize(input_image, (input_tensor.shape[3], input_tensor.shape[2]))
input_image = np.transpose(input_image, [2, 0, 1])
input_image = np.expand_dims(input_image, axis=0)
# 创建输入输出映射表
bindings = [int(input_tensor.device_buffer), int(output_tensor.device_buffer)]
# 创建执行上下文
context = engine.create_execution_context()
# 将输入数据复制到GPU
cuda.memcpy_htod(input_tensor.device_buffer, input_image)
# 执行推理
context.execute_v2(bindings)
# 将输出数据从GPU复制回CPU
output = np.empty(output_tensor.shape, dtype=np.float32)
cuda.memcpy_dtoh(output, output_tensor.device_buffer)
# 获取分类结果
class_id = np.argmax(output)
return class_id
# 加载优化后的模型
engine_path = 'model.trt'
engine = load_engine(engine_path)
# 进行推理操作
input_image = cv2.imread('test.jpg')
class_id = inference(engine, input_image)
print('预测结果:{}'.format(class_id))
```
需要注意的是,TensorRT只支持FP16和INT8数据类型,如果模型中有其他数据类型,需要进行转换。同时,TensorRT只支持静态图模型,如果使用动态图框架训练的模型,需要先将其转换为静态图模型。
相关推荐
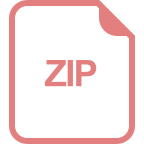














