编程实现:从键盘输入两个整数n和m,计算n的m次方,输出结果。
时间: 2024-10-06 18:02:03 浏览: 82
要使用C语言编写程序来计算任意两个整数`n`和`m`之间的幂运算,你可以使用循环或递归来实现。这里我会提供两种方法的示例代码:
**使用循环(迭代法):**
```c
#include <stdio.h>
int power(int base, int exponent) {
int result = 1;
for (int i = 0; i < exponent; i++) {
result *= base;
}
return result;
}
int main() {
int n, m;
printf("Enter the base number (n): ");
scanf("%d", &n);
printf("Enter the exponent (m): ");
scanf("%d", &m);
int result = power(n, m);
printf("The %dth power of %d is %d.\n", m, n, result);
return 0;
}
```
在这个例子中,我们定义了一个名为`power`的函数,它接受两个整数参数并返回它们的乘积(即幂运算的结果)。在主函数里,用户输入n和m,然后调用这个函数。
**使用递归(递归法):**
```c
#include <stdio.h>
// 递归版本的pow函数
int power(int base, int exponent) {
if (exponent == 0) {
return 1; // 当指数为0时,任何数的0次方都是1
} else if (exponent > 0) {
return base * power(base, exponent - 1); // 递归调用,减小指数
} else { // 如果指数小于0,则先取倒数再乘
return 1 / power(base, -exponent); // 注意此处除以1是因为负指数表示取倒数
}
}
int main() {
int n, m;
printf("Enter the base number (n): ");
scanf("%d", &n);
printf("Enter the exponent (m): ");
scanf("%d", &m);
int result = power(n, m);
printf("The %dth power of %d is %d.\n", m, n, result);
return 0;
}
```
在这段代码中,我们使用了递归的方式来实现幂运算。当指数减少到0时,递归结束,否则继续递归,直到达到基本情况。
阅读全文
相关推荐




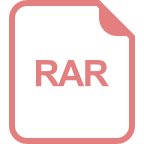













