写代码,实现一个分布式网络爬虫
时间: 2024-06-08 07:06:03 浏览: 23
实现一个分布式网络爬虫需要以下步骤:
1. 确定需要爬取的网站和数据结构
2. 设计爬取策略,如广度优先、深度优先等
3. 使用分布式技术,将爬取任务分配给不同的节点
4. 实现节点间的通信和数据同步
5. 解析网页并提取所需信息
6. 存储数据并进行去重处理
7. 定期更新和维护爬虫
下面是一个简单的分布式网络爬虫实现示例:
```python
import requests
from bs4 import BeautifulSoup
import redis
from multiprocessing import Pool, cpu_count
# 爬取的网站
url = 'https://www.example.com'
# Redis数据库
redis_db = redis.StrictRedis()
# 定义爬取函数
def crawl(url):
try:
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
# 提取所需信息
data = soup.select('...')
# 存储数据
redis_db.sadd('data', data)
# 提取链接
links = soup.select('a')
for link in links:
href = link.get('href')
if href.startswith('https://www.example.com'):
# 将链接加入Redis队列
redis_db.rpush('queue', href)
else:
print('Failed to crawl', url)
except Exception as e:
print(e)
# 定义爬虫函数
def spider():
while True:
# 从Redis队列中取出链接
url = redis_db.lpop('queue')
if url:
crawl(url)
else:
break
# 分布式爬虫
if __name__ == '__main__':
# 初始化队列
redis_db.delete('queue')
redis_db.delete('data')
redis_db.rpush('queue', url)
# 启动多进程
pool = Pool(cpu_count())
pool.map(spider, range(cpu_count()))
# 输出结果
print('Crawling finished')
```
该示例使用了Redis作为分布式队列,使用了多进程实现分布式爬虫。在实际应用中,还需要考虑反爬虫策略、IP代理、用户代理等问题。
相关推荐
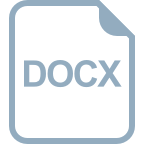
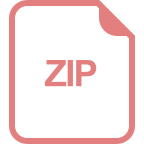
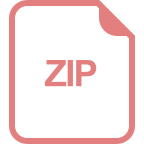
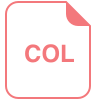
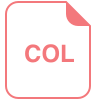
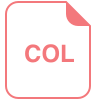
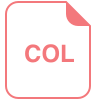
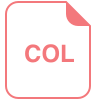










