pthread_create强制退出
时间: 2024-12-17 19:43:09 浏览: 2
`pthread_create`是POSIX线程库中的一个函数,用于创建新的线程。它并不会直接导致现有线程的强制退出。如果你希望在一个线程中强制另一个线程停止运行,通常需要通过一些同步机制,如信号(`kill`, `pthread_kill`)、条件变量(`pthread_mutex_destroy`配合`pthread_cond_wait`)或者共享内存(通过设置标志位通知其他线程退出)来实现。
如果你想在主线程中终止某个特定的子线程,可以在线程函数中处理相应的终止请求,比如检查一个退出标记或者捕获某种特殊类型的异常。例如:
```c
void *thread_function(void *arg) {
// ... 线程业务逻辑
if (/* 检查是否收到退出请求 */) {
pthread_exit(NULL); // 主动退出
}
return NULL;
}
int main() {
pthread_t thread_id;
int exit_flag = 0; // 存储退出标志
pthread_create(&thread_id, NULL, thread_function, &exit_flag);
// 后续主线程可以修改exit_flag来终止子线程
exit_flag = 1;
// 等待子线程结束
pthread_join(thread_id, NULL);
return 0;
}
```
相关问题
pthread_create传递多个参数的方法
可以通过定义一个结构体来包含需要传递的多个参数,然后将该结构体的指针作为参数传递给pthread_create函数。示例代码如下:
```
#include <pthread.h>
#include <stdio.h>
struct thread_data {
int arg1;
char arg2;
float arg3;
};
void *thread_function(void *arg) {
struct thread_data *my_data;
my_data = (struct thread_data *) arg;
printf("arg1 = %d, arg2 = %c, arg3 = %f\n", my_data->arg1, my_data->arg2, my_data->arg3);
pthread_exit(NULL);
}
int main() {
pthread_t my_thread;
struct thread_data data;
data.arg1 = 10;
data.arg2 = 'a';
data.arg3 = 3.14;
int ret = pthread_create(&my_thread, NULL, thread_function, (void *)&data);
if(ret != 0) {
printf("Error: pthread_create failed\n");
return -1;
}
pthread_join(my_thread, NULL);
return 0;
}
```
在上述示例中,定义了一个结构体thread_data,包含需要传递的三个参数arg1、arg2和arg3。在主函数中创建了一个thread_data类型的变量data,并且给其三个成员赋值。然后将data的地址作为参数传递给pthread_create函数。在线程函数thread_function中,通过强制类型转换将参数转换为thread_data类型,然后访问其三个成员,最后退出线程。主函数中使用pthread_join等待线程结束。
pthread_create()中start_routine的示例
下面是一个简单的示例,展示了如何在pthread_create()中使用start_routine参数:
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
void *print_message(void *arg) {
char *message = (char *) arg;
printf("%s\n", message);
pthread_exit(NULL);
}
int main() {
pthread_t thread;
char *message = "Hello, world!";
int result = pthread_create(&thread, NULL, print_message, (void *) message);
if (result) {
printf("Error creating thread: %d\n", result);
exit(EXIT_FAILURE);
}
pthread_exit(NULL);
}
```
在这个示例中,我们定义了一个名为print_message()的函数作为我们要在新线程中运行的start_routine。该函数接受一个void指针参数,我们在调用pthread_create()时将message指针传递给该参数。在print_message()函数中,我们将该参数强制转换为char指针,然后将其打印到控制台中。
在主函数中,我们创建了一个名为thread的pthread_t变量,并将其传递给pthread_create()函数。如果成功创建线程,则pthread_create()函数将返回0,否则返回一个非零值。在这个示例中,我们简单地检查返回值,如果有错误,则打印错误消息并退出程序。
最后,在主函数的末尾,我们调用pthread_exit(NULL),以确保主线程等待新线程完成执行后再退出。
阅读全文
相关推荐
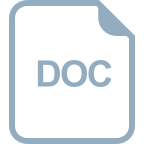
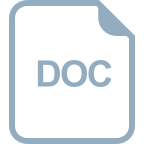
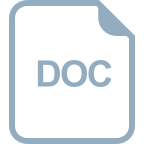




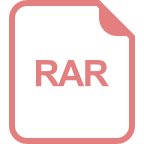

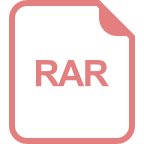
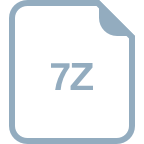
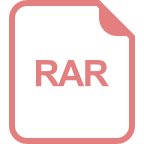
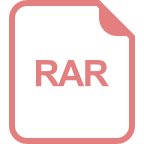
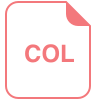
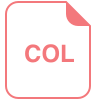

