Python语言编写一个台球小游戏
时间: 2023-07-07 10:15:33 浏览: 203
好的,我可以给你提供一个简单的 Python 台球小游戏的代码示例。这个游戏是一个单人游戏,玩家需要控制一个白球,用它撞击其他球,使它们进袋。以下是示例代码:
```python
import pygame
import sys
import math
import random
# 初始化 Pygame
pygame.init()
# 游戏窗口大小
size = (800, 600)
# 游戏窗口
screen = pygame.display.set_mode(size)
pygame.display.set_caption("台球小游戏")
# 颜色
white = (255, 255, 255)
black = (0, 0, 0)
red = (255, 0, 0)
green = (0, 255, 0)
blue = (0, 0, 255)
# 球的参数
ball_radius = 20
ball_color = white
ball_speed = 5
# 洞的参数
hole_radius = 30
hole_color = black
hole_positions = [(hole_radius, hole_radius),
(size[0] - hole_radius, hole_radius),
(hole_radius, size[1] - hole_radius),
(size[0] - hole_radius, size[1] - hole_radius)]
# 碰撞检测函数
def is_collision(ball_x, ball_y, hole_x, hole_y):
distance = math.sqrt((ball_x - hole_x) ** 2 + (ball_y - hole_y) ** 2)
if distance < ball_radius + hole_radius:
return True
else:
return False
# 创建球和洞
balls = []
for i in range(6):
ball = {'rect': pygame.Rect(random.randint(ball_radius, size[0] - ball_radius),
random.randint(ball_radius, size[1] - ball_radius),
ball_radius * 2, ball_radius * 2),
'color': ball_color,
'speed': ball_speed,
'direction': random.randint(0, 360)}
balls.append(ball)
holes = []
for pos in hole_positions:
hole = {'rect': pygame.Rect(pos[0] - hole_radius, pos[1] - hole_radius,
hole_radius * 2, hole_radius * 2),
'color': hole_color}
holes.append(hole)
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# 获取按键状态
keys = pygame.key.get_pressed()
# 移动白球
if keys[pygame.K_LEFT]:
balls[0]['rect'].left -= balls[0]['speed']
if keys[pygame.K_RIGHT]:
balls[0]['rect'].left += balls[0]['speed']
if keys[pygame.K_UP]:
balls[0]['rect'].top -= balls[0]['speed']
if keys[pygame.K_DOWN]:
balls[0]['rect'].top += balls[0]['speed']
# 碰撞检测
for i in range(len(balls)):
for j in range(len(holes)):
if is_collision(balls[i]['rect'].centerx, balls[i]['rect'].centery,
holes[j]['rect'].centerx, holes[j]['rect'].centery):
balls.pop(i)
holes.pop(j)
# 绘制游戏界面
screen.fill(green)
for hole in holes:
pygame.draw.circle(screen, hole['color'], hole['rect'].center, hole_radius)
for ball in balls:
pygame.draw.circle(screen, ball['color'], ball['rect'].center, ball_radius)
ball['rect'].left += ball['speed'] * math.cos(ball['direction'] * math.pi / 180)
ball['rect'].top += ball['speed'] * math.sin(ball['direction'] * math.pi / 180)
if ball['rect'].left < ball_radius or ball['rect'].right > size[0] - ball_radius:
ball['direction'] = 180 - ball['direction']
if ball['rect'].top < ball_radius or ball['rect'].bottom > size[1] - ball_radius:
ball['direction'] = -ball['direction']
pygame.display.update()
```
以上代码会创建一个带6个球和4个洞的游戏界面,玩家可以控制白球移动,用它撞击其他球,使它们进袋。球在碰到边界时会弹回,撞击后会改变方向。当所有球都进袋时,游戏结束。
相关推荐
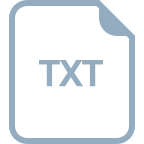
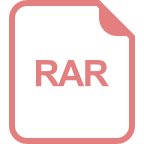
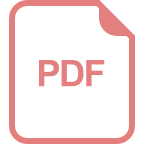















