用建造者模式实现计算机的“计算机组装”类。Java
时间: 2024-05-25 19:17:56 浏览: 9
public class ComputerBuilder {
private String cpu;
private String gpu;
private String motherboard;
private String memory;
private String storage;
private String powerSupply;
public ComputerBuilder() {
}
public ComputerBuilder setCpu(String cpu) {
this.cpu = cpu;
return this;
}
public ComputerBuilder setGpu(String gpu) {
this.gpu = gpu;
return this;
}
public ComputerBuilder setMotherboard(String motherboard) {
this.motherboard = motherboard;
return this;
}
public ComputerBuilder setMemory(String memory) {
this.memory = memory;
return this;
}
public ComputerBuilder setStorage(String storage) {
this.storage = storage;
return this;
}
public ComputerBuilder setPowerSupply(String powerSupply) {
this.powerSupply = powerSupply;
return this;
}
public Computer build() {
return new Computer(cpu, gpu, motherboard, memory, storage, powerSupply);
}
}
public class Computer {
private String cpu;
private String gpu;
private String motherboard;
private String memory;
private String storage;
private String powerSupply;
public Computer(String cpu, String gpu, String motherboard, String memory, String storage, String powerSupply) {
this.cpu = cpu;
this.gpu = gpu;
this.motherboard = motherboard;
this.memory = memory;
this.storage = storage;
this.powerSupply = powerSupply;
}
public String getCpu() {
return cpu;
}
public String getGpu() {
return gpu;
}
public String getMotherboard() {
return motherboard;
}
public String getMemory() {
return memory;
}
public String getStorage() {
return storage;
}
public String getPowerSupply() {
return powerSupply;
}
}
public class TestComputerBuilder {
public static void main(String[] args) {
Computer computer = new ComputerBuilder()
.setCpu("i7-10700K")
.setGpu("RTX 3070")
.setMotherboard("ASUS ROG Strix Z490-E")
.setMemory("Corsair Vengeance RGB Pro 16GB")
.setStorage("Samsung 970 EVO Plus 1TB")
.setPowerSupply("Corsair RM850x")
.build();
System.out.println("CPU: " + computer.getCpu());
System.out.println("GPU: " + computer.getGpu());
System.out.println("Motherboard: " + computer.getMotherboard());
System.out.println("Memory: " + computer.getMemory());
System.out.println("Storage: " + computer.getStorage());
System.out.println("Power Supply: " + computer.getPowerSupply());
}
}
相关推荐
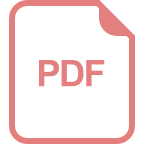
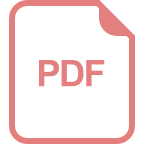














