vue移动端实现横屏手写签名并且可以保存和重签
时间: 2023-09-11 10:08:38 浏览: 97
对于Vue移动端实现横屏手写签名,可以使用HTML5的Canvas元素结合touch事件来实现。具体步骤如下:
1. 在Vue组件中引入Canvas元素,并为其设置样式。
2. 监听touch事件,获取手写路径的坐标,并使用Canvas绘制。
3. 提供保存和重签功能,可以使用Canvas的toDataURL()方法将签名转为图片格式保存,并清空Canvas重新开始签名。
下面是一个简单的示例代码,供你参考:
```
<template>
<div class="signature">
<canvas ref="canvas" class="canvas"></canvas>
<div class="btns">
<button @click="save">保存</button>
<button @click="clear">重签</button>
</div>
</div>
</template>
<script>
export default {
data() {
return {
canvas: null,
ctx: null,
drawing: false,
lastX: null,
lastY: null,
lineWidth: 2,
};
},
mounted() {
this.canvas = this.$refs.canvas;
this.ctx = this.canvas.getContext('2d');
this.canvas.width = window.innerWidth;
this.canvas.height = window.innerHeight;
this.ctx.strokeStyle = '#000000';
this.ctx.lineWidth = this.lineWidth;
this.ctx.lineJoin = 'round';
this.ctx.lineCap = 'round';
this.canvas.addEventListener('touchstart', this.startDrawing);
this.canvas.addEventListener('touchmove', this.draw);
this.canvas.addEventListener('touchend', this.stopDrawing);
},
methods: {
startDrawing(e) {
this.drawing = true;
this.lastX = e.touches[0].clientX;
this.lastY = e.touches[0].clientY;
},
draw(e) {
if (!this.drawing) return;
const x = e.touches[0].clientX;
const y = e.touches[0].clientY;
this.ctx.beginPath();
this.ctx.moveTo(this.lastX, this.lastY);
this.ctx.lineTo(x, y);
this.ctx.stroke();
this.lastX = x;
this.lastY = y;
},
stopDrawing() {
this.drawing = false;
},
save() {
const dataURL = this.canvas.toDataURL();
// 将dataURL保存到本地或上传到服务器
},
clear() {
this.ctx.clearRect(0, 0, this.canvas.width, this.canvas.height);
},
},
};
</script>
<style scoped>
.signature {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: #ffffff;
}
.canvas {
width: 100%;
height: 100%;
}
.btns {
position: absolute;
bottom: 20px;
left: 50%;
transform: translateX(-50%);
}
button {
margin-right: 10px;
background-color: #000000;
color: #ffffff;
border: none;
padding: 10px 20px;
border-radius: 5px;
font-size: 16px;
cursor: pointer;
}
</style>
```
注意,由于移动端横屏显示,需要在head标签中添加以下meta标签:
```
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=no, orientation=landscape">
```
相关推荐
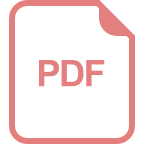
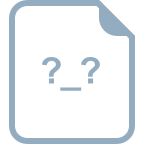
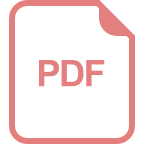













