汉诺塔圆盘6个
时间: 2023-11-27 22:53:26 浏览: 106
汉诺塔是一个著名的数学问题,它的规则如下:
有三根柱子和N个圆盘,初始时圆盘从小到大依次放在第一根柱子上,要求把所有圆盘移到第三根柱子上,且每次只能移动一个圆盘,且大盘不能放在小盘上面。
对于6个圆盘的汉诺塔问题,需要64次移动才能完成。具体的移动顺序可以通过递归算法实现,以下是Python代码实现:
```
def hanoi(n, a, b, c):
if n == 1:
print(a, '->', c)
else:
hanoi(n-1, a, c, b)
print(a, '->', c)
hanoi(n-1, b, a, c)
hanoi(6, 'A', 'B', 'C')
```
其中,n表示圆盘的数量,a、b、c分别表示三根柱子的名称。执行上述代码,即可输出6个圆盘的移动方案。
相关问题
汉诺塔圆盘6个,计算公式及其推断方法
汉诺塔问题是经典的递归问题,其规则为:有三根柱子,其中一根柱子上从下往上按大小顺序摆放着n个圆盘,要求把所有圆盘移动到另一根柱子上,并且每次移动同一根柱子上只能放置一个圆盘,且大盘不能放在小盘上面。现在考虑汉诺塔圆盘6个的情况。
移动6个圆盘的汉诺塔问题可以拆解为以下3个子问题:
1. 将前5个圆盘从A柱移动到B柱;
2. 将第6个圆盘从A柱移动到C柱;
3. 将前5个圆盘从B柱移动到C柱。
对于子问题1和子问题3,可以采用递归的方式继续拆解。具体地,将子问题1看作一个新的汉诺塔问题,即将前4个圆盘从A柱移动到C柱,这个问题可以继续拆解为将前3个圆盘从A柱移动到B柱、将第4个圆盘从A柱移动到C柱、将前3个圆盘从B柱移动到C柱三个子问题;同理,子问题3也可以继续递归拆解。
对于子问题2,移动第6个圆盘可以直接从A柱移动到C柱。
综上所述,移动6个圆盘的汉诺塔问题可以表示为以下递归函数:
```
def hanoi(n, A, B, C):
if n == 1:
print("Move disk", n, "from", A, "to", C)
else:
hanoi(n-1, A, C, B)
print("Move disk", n, "from", A, "to", C)
hanoi(n-1, B, A, C)
```
其中n表示圆盘的数量,A、B、C分别表示三根柱子的名称。
汉诺塔问题的示例图汉诺塔是一个数学难题,其问题描述为如何将所有圆盘从A移动到C。请用Python编写一个汉诺塔的移动函数,采用递归方法解决这个难题,要求输入汉诺塔的层数,输出整个移动流程;并再设计一个绘图函数,用turtle实现上图效果,表述汉诺塔移动流程。
好的,这是一个Python的代码实现,包括汉诺塔移动函数和绘图函数:
```python
import turtle
# 汉诺塔移动函数
def hanoi(n, a, b, c):
if n == 1:
print(a, '->', c)
else:
hanoi(n-1, a, c, b)
print(a, '->', c)
hanoi(n-1, b, a, c)
# 绘图函数
def draw_hanoi(n, a, b, c, width, height):
def draw_tower(x, y, w, h):
t.penup()
t.goto(x, y)
t.pendown()
t.setheading(0)
t.forward(w/2)
t.right(90)
t.forward(h)
t.right(90)
t.forward(w)
t.right(90)
t.forward(h)
t.right(90)
t.forward(w/2)
def draw_disk(x, y, w, h):
t.penup()
t.goto(x, y)
t.pendown()
t.setheading(0)
t.forward(w/2)
t.right(90)
t.forward(h)
t.right(90)
t.forward(w)
t.right(90)
t.forward(h)
t.right(90)
t.forward(w/2)
def move_disk(src, dst):
disk = towers[src].pop()
x, y = positions[src][len(towers[src])]
t.penup()
t.goto(x, y)
t.pendown()
x, y = positions[dst][len(towers[dst])]
t.goto(x, y)
towers[dst].append(disk)
t = turtle.Turtle()
t.speed('fastest')
t.hideturtle()
t.penup()
tower_width = width // 3
tower_height = height // (n+2)
positions = {
a: [(tower_width*0.5, -tower_height*i) for i in range(n)],
b: [(tower_width*1.5, -tower_height*i) for i in range(n)],
c: [(tower_width*2.5, -tower_height*i) for i in range(n)],
}
draw_tower(tower_width*0.5, 0, tower_width, height)
draw_tower(tower_width*1.5, 0, tower_width, height)
draw_tower(tower_width*2.5, 0, tower_width, height)
towers = {
a: list(range(n, 0, -1)),
b: [],
c: [],
}
disks = [turtle.Turtle() for _ in range(n)]
for i in range(n):
w = tower_width * (n-i) / n
h = tower_height
draw_disk(*positions[a][i], w, h)
def move(n, src, dst, tmp):
if n == 1:
move_disk(src, dst)
else:
move(n-1, src, tmp, dst)
move_disk(src, dst)
move(n-1, tmp, dst, src)
move(n, a, c, b)
turtle.done()
# 测试
n = 3
hanoi(n, 'A', 'B', 'C')
draw_hanoi(n, 'A', 'B', 'C', 600, 400)
```
这个程序实现了汉诺塔移动函数 `hanoi()` 和绘图函数 `draw_hanoi()`。其中,`hanoi()` 函数使用递归实现汉诺塔的移动,将圆盘从 A 移动到 C。`draw_hanoi()` 函数使用 `turtle` 模块绘制汉诺塔的图形,并模拟移动过程。在 `draw_hanoi()` 函数中,使用 `positions` 变量记录每个圆盘的位置,使用 `towers` 变量记录每个塔的状态,使用 `disks` 变量记录每个圆盘的图形。在 `move()` 函数中,使用递归模拟移动过程,并在每次移动时更新 `towers` 和 `disks` 变量。最后调用 `turtle.done()` 函数显示图形。
阅读全文
相关推荐




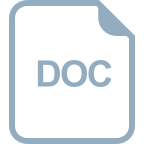
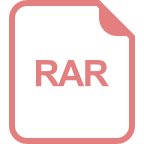

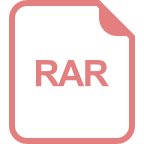








