java写一个斗地主游戏的游戏规则代码
时间: 2023-12-20 11:04:37 浏览: 25
斗地主是一种扑克牌游戏,以下是一个简单的斗地主游戏规则的 Java 代码实现:
1. 定义扑克牌类 Poker,包括花色和点数属性:
```java
public class Poker {
private String suit; // 花色
private int rank; // 点数
public Poker(String suit, int rank) {
this.suit = suit;
this.rank = rank;
}
public String getSuit() {
return suit;
}
public int getRank() {
return rank;
}
// 覆盖 toString() 方法,输出扑克牌的字符串表示
public String toString() {
String[] suits = {"♠", "♥", "♣", "♦"};
String[] ranks = {"2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K", "A"};
return suits[suit] + ranks[rank-2];
}
}
```
2. 定义牌堆类 Deck,包括扑克牌的初始化、洗牌和发牌方法:
```java
import java.util.ArrayList;
import java.util.Collections;
public class Deck {
private ArrayList<Poker> cards;
public Deck() {
cards = new ArrayList<Poker>();
for (int i = 0; i < 4; i++) { // 四种花色
for (int j = 2; j <= 14; j++) { // 13种点数
cards.add(new Poker(i, j));
}
}
}
public void shuffle() {
Collections.shuffle(cards);
}
public ArrayList<Poker> deal(int n) {
ArrayList<Poker> hand = new ArrayList<Poker>();
for (int i = 0; i < n; i++) {
hand.add(cards.remove(0));
}
return hand;
}
}
```
3. 定义游戏规则类 Rules,包括牌型判断和比较方法:
```java
import java.util.ArrayList;
public class Rules {
public static boolean isSingle(ArrayList<Poker> hand) {
return hand.size() == 1;
}
public static boolean isPair(ArrayList<Poker> hand) {
if (hand.size() != 2) {
return false;
}
Poker p1 = hand.get(0);
Poker p2 = hand.get(1);
return p1.getRank() == p2.getRank();
}
public static boolean isThree(ArrayList<Poker> hand) {
if (hand.size() != 3) {
return false;
}
Poker p1 = hand.get(0);
Poker p2 = hand.get(1);
Poker p3 = hand.get(2);
return p1.getRank() == p2.getRank() && p2.getRank() == p3.getRank();
}
public static boolean isBomb(ArrayList<Poker> hand) {
if (hand.size() != 4) {
return false;
}
Poker p1 = hand.get(0);
Poker p2 = hand.get(1);
Poker p3 = hand.get(2);
Poker p4 = hand.get(3);
return p1.getRank() == p2.getRank() && p2.getRank() == p3.getRank() && p3.getRank() == p4.getRank();
}
public static boolean isStraight(ArrayList<Poker> hand) {
if (hand.size() != 5) {
return false;
}
int rank = hand.get(0).getRank();
for (int i = 1; i < 5; i++) {
if (hand.get(i).getRank() != rank + i) {
return false;
}
}
return true;
}
public static boolean isFlush(ArrayList<Poker> hand) {
if (hand.size() != 5) {
return false;
}
String suit = hand.get(0).getSuit();
for (int i = 1; i < 5; i++) {
if (!hand.get(i).getSuit().equals(suit)) {
return false;
}
}
return true;
}
public static boolean isFullHouse(ArrayList<Poker> hand) {
if (hand.size() != 5) {
return false;
}
ArrayList<Poker> pair = new ArrayList<Poker>();
ArrayList<Poker> three = new ArrayList<Poker>();
for (Poker card : hand) {
if (pair.size() == 0 || card.getRank() == pair.get(0).getRank()) {
pair.add(card);
} else if (three.size() == 0 || card.getRank() == three.get(0).getRank()) {
three.add(card);
} else {
return false;
}
}
return pair.size() == 2 && three.size() == 3;
}
public static boolean isStraightFlush(ArrayList<Poker> hand) {
return isStraight(hand) && isFlush(hand);
}
public static boolean isRoyalFlush(ArrayList<Poker> hand) {
if (hand.size() != 5) {
return false;
}
int rank = hand.get(0).getRank();
for (int i = 1; i < 5; i++) {
if (hand.get(i).getRank() != rank + i) {
return false;
}
}
String suit = hand.get(0).getSuit();
for (int i = 1; i < 5; i++) {
if (!hand.get(i).getSuit().equals(suit)) {
return false;
}
}
return rank == 10;
}
public static boolean compare(ArrayList<Poker> hand1, ArrayList<Poker> hand2) {
if (isRoyalFlush(hand1) && !isRoyalFlush(hand2)) {
return true;
} else if (!isRoyalFlush(hand1) && isRoyalFlush(hand2)) {
return false;
} else if (isRoyalFlush(hand1) && isRoyalFlush(hand2)) {
return false;
} else if (isStraightFlush(hand1) && !isStraightFlush(hand2)) {
return true;
} else if (!isStraightFlush(hand1) && isStraightFlush(hand2)) {
return false;
} else if (isStraightFlush(hand1) && isStraightFlush(hand2)) {
return hand1.get(4).getRank() > hand2.get(4).getRank();
} else if (isFourOfAKind(hand1) && !isFourOfAKind(hand2)) {
return true;
} else if (!isFourOfAKind(hand1) && isFourOfAKind(hand2)) {
return false;
} else if (isFourOfAKind(hand1) && isFourOfAKind(hand2)) {
return hand1.get(2).getRank() > hand2.get(2).getRank();
} else if (isFullHouse(hand1) && !isFullHouse(hand2)) {
return true;
} else if (!isFullHouse(hand1) && isFullHouse(hand2)) {
return false;
} else if (isFullHouse(hand1) && isFullHouse(hand2)) {
return hand1.get(2).getRank() > hand2.get(2).getRank();
} else if (isFlush(hand1) && !isFlush(hand2)) {
return true;
} else if (!isFlush(hand1) && isFlush(hand2)) {
return false;
} else if (isFlush(hand1) && isFlush(hand2)) {
return hand1.get(4).getRank() > hand2.get(4).getRank();
} else if (isStraight(hand1) && !isStraight(hand2)) {
return true;
} else if (!isStraight(hand1) && isStraight(hand2)) {
return false;
} else if (isStraight(hand1) && isStraight(hand2)) {
return hand1.get(4).getRank() > hand2.get(4).getRank();
} else if (isThree(hand1) && !isThree(hand2)) {
return true;
} else if (!isThree(hand1) && isThree(hand2)) {
return false;
} else if (isThree(hand1) && isThree(hand2)) {
return hand1.get(2).getRank() > hand2.get(2).getRank();
} else if (isPair(hand1) && !isPair(hand2)) {
return true;
} else if (!isPair(hand1) && isPair(hand2)) {
return false;
} else if (isPair(hand1) && isPair(hand2)) {
return hand1.get(1).getRank() > hand2.get(1).getRank();
} else {
return hand1.get(4).getRank() > hand2.get(4).getRank();
}
}
}
```
4. 定义斗地主游戏类 Landlord,包括游戏流程和判断胜负:
```java
import java.util.ArrayList;
import java.util.Scanner;
public class Landlord {
private Deck deck;
private ArrayList<ArrayList<Poker>> hands;
private ArrayList<Poker> pool;
private int currentPlayer;
private int lastPlayer;
private ArrayList<Poker> lastHand;
public Landlord() {
deck = new Deck();
deck.shuffle();
hands = new ArrayList<ArrayList<Poker>>();
for (int i = 0; i < 3; i++) {
hands.add(deck.deal(17));
}
pool = deck.deal(3);
currentPlayer = 0;
lastPlayer = -1;
lastHand = null;
}
public void play() {
while (!gameOver()) {
showHands();
showPool();
if (currentPlayer == lastPlayer) {
lastHand = null;
}
ArrayList<Poker> hand = getHand();
if (hand == null) {
System.out.println("不符合规则,请重新出牌!");
continue;
}
if (lastHand != null && !Rules.compare(hand, lastHand)) {
System.out.println("不能出这个组合,请重新出牌!");
continue;
}
lastPlayer = currentPlayer;
lastHand = hand;
ArrayList<Poker> currentHand = hands.get(currentPlayer);
for (Poker card : hand) {
currentHand.remove(card);
}
pool.addAll(hand);
currentPlayer = (currentPlayer + 1) % 3;
}
showHands();
showPool();
int winner = getWinner();
System.out.println("游戏结束,玩家 " + winner + " 获胜!");
}
private boolean gameOver() {
for (ArrayList<Poker> hand : hands) {
if (hand.size() == 0) {
return true;
}
}
return false;
}
private void showHands() {
for (int i = 0; i < 3; i++) {
System.out.print("玩家 " + i + ":");
ArrayList<Poker> hand = hands.get(i);
for (Poker card : hand) {
System.out.print(card + " ");
}
System.out.println();
}
}
private void showPool() {
System.out.print("桌面牌池:");
for (Poker card : pool) {
System.out.print(card + " ");
}
System.out.println();
}
private ArrayList<Poker> getHand() {
System.out.print("玩家 " + currentPlayer + " 请出牌:");
Scanner scanner = new Scanner(System.in);
String input = scanner.nextLine();
String[] cards = input.split(" ");
ArrayList<Poker> hand = new ArrayList<Poker>();
for (String card : cards) {
boolean found = false;
for (Poker p : hands.get(currentPlayer)) {
if (card.equals(p.toString())) {
hand.add(p);
found = true;
break;
}
}
if (!found) {
return null;
}
}
return hand;
}
private int getWinner() {
int winner = -1;
for (int i = 0; i < 3; i++) {
if (hands.get(i).size() == 0) {
winner = i;
}
}
if (winner == -1) {
return -1;
}
int score = 0;
for (Poker card : pool) {
if (card.getRank() == 5 || card.getRank() == 10 || card.getRank() == 13) {
score += 1;
} else if (card.getRank() == 11) {
score += 2;
} else if (card.getRank() == 12) {
score += 3;
}
}
return (winner + score) % 3;
}
public static void main(String[] args) {
Landlord game = new Landlord();
game.play();
}
}
```
以上是一个简单的斗地主游戏规则的 Java 代码实现,仅供参考。
相关推荐
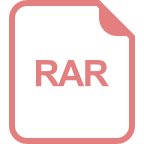
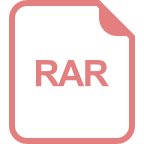










