#include<iostream> #include<stdio.h> using namespace std; const int MAX = 20000;//最大输入文本长度为10,000 unsigned char In[MAX + 1]; //输入文本的字节存储形式 unsigned int Out[MAX / 2 + 1]; //输出编码,以4个字节存储 int len=0; //文本数据的长度 int len_of_str = 0; //字节数组的长度 //计算文本数据的长度并保证其不大于10,000 int lenth(unsigned char* a) { int cnt = 0; while (*a != NULL) { cnt++; a++; if (cnt >= MAX) break; } return cnt/2; } //查找与传入字或标点符号相同的字(往后索引,避免重复) int find_same(unsigned char* a,unsigned char b,unsigned char c,int b_i,int len_a) { for (int i = b_i+2; i < len_a; i++) { if (b == a[i]&&c==a[i+1]) return i/2; } return -1; } //数组a为输入,b为编码后的数组 void code(unsigned char* a, unsigned int* b,int len_a,int len_b) { for (int i = 0; i < len_b; i ++) { b[i] = i; } for (int i = 0; i < len_a; i += 2) { int j = find_same(a, a[i], a[i + 1], i, len_a); if (j == -1||b[j] == b[i]) { continue; } b[j] = b[i/2]; } } int main() { int i = 0; //读取不定长度的文本 while(scanf_s("%c", &In[i], unsigned int(sizeof(In[i])))) { if (In[i] == '\n') break; i++; } len = lenth(In); len_of_str = len * 2 + 1; code(In, Out, len_of_str, len); for (int i = 0; i < len; i++) cout << Out[i] << " "; cout <<endl<< "传输数据的大小为:"<<len << endl; system("pause"); return 0; } 优化这段代码
时间: 2024-04-21 21:28:31 浏览: 28
1. 将使用的头文件放在代码文件的最开始,避免出现一些不必要的问题
2. 将常量MAX定义为20000,但是输入的文本数据的长度最大为10000,这里可以将MAX定义为10001,以便进行优化。
3. 由于In数组和Out数组的长度都是定值,可以直接用定义数组时的常量定义数组长度。
4. 可以将len_of_str的计算移到lenth函数中,避免重复计算。
5. 在函数中使用常量引用替代非常量引用,可以提高效率。
6. 在使用scanf_s时,第二个参数中不应该使用sizeof(In[i]),应该使用sizeof(In[0]),这里可以使用%u代替%c,提高效率。
7. 将数据类型无关的常量定义为const,可以提高代码的可读性。
8. 将代码块内的变量定义放在代码块的开头,避免出现一些不必要的问题。
综上所述,优化后的代码如下:
相关问题
#include<cstdio> #include<cstdlib> #include<cstring> #include<cmath> #include<ctime> #include<iostream> #include<algorithm> #include<map> #include<stack> #include<queue> #include<vector> #include<set> #include<string> #define dd double #define ll long long dd PI = acos(-1); using namespace std; const ll MAXN = 1e5 + 5; const ll INF = 1e9 + 5; ll n; struct node { ll x, y; }s[5000]; ll dp[MAXN] = { 0 }; int main() { //ios::sync_with_stdio(false); ll n, m; cin >> n >> m; for (ll i = 1; i <= n; i++) { cin >> s[i].x >> s[i].y; } for (ll i = 1; i <= n; i++) { for (ll j = m; j >= s[i].x; j--) { dp[j] = max(dp[j], dp[j - s[i].x] + s[i].y); } } cout << dp[m] << endl; }
这是一段动态规划求解背包问题的代码,用来求解一个容量为m的背包能够装下的最大价值。具体实现过程为:
1. 定义一个结构体node,用来存储物品的重量和价值。
2. 读入物品数量n和背包容量m,然后读入每个物品的重量和价值。
3. 使用二重循环,遍历每一个物品,然后从大到小枚举背包容量j,更新dp[j]的值,表示容量为j的背包能够装下的最大价值。
4. 最后输出dp[m]的值,即容量为m的背包能够装下的最大价值。
值得注意的是,这段代码中还定义了一些常量和宏定义,如MAXN、INF等,以及一些头文件和命名空间的使用。
使用c++编程详细补充函数接口,要求运行结果和输出样例相同,陈老师是一个比赛队的主教练。有一天,他想与团队成员开会,应该为这次会议安排教室。教室非常缺乏,所以教室管理员必须接受订单和拒绝订单以优化教室的利用率。如果接受一个订单,该订单的开始时间和结束时间成为一个活动。每个时间段只能安排一个订单(即假设只有一个教室)。请你找出一个最大化的总活动时间的方法。你的任务是这样的:读入订单,计算所有活动(接受的订单)占用时间的最大值。 函数接口定义: void solve(); 裁判测试程序样例: #include <stdio.h> #include <string.h> #include <vector> #include <algorithm> #include <iostream> using namespace std; #define MAX 101 struct NodeType { int b; //开始时间 int e; //结束时间 int length; //订单的执行时间 }; bool cmp(const NodeType &a,const NodeType &b) { //用于排序的运算符重载函数 return a.e<b.e; //按结束时间递增排序 } int n; //订单个数 NodeType A[MAX]; //存放订单 int dp[MAX]; //动态规划数组 int pre[MAX]; //pre[i]存放前驱订单编号 void solve(); int main() { cin>>n; for(int i=0;i<n;i++) cin>>A[i].b>>A[i].e; for (int i=0; i<n; i++) A[i].length=A[i].e-A[i].b; solve(); cout<<dp[n-1]; return 0; } /* 请在这里填写答案 */ 输入格式: 第一行是一个整数n,接着的n行中每一行包括两个整数b和e,其中b是一个订单开始时间,e是的结束时间。。 输出格式: 输出一行包括所有活动占用时间的最大值。 输入样例1: 11 1 4 3 5 0 6 5 7 3 8 5 9 6 10 8 11 8 12 2 13 12 15 输出样例1: 13
```c++
#include <iostream>
#include <algorithm>
using namespace std;
const int N = 110;
struct NodeType {
int b; // 开始时间
int e; // 结束时间
int length; // 订单的执行时间
} A[N];
int n;
int dp[N]; // 动态规划数组
int pre[N]; // pre[i]存放前驱订单编号
bool cmp(const NodeType &a, const NodeType &b) {
return a.e < b.e; // 按结束时间递增排序
}
void solve() {
sort(A, A + n, cmp); // 按结束时间递增排序
dp[0] = A[0].length;
for (int i = 1; i < n; i++) {
dp[i] = A[i].length;
pre[i] = -1; // 初始化为-1
for (int j = i - 1; j >= 0; j--) {
if (A[j].e <= A[i].b) { // j号订单不与i冲突
if (dp[j] + A[i].length > dp[i]) {
dp[i] = dp[j] + A[i].length;
pre[i] = j; // 更新前驱
}
}
}
}
}
void print_path(int i) {
if (i == -1) return; // 递归边界
print_path(pre[i]); // 先输出前驱
cout << i << " "; // 再输出自己的编号
}
int main() {
cin >> n;
for (int i = 0; i < n; i++) {
cin >> A[i].b >> A[i].e;
A[i].length = A[i].e - A[i].b;
}
solve();
cout << dp[n-1] << endl;
print_path(n-1); // 输出路径
return 0;
}
```
相关推荐
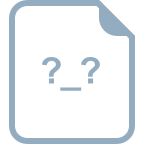












