Tree insert(Tree BST, int x){ if(!BST){ BST=(Tree)malloc(sizeof(struct TNode)); BST->Data=x; BST->Left=BST->Right=NULL; } if(x<BST->Data) BST->Right=insert(BST->Right,x); else if(x>BST->Data) BST->Left=insert(BST->Left,x); return BST; }
时间: 2024-04-28 20:26:21 浏览: 80
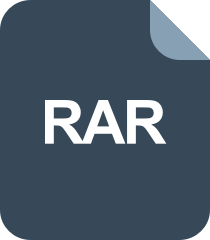
BST_Tree.rar_bst_tree
这是一段二叉搜索树(Binary Search Tree,BST)的插入操作代码。它的作用是将一个值为 x 的节点插入到 BST 中,并返回插入后的 BST 的根节点指针。
首先,如果 BST 为空,就创建一个新节点,并将 x 赋值给它的 Data 域,同时将其左右子树指针设为空。然后,根据 x 和当前节点的大小关系,选择递归插入左子树或右子树,直到找到一个空位置。最后,返回插入后的 BST 的根节点指针。
需要注意的是,这段代码中的 BST 参数是指向根节点的指针,因为在递归过程中,BST 的值可能会被改变,直到最终插入新节点后,根节点可能已经发生了变化。因此,必须返回插入后的根节点指针。
阅读全文
相关推荐
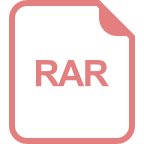
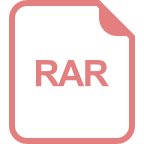

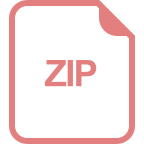
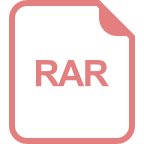









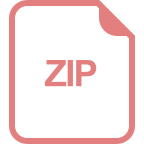
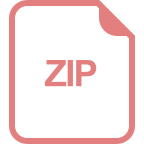
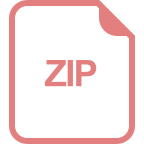