## Problem 7: Is BST Write a function `is_bst`, which takes a Tree `t` and returns `True` if, and only if, `t` is a valid binary search tree, which means that: - Each node has at most two children (a leaf is automatically a valid binary search tree). - The children are valid binary search trees. - For every node, the entries in that node's left child are less than or equal to the label of the node. - For every node, the entries in that node's right child are greater than the label of the node. An example of a BST is:  Note that, if a node has only one child, that child could be considered either the left or right child. You should take this into consideration. Hint: It may be helpful to write helper functions `bst_min` and `bst_max` that return the minimum and maximum, respectively, of a Tree if it is a valid binary search tree. ```python def is_bst(t): """Returns True if the Tree t has the structure of a valid BST. >>> t1 = Tree(6, [Tree(2, [Tree(1), Tree(4)]), Tree(7, [Tree(7), Tree(8)])]) >>> is_bst(t1) True >>> t2 = Tree(8, [Tree(2, [Tree(9), Tree(1)]), Tree(3, [Tree(6)]), Tree(5)]) >>> is_bst(t2) False >>> t3 = Tree(6, [Tree(2, [Tree(4), Tree(1)]), Tree(7, [Tree(7), Tree(8)])]) >>> is_bst(t3) False >>> t4 = Tree(1, [Tree(2, [Tree(3, [Tree(4)])])]) >>> is_bst(t4) True >>> t5 = Tree(1, [Tree(0, [Tree(-1, [Tree(-2)])])]) >>> is_bst(t5) True >>> t6 = Tree(1, [Tree(4, [Tree(2, [Tree(3)])])]) >>> is_bst(t6) True >>> t7 = Tree(2, [Tree(1, [Tree(5)]), Tree(4)]) >>> is_bst(t7) False """ "*** YOUR CODE HERE ***" ``` 。
时间: 2024-03-03 20:47:21 浏览: 104
根据你的问题,`is_bst` 函数存在问题,因为它只有一个参数。为了实现该函数,需要编写两个辅助函数 `bst_min` 和 `bst_max`,它们返回一个树的最小值和最大值。然后,可以使用这些帮助器函数来实现主函数 `is_bst`。以下是代码的修改版本:
```python
def bst_min(t):
"""Returns the minimum value in a binary search tree."""
if t.is_leaf():
return t.label
return min(t.label, bst_min(t.branches[0]), bst_min(t.branches[-1]))
def bst_max(t):
"""Returns the maximum value in a binary search tree."""
if t.is_leaf():
return t.label
return max(t.label, bst_max(t.branches[-1]), bst_max(t.branches[0]))
def is_bst(t):
"""Returns True if the Tree t has the structure of a valid BST.
>>> t1 = Tree(6, [Tree(2, [Tree(1), Tree(4)]), Tree(7, [Tree(7), Tree(8)])])
>>> is_bst(t1)
True
>>> t2 = Tree(8, [Tree(2, [Tree(9), Tree(1)]), Tree(3, [Tree(6)]), Tree(5)])
>>> is_bst(t2)
False
>>> t3 = Tree(6, [Tree(2, [Tree(4), Tree(1)]), Tree(7, [Tree(7), Tree(8)])])
>>> is_bst(t3)
False
>>> t4 = Tree(1, [Tree(2, [Tree(3, [Tree(4)])])])
>>> is_bst(t4)
True
>>> t5 = Tree(1, [Tree(0, [Tree(-1, [Tree(-2)])])])
>>> is_bst(t5)
True
>>> t6 = Tree(1, [Tree(4, [Tree(2, [Tree(3)])])])
>>> is_bst(t6)
True
>>> t7 = Tree(2, [Tree(1, [Tree(5)]), Tree(4)])
>>> is_bst(t7)
False
"""
if t.is_leaf():
return True
elif len(t.branches) == 1:
return is_bst(t.branches[0])
else:
left = t.branches[0]
right = t.branches[-1]
if bst_max(left) <= t.label < bst_min(right):
return is_bst(left) and is_bst(right)
else:
return False
```
阅读全文
相关推荐
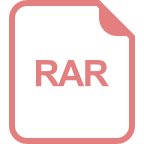
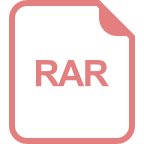











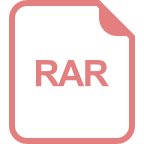
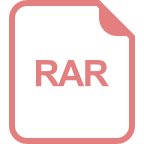
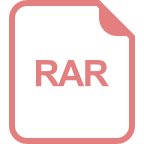
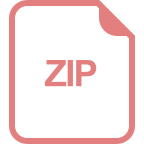
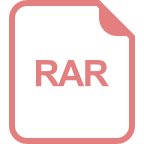