The Lowest Common Ancestors (LCA) of two nodes in a BST is the deepest node that has both nodes as its descendants (A node itself can be its descendant). For example, in the following BST: 10 / \ 8 13 / \ / \ 7 9 11 15 The LCA of 7 and 9 is 8, the LCA of 7 and 8 is 8, the LCA of 7 and 11 is 10. Your task is to implement a function LCA(bst, x, y) to find the lowest common ancestors of two nodes x and y in the binary search tree bst. If both x and y exists in the BST, return the LCA, otherwise return None. Note that, you will need to implement the BST with insert and search operations in the answer box. Hint: The LCA should have one of the two nodes in its left subtree and the other in its right subtree.
时间: 2023-12-03 14:02:25 浏览: 102
Here's an implementation of the BST with insert and search operations, as well as the LCA function:
```python
class Node:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
class BST:
def __init__(self):
self.root = None
def insert(self, val):
if not self.root:
self.root = Node(val)
else:
curr = self.root
while True:
if val < curr.val:
if not curr.left:
curr.left = Node(val)
break
else:
curr = curr.left
else:
if not curr.right:
curr.right = Node(val)
break
else:
curr = curr.right
def search(self, val):
curr = self.root
while curr:
if val == curr.val:
return True
elif val < curr.val:
curr = curr.left
else:
curr = curr.right
return False
def LCA(self, x, y):
if not self.root:
return None
curr = self.root
while curr:
if x < curr.val and y < curr.val:
curr = curr.left
elif x > curr.val and y > curr.val:
curr = curr.right
else:
return curr.val
return None
```
The insert and search operations are fairly standard for a binary search tree. The LCA function works by starting at the root node and traversing down the tree. If both x and y are less than the current node's value, we move to the left subtree. If both x and y are greater than the current node's value, we move to the right subtree. If x and y are on opposite sides of the current node's value, then the current node is the LCA. If we reach a leaf node without finding the LCA, then x and/or y are not in the tree and we return None.
阅读全文
相关推荐
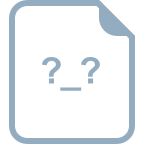
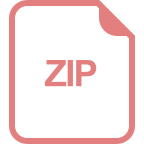
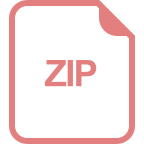
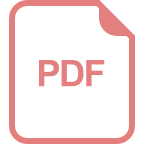
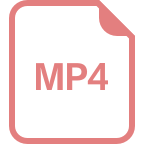
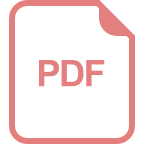
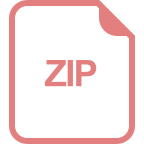
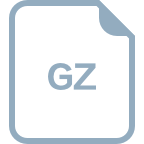











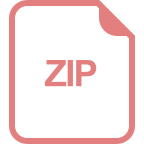