Write a procedure named ShowParams that displays the address and hexadecimal value of the 32-bit parameters on the runtime stack of the procedure that called it. The parameters are to be displayed in order from the lowest address to the highest. Input to the procedure will be a single integer that indicates the number of parameters to display. For example, suppose the following statement in main calls MySample, passing three arguments: INVOKE MySample, 1234h, 5000h, 6543h Next, inside MySample, we make a call to ShowParams, passing the number of parameters: MySample PROC first:DWORD, second:DWORD, third:DWORD paramCount = 3 call ShowParams, paramCount
时间: 2024-01-28 16:05:25 浏览: 44
Sure, here's a possible implementation of the ShowParams procedure in x86 assembly language:
```
ShowParams PROC paramCount:DWORD
push ebp
mov ebp, esp
sub esp, 8 ; reserve space for local variables
mov eax, [ebp + 8] ; get the number of parameters from the stack
mov ebx, ebp ; initialize ebx to the base pointer
add ebx, 12 ; skip the return address and the base pointer
; loop over the parameters and display their addresses and values
mov ecx, 0 ; initialize a loop counter
LoopParams:
cmp ecx, eax ; have we displayed all the parameters?
jge EndLoopParams
push ebx ; save the current parameter address on the stack
push dword ptr [ebx] ; push the parameter value on the stack
push offset ParamFormat ; push the format string on the stack
call printf ; call the C standard library printf function
add esp, 12 ; clean up the stack
add ebx, 4 ; move to the next parameter
inc ecx ; increment the loop counter
jmp LoopParams
EndLoopParams:
mov esp, ebp ; restore the stack pointer
pop ebp
ret
ParamFormat db "Param %d: address=%p, value=%08Xh", 0
ShowParams ENDP
```
The procedure first saves the base pointer and sets up a new stack frame. It then subtracts 8 bytes from the stack pointer to reserve space for local variables. The loop over the parameters starts by getting the number of parameters from the stack and initializing the ebx register to the address of the first parameter. The loop counter is initialized to 0, and we jump to the label LoopParams. Inside the loop, we push the current parameter address, value, and a format string on the stack, and call the C standard library function printf to display them. We then clean up the stack and move to the next parameter. The loop counter is incremented, and we jump back to LoopParams if we haven't displayed all the parameters yet. Once the loop is done, we restore the stack pointer, pop the base pointer, and return.
Note that this implementation assumes that the printf function is available and properly linked with the program. Also, the format string uses the %p and %08X format specifiers to display the parameter address and value in hexadecimal format, respectively. You may need to adjust the format string if your platform or compiler uses different conventions.
相关推荐
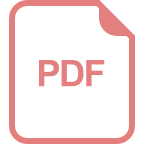
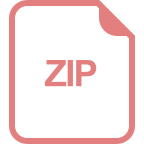
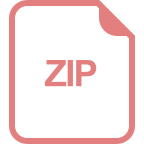















