Write a procedure named DumpMemory that encapsulates the DumpMem procedure in the Irvine32 library. Use declared parameters and the USES directive. The following is an example of how it should be called: INVOKE DumpMemory,OFFSET array,LENGTHOF array,TYPE array Write a test program that calls your procedure several times, using a variety of data types.
时间: 2024-02-04 15:03:46 浏览: 100
Sure, here's the procedure you requested:
```
DumpMemory PROC uses ebx esi edi,
pAddress:PTR BYTE, // pointer to the start of the memory block
dwBytes:DWORD, // size of the memory block in bytes
dwType:DWORD // type of the memory block (see the Irvine32 library for a list of types)
; This procedure encapsulates the DumpMem procedure in the Irvine32 library
; and displays the contents of the memory block starting at pAddress
; with the specified type and length.
; Save the registers that will be modified
push ebx
push esi
push edi
; Call the Irvine32 DumpMem procedure
lea ebx, pAddress
mov esi, dwBytes
mov edi, dwType
call DumpMem
; Restore the registers
pop edi
pop esi
pop ebx
ret
DumpMemory ENDP
```
And here's an example test program that calls the DumpMemory procedure with different data types:
```
include Irvine32.inc
.data
array1 BYTE 10h, 20h, 30h, 40h, 50h
array2 WORD 1000h, 2000h, 3000h, 4000h, 5000h
array3 DWORD 100000h, 200000h, 300000h, 400000h, 500000h
array4 QWORD 1000000000h, 2000000000h, 3000000000h, 4000000000h, 5000000000h
message1 BYTE "Dumping array1:", 0
message2 BYTE "Dumping array2:", 0
message3 BYTE "Dumping array3:", 0
message4 BYTE "Dumping array4:", 0
.code
main PROC
; Dump array1
lea ebx, message1
call WriteString
INVOKE DumpMemory,OFFSET array1,LENGTHOF array1,TYPE BYTE
; Dump array2
lea ebx, message2
call WriteString
INVOKE DumpMemory,OFFSET array2,LENGTHOF array2,TYPE WORD
; Dump array3
lea ebx, message3
call WriteString
INVOKE DumpMemory,OFFSET array3,LENGTHOF array3,TYPE DWORD
; Dump array4
lea ebx, message4
call WriteString
INVOKE DumpMemory,OFFSET array4,LENGTHOF array4,TYPE QWORD
exit
main ENDP
```
This program creates four different arrays of different data types, and then calls the DumpMemory procedure to display their contents. The output should show the memory contents of each array in hexadecimal format.
阅读全文
相关推荐
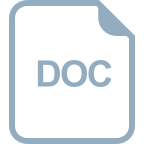
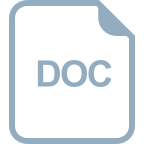
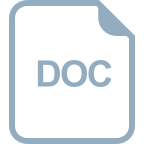

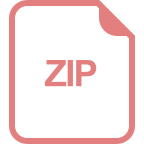
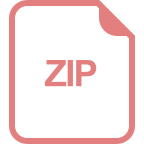
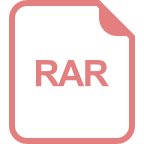
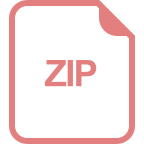
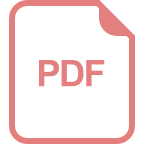
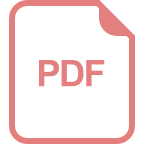
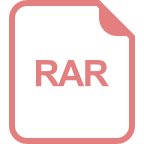
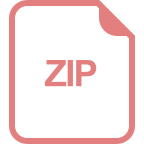
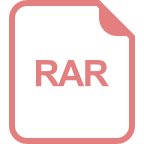
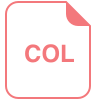
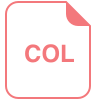



