有一段PCM单声道数据,采样是44100,16位,使用C语言写第一个分析分贝大小的程序,分贝最大为100,最小为0,
时间: 2024-10-11 07:17:54 浏览: 41
在C语言中,你可以使用标准库函数来进行这样的音频处理,首先需要将16位的PCM数据转换成浮点数,然后计算其幅度并将其转换为分贝值。这里是一个简单的示例:
```c
#include <stdio.h>
#include <math.h>
// 计算样本的幅度并转为dB
double sample_to_dB(short sample) {
float magnitude = ((float)sample) / (float)(1 << 15); // 转换为0到1范围内的浮点数
return 20 * log10(magnitude); // 对数计算分贝值
}
int main() {
const int sample_rate = 44100;
short pcm_data[/*假设数据长度*/]; // 单声道数据
int data_length = sizeof(pcm_data) / sizeof(short);
for (int i = 0; i < data_length; ++i) {
double db_value = sample_to_dB(pcm_data[i]);
if (db_value > 100) { // 如果超过最大分贝
printf("Sample %d is at dB level beyond the limit (100). Adjust accordingly.\n", i);
db_value = 100;
} else if (db_value < 0) { // 如果低于最小分贝
printf("Sample %d is at dB level below the limit (0). Set to 0.\n", i);
db_value = 0;
}
// 打印当前样本的dB值
printf("Sample %d: %.2f dB\n", i, db_value);
}
return 0;
}
```
这个程序会遍历PCM数据,对每个样本进行分贝计算,并根据给定的最大和最小分贝限制调整结果。请注意,实际操作时,你需要提供实际的PCM数据数组,并处理可能的数据读取错误。
阅读全文
相关推荐
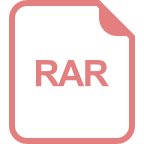
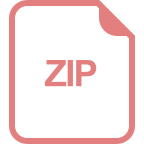

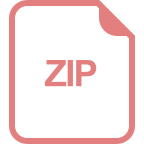
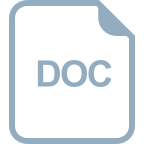










