android studio广播示例
时间: 2024-12-07 15:13:26 浏览: 28
在Android开发中,广播(Broadcast)是一种用于在不同组件之间传递消息的机制。广播可以用于在应用程序内部或与其他应用程序之间传递消息。以下是一个简单的Android Studio广播示例,展示如何发送和接收广播。
### 1. 创建广播接收器
首先,我们需要创建一个继承自`BroadcastReceiver`的类,并重写`onReceive`方法来处理接收到的广播。
```java
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.widget.Toast;
public class MyBroadcastReceiver extends BroadcastReceiver {
@Override
public void onReceive(Context context, Intent intent) {
// 处理接收到的广播
String action = intent.getAction();
if ("com.example.MY_ACTION".equals(action)) {
Toast.makeText(context, "Received broadcast", Toast.LENGTH_SHORT).show();
}
}
}
```
### 2. 注册广播接收器
在`AndroidManifest.xml`中注册广播接收器,或者在代码中动态注册。
#### 在`AndroidManifest.xml`中注册
```xml
<receiver android:name=".MyBroadcastReceiver">
<intent-filter>
<action android:name="com.example.MY_ACTION"/>
</intent-filter>
</receiver>
```
#### 在代码中动态注册
```java
import android.content.IntentFilter;
// 在Activity或其他组件中
MyBroadcastReceiver receiver = new MyBroadcastReceiver();
IntentFilter filter = new IntentFilter("com.example.MY_ACTION");
registerReceiver(receiver, filter);
```
### 3. 发送广播
使用`Intent`来发送广播。
```java
Intent intent = new Intent("com.example.MY_ACTION");
sendBroadcast(intent);
```
### 4. 动态注册和注销接收器
在不需要接收广播时,记得注销接收器以避免内存泄漏。
```java
@Override
protected void onDestroy() {
super.onDestroy();
unregisterReceiver(receiver);
}
```
### 完整示例
#### `MainActivity.java`
```java
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.content.IntentFilter;
import android.os.Bundle;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
private MyBroadcastReceiver receiver;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
receiver = new MyBroadcastReceiver();
IntentFilter filter = new IntentFilter("com.example.MY_ACTION");
registerReceiver(receiver, filter);
// 发送广播
sendBroadcast(new Intent("com.example.MY_ACTION"));
}
@Override
protected void onDestroy() {
super.onDestroy();
unregisterReceiver(receiver);
}
}
```
#### `MyBroadcastReceiver.java`
```java
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.widget.Toast;
public class MyBroadcastReceiver extends BroadcastReceiver {
@Override
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
if ("com.example.MY_ACTION".equals(action)) {
Toast.makeText(context, "Received broadcast", Toast.LENGTH_SHORT).show();
}
}
}
```
###
阅读全文
相关推荐
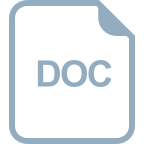
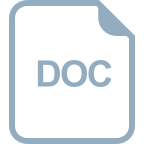
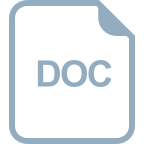
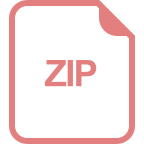
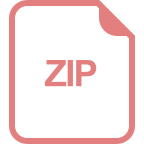
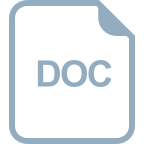












