c++bool和if else
时间: 2024-02-17 12:57:18 浏览: 23
以下是关于C++中bool和if else的介绍:
1. bool类型是C++中的一种基本数据类型,它只有两个取值:true和false。在C++中,true和false分别被定义为1和0。
2. if else是C++中的一种条件语句,用于根据条件执行不同的代码块。if else语句的基本语法如下:
```c++
if (condition) {
// 如果条件成立,执行这里的代码块
} else {
// 如果条件不成立,执行这里的代码块
}
```
其中,condition是一个表达式,如果它的值为true,则执行if语句后面的代码块;否则,执行else语句后面的代码块。
例如,下面的代码演示了如何使用if else语句判断一个数是否为偶数:
```c++
int num = 6;
if (num % 2 == 0) {
std::cout << num << " is even." << std::endl;
} else {
std::cout << num << " is odd." << std::endl; }
```
输出结果为:6 is even.
3. 在if else语句中,条件表达式的值可以是任何类型,不一定是bool类型。如果条件表达式的值不是bool类型,则会自动转换为bool类型。如果条件表达式的值为0,则被视为false;则,被视为true。
例如,下面的代码演示了如何使用if else语句判断一个数是否为正数:
```c++
int num = -3;
if (num > 0) {
std::cout << num << " is positive." << std::endl;
} else {
std::cout << num << " is not positive." << std::endl;
}
```
输出结果为:-3 is not positive.
4. 在if else语句中,可以使用嵌套的if else语句来实现多重条件判断。例如,下面的代码演示了如何使用嵌套的if else语句判断一个数的正负性:
```c++
int num = -3;
if (num > 0) {
std::cout << num << " is positive." << std::endl;
} else if (num < 0) {
std::cout << num << " is negative." << std::endl;
} else {
std::cout << num << " is zero." << std::endl;
}
```
输出结果为:-3 is negative.
相关推荐
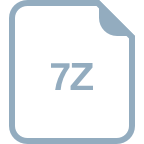
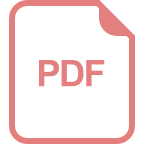
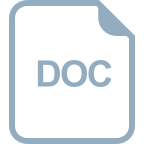














